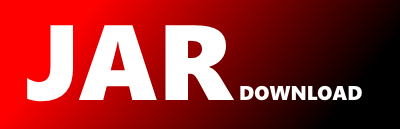
io.imunity.furms.ui.charts.service.CommunityAllocUsageSeriesGenerator Maven / Gradle / Ivy
/*
* Copyright (c) 2020 Bixbit s.c. All rights reserved.
* See LICENSE file for licensing information.
*/
package io.imunity.furms.ui.charts.service;
import io.imunity.furms.domain.project_allocation.ProjectAllocationId;
import io.imunity.furms.domain.resource_usage.ResourceUsage;
import org.springframework.stereotype.Component;
import java.time.LocalDate;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.BinaryOperator;
import static java.util.function.Function.identity;
import static java.util.stream.Collectors.collectingAndThen;
import static java.util.stream.Collectors.groupingBy;
import static java.util.stream.Collectors.toList;
import static java.util.stream.Collectors.toMap;
@Component
class CommunityAllocUsageSeriesGenerator {
List prepareYValuesForCommunityAllocationUsageLine(List xArguments,
Set allResourceUsageHistory) {
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy