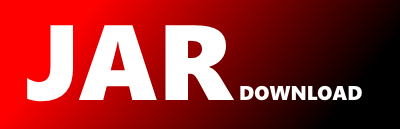
io.hypertrack.factory.UserFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hypertrack-java Show documentation
Show all versions of hypertrack-java Show documentation
A Java wrapper for the HyperTrack API https://hypertrack.io
The newest version!
package io.hypertrack.factory;
import io.hypertrack.model.User;
import io.hypertrack.net.HyperTrackClient;
import io.hypertrack.net.HyperTrackException;
import java.io.File;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Factory class for User model.
*/
public class UserFactory extends HyperTrackFactory {
private static String modelUrl = "users/";
public UserFactory(HyperTrackClient client) {
super(client);
}
@Override
protected User makeNew(Map params) {
return new User(params);
}
@Override
protected String getModelUrl() {
return modelUrl;
}
/**
* Patch user instance with a photo.
*
* @param user User instance to be patched
* @param photo Photo file
*/
public void patchWithPhoto(User user, File photo) {
String userId = user.getId();
HyperTrackClient client = getClient();
try {
Map fileParam = new HashMap<>();
fileParam.put("photo", photo);
String response = client.request(getInstanceUrl(userId), fileParam);
System.out.println("User photo updated: " + response);
updateFromParams(user, getMapFromResponse(response));
} catch (HyperTrackException e) {
e.printStackTrace();
throw e;
}
}
/**
* Assign actions to a user
*
* @param user User instance to be assigned actions
* @param actionIds List of action ids
*/
public void assignActions(User user, List actionIds) {
String userId = user.getId();
HyperTrackClient client = getClient();
try {
Map params = new HashMap<>();
params.put("action_ids", actionIds);
String response = client.request(getInstanceUrl(userId) + "assign_actions/", "POST", params);
System.out.println("Action Has been assigned to user: " + response);
updateFromParams(user, getMapFromResponse(response));
} catch (HyperTrackException e) {
e.printStackTrace();
throw new HyperTrackException("Could not assign actions", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy