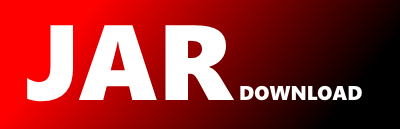
io.imunity.furms.ui.charts.ChartData Maven / Gradle / Ivy
/*
* Copyright (c) 2020 Bixbit s.c. All rights reserved.
* See LICENSE file for licensing information.
*/
package io.imunity.furms.ui.charts;
import io.imunity.furms.ui.charts.service.UserResourceUsage;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.Objects;
public class ChartData {
public final String unit;
public final String projectAllocationName;
public final LocalDate endTime;
public final List yResourceUsageLineValues;
public final List yChunkLineValues;
public final List yThresholdLineValues;
public final List yUsersUsageLinesValues;
public final List xArguments;
private ChartData(String unit, String projectAllocationName, LocalDate endTime,
List yResourceUsageLineValues, List yChunkLineValues, List yThresholdLineValues, List xArguments,
List yUsersUsageLinesValues) {
this.unit = unit;
this.projectAllocationName = projectAllocationName;
this.endTime = endTime;
this.yResourceUsageLineValues = Collections.unmodifiableList(yResourceUsageLineValues);
this.yChunkLineValues = Collections.unmodifiableList(yChunkLineValues);
this.yThresholdLineValues = Collections.unmodifiableList(yThresholdLineValues);
this.yUsersUsageLinesValues = Collections.unmodifiableList(yUsersUsageLinesValues);
this.xArguments = xArguments;
}
double getThresholdValue() {
Iterator iterator = yThresholdLineValues.iterator();
if(iterator.hasNext())
return iterator.next();
return 0;
}
List getAllXArguments() {
List times = new ArrayList<>(this.xArguments);
times.add(endTime);
return times;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ChartData chartData = (ChartData) o;
return Objects.equals(unit, chartData.unit) &&
Objects.equals(projectAllocationName, chartData.projectAllocationName) &&
Objects.equals(endTime, chartData.endTime) &&
Objects.equals(yResourceUsageLineValues, chartData.yResourceUsageLineValues) &&
Objects.equals(yChunkLineValues, chartData.yChunkLineValues) &&
Objects.equals(yThresholdLineValues, chartData.yThresholdLineValues) &&
Objects.equals(yUsersUsageLinesValues, chartData.yUsersUsageLinesValues) &&
Objects.equals(xArguments, chartData.xArguments);
}
@Override
public int hashCode() {
return Objects.hash(unit, projectAllocationName, endTime, yResourceUsageLineValues, yChunkLineValues, yThresholdLineValues, xArguments, yUsersUsageLinesValues);
}
@Override
public String toString() {
return "ChartData{" +
", unit='" + unit + '\'' +
", projectAllocationName='" + projectAllocationName + '\'' +
", endTime=" + endTime +
", yResourceUsageLineValues=" + yResourceUsageLineValues +
", yChunkLineValues=" + yChunkLineValues +
", yThresholdLineValues=" + yThresholdLineValues +
", yUsersUsageLinesValues=" + yUsersUsageLinesValues +
", xArguments=" + xArguments +
'}';
}
public static ChartDataBuilder builder() {
return new ChartDataBuilder();
}
public static final class ChartDataBuilder {
private String unit;
private String projectAllocationName;
private LocalDate endTime;
private List yResourceUsageLineValues = List.of();
private List yChunkLineValues = List.of();
private List yThresholdLineValues = List.of();
private List yUsersUsagesValues = List.of();
private List xArguments = List.of();
private ChartDataBuilder() {
}
public ChartDataBuilder unit(String unit) {
this.unit = unit;
return this;
}
public ChartDataBuilder projectAllocationName(String projectAllocationName) {
this.projectAllocationName = projectAllocationName;
return this;
}
public ChartDataBuilder endTime(LocalDate endTime) {
this.endTime = endTime;
return this;
}
public ChartDataBuilder yResourceUsageLineValues(List resourceUsages) {
this.yResourceUsageLineValues = resourceUsages;
return this;
}
public ChartDataBuilder yChunkLineValues(List chunks) {
this.yChunkLineValues = chunks;
return this;
}
public ChartDataBuilder yThresholdLineValues(List thresholds) {
this.yThresholdLineValues = thresholds;
return this;
}
public ChartDataBuilder xArguments(List times) {
this.xArguments = times;
return this;
}
public ChartDataBuilder yUsersUsagesValues(List usersUsages) {
this.yUsersUsagesValues = usersUsages;
return this;
}
public ChartData build() {
return new ChartData(unit, projectAllocationName, endTime, yResourceUsageLineValues, yChunkLineValues, yThresholdLineValues, xArguments, yUsersUsagesValues);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy