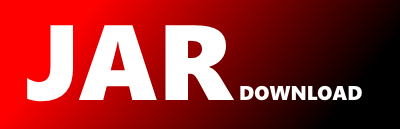
io.hypertrack.factory.HyperTrackFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hypertrack-java Show documentation
Show all versions of hypertrack-java Show documentation
A Java wrapper for the HyperTrack API https://hypertrack.io
The newest version!
package io.hypertrack.factory;
import com.google.gson.Gson;
import com.google.gson.internal.LinkedTreeMap;
import io.hypertrack.model.HyperTrackArrayList;
import io.hypertrack.model.HyperTrackModel;
import io.hypertrack.net.HyperTrackClient;
import io.hypertrack.net.HyperTrackException;
import org.apache.http.NameValuePair;
import org.apache.http.client.utils.URLEncodedUtils;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Abstract factory class for HyperTrack models.
* Factories handle all communication between models and client.
* Defines methods for create, retrieve, list models.
*
* @param Class of model
*/
public abstract class HyperTrackFactory {
/** HyperTrack client object to send requests. */
private HyperTrackClient client;
/**
* Instantiate factory class object by injecting client.
*
* @param client HyperTrack client object
*/
protected HyperTrackFactory(final HyperTrackClient client) {
this.client = client;
}
/**
* Method to instantiate build HyperTrack model objects.
*
* @param params Properties of model object
* @return HyperTrack model object
*/
protected abstract T makeNew(Map params);
/**
* Return url endpoint for the model.
*
* @return API endpoint for model
*/
protected abstract String getModelUrl();
/**
* Return url of model instance.
*
* @param instanceId ID of model instance
* @return API endpoint for instance
*/
public String getInstanceUrl(String instanceId) {
return getModelUrl() + instanceId + "/";
}
/**
* Retrieve model instance from client by instance id.
*
* @param id ID of model instance
* @return Model object with instance parameters
*/
public T retrieve(String id) {
try {
return getAndCreateFromId(id);
} catch (HyperTrackException e) {
e.printStackTrace();
throw e;
}
}
/**
* Create model instance with model parameters.
*
* @param params Map of instance parameters
* @return Model instance with id and other parameters
*/
public T create(Map params) {
try {
return postAndCreateFromParams(params);
} catch (HyperTrackException e) {
e.printStackTrace();
throw e;
}
}
/**
* Retrieve list of model instances with optional filters.
*
* @param filters Filtering parameters for retrieval
* @return Custom array list of model instances
*/
public HyperTrackArrayList list(Map filters) {
try {
return getListAndCreate(filters, null);
} catch (HyperTrackException e) {
e.printStackTrace();
throw e;
}
}
/**
* Retrieve next page of instances list.
*
* @param list Paginated list object
* @return Next page as a list
*/
public HyperTrackArrayList getNextPage(HyperTrackArrayList list) {
Map filters = (Map)list.getNextFilters();
return getPageFromFilters(filters);
}
/**
* Retrieve previous page of instances list.
*
* @param list Paginated list object
* @return Previous page as a list
*/
public HyperTrackArrayList getPreviousPage(HyperTrackArrayList list) {
Map filters = (Map)list.getPreviousFilters();
return getPageFromFilters(filters);
}
/**
* Retrieve page of a list request.
*
* @param filters GET params to identify page
* @return Page with instances as list
*/
protected HyperTrackArrayList getPageFromFilters(Map filters) {
if (filters == null) {
return null;
}
return list(filters);
}
/**
* Patches model instance and updates with new values.
* For photo upload on User, use driverFactory.patchWithPhoto()
*
* @param model Model instance to be patched
* @param params New params to be patched in instance
*/
public void patch(T model, Map params) {
try {
String instanceId = model.getId();
Map newParams = getPatchedParams(instanceId, params);
updateFromParams(model, newParams);
} catch (HyperTrackException e) {
e.printStackTrace();
throw e;
}
}
/**
* Delete model instance from server.
*
* @param model Instance that is to be deleted
*/
public void delete(T model) {
try {
String instanceId = model.getId();
deleteById(instanceId);
model.setProperties(null);
} catch (HyperTrackException e) {
e.printStackTrace();
throw e;
}
}
/**
* Return model instance after retrieving it from client.
*
* @param id Id of instance to be retrieved
* @return Model instance
* @throws HyperTrackException
*/
protected T getAndCreateFromId(String id) throws HyperTrackException {
String response = client.request(getInstanceUrl(id), "GET", null);
return createFromParams(getMapFromResponse(response));
}
/**
* Return model instance after creating in at server.
*
* @param params Properties of object to be created
* @return Model instance
* @throws HyperTrackException
*/
protected T postAndCreateFromParams(Map params) throws HyperTrackException {
String response = client.request(getModelUrl(), "POST", params);
return createFromParams(getMapFromResponse(response));
}
/**
* Return list of model instances fetched from the server.
*
* @param filters Map to filter the list
* @param customUrl If fetching list from custom endpoint, else model url is taken
* @return List of fetched instances with pagination variables
* @throws HyperTrackException
*/
protected HyperTrackArrayList getListAndCreate(Map filters, String customUrl) throws HyperTrackException {
String url;
if (customUrl != null) {
url = customUrl;
} else {
url = getModelUrl();
}
String response = client.request(url, "GET", filters);
Map responseMap = getMapFromResponse(response);
ArrayList results = (ArrayList)responseMap.get("results");
ArrayList objectList = new ArrayList<>();
for (LinkedTreeMap temp : results) {
Map params = new HashMap<>(temp);
objectList.add(createFromParams(params));
}
Double countDouble = (Double)responseMap.get("count");
Integer count = countDouble.intValue();
String nextUrl = (String)responseMap.get("next");
String previousUrl = (String)responseMap.get("previous");
Map nextParams = getParamsFromUrl(nextUrl);
Map previousParams = getParamsFromUrl(previousUrl);
return new HyperTrackArrayList<>(count, previousParams, nextParams, objectList);
}
/**
* Delete model instance by id.
*
* @param instanceId Id of instance to be deleted
*/
protected void deleteById(String instanceId) throws HyperTrackException {
client.request(getInstanceUrl(instanceId), "DELETE", null);
}
/**
* Patch instance on the server and get new properties
*
* @param instanceId Id of instance to be patched.
* @param params Properties to be patched.
* @return
* @throws HyperTrackException
*/
protected Map getPatchedParams(String instanceId, Map params) throws HyperTrackException {
String response = client.request(getInstanceUrl(instanceId), "PATCH", params);
return getMapFromResponse(response);
}
/**
* Create new model instance from properties
*
* @param params Properties of new model instance
* @return Model instance
*/
private T createFromParams(Map params) {
return makeNew(params);
}
/**
* Convert response JSON string to POJO params map
*
* @param response Response string received from server
* @return Map of properties
*/
protected static Map getMapFromResponse(String response) {
Gson gson = new Gson();
Map map = new HashMap();
map = (Map)gson.fromJson(response, map.getClass());
return map;
}
/**
* Set properties of model instance.
*
* @param model Model instance to be updated
* @param params New properties for model instance
*/
protected void updateFromParams(T model, Map params) {
model.setProperties(params);
}
/**
* Return client object
*
* @return HyperTrack client object
*/
public HyperTrackClient getClient() {
return client;
}
/**
* Set client object as factory property
*
* @param client Instance of HyperTrack client
*/
public void setClient(HyperTrackClient client) {
this.client = client;
}
/**
* Reads GET params from a url as POJO map
*
* @param url Url to be decoded
* @return Map of GET parameters
*/
private Map getParamsFromUrl(String url) {
Map params = new HashMap<>();
if (url == null) {
return null;
}
try {
List rawParams = URLEncodedUtils.parse(new URI(url), "UTF-8");
for (NameValuePair param : rawParams) {
params.put(param.getName(), param.getValue());
}
return params;
} catch (final URISyntaxException e) {
throw new IllegalStateException("Invalid uri", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy