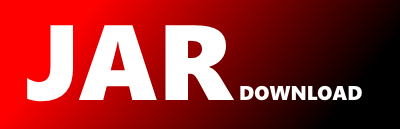
io.imunity.furms.ui.project_allocation.ProjectAllocationDataSnapshot Maven / Gradle / Ivy
/*
* Copyright (c) 2020 Bixbit s.c. All rights reserved.
* See LICENSE file for licensing information.
*/
package io.imunity.furms.ui.project_allocation;
import io.imunity.furms.domain.alarms.AlarmWithUserEmails;
import io.imunity.furms.domain.project_allocation.ProjectAllocationId;
import io.imunity.furms.domain.project_allocation_installation.ProjectAllocationChunk;
import io.imunity.furms.domain.project_allocation_installation.ProjectAllocationInstallation;
import io.imunity.furms.domain.project_allocation_installation.ProjectDeallocation;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import static java.util.function.Function.identity;
import static java.util.stream.Collectors.groupingBy;
import static java.util.stream.Collectors.toMap;
import static java.util.stream.Collectors.toSet;
public class ProjectAllocationDataSnapshot {
private final Map allocationByProjectAllocation;
private final Map deallocationsByProjectAllocationId;
private final Map> chunksByProjectAllocationId;
private final Map alarmsThresholdByProjectAllocationId;
public ProjectAllocationDataSnapshot(Set installations, Set uninstallations,
Set chunks, Set alarms) {
this.allocationByProjectAllocation = installations.stream()
.collect(toMap(installation -> installation.projectAllocationId, identity()));
this.deallocationsByProjectAllocationId = uninstallations.stream()
.collect(toMap(uninstallation -> uninstallation.projectAllocationId, identity()));
this.chunksByProjectAllocationId = chunks.stream()
.collect(groupingBy(chunk -> chunk.projectAllocationId, toSet()));
this.alarmsThresholdByProjectAllocationId = alarms.stream()
.collect(toMap(alarm -> alarm.projectAllocationId, alarm -> alarm.threshold));
}
public ProjectAllocationDataSnapshot(Set installations, Set uninstallations,
Set chunks) {
this(installations, uninstallations, chunks, Set.of());
}
public Optional getAllocation(ProjectAllocationId projectAllocationId) {
return Optional.ofNullable(allocationByProjectAllocation.get(projectAllocationId));
}
public Set getChunks(ProjectAllocationId projectAllocationId) {
return chunksByProjectAllocationId.getOrDefault(projectAllocationId, Set.of());
}
public Optional getDeallocationStatus(ProjectAllocationId projectAllocationId) {
return Optional.ofNullable(deallocationsByProjectAllocationId.get(projectAllocationId));
}
public int getAlarmThreshold(ProjectAllocationId projectAllocationId) {
return alarmsThresholdByProjectAllocationId.getOrDefault(projectAllocationId, 0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy