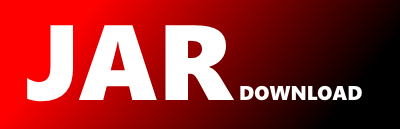
io.imunity.console.attribute.AttributeFieldWithEdit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unity-server-console Show documentation
Show all versions of unity-server-console Show documentation
Task oriented web based administration UI
/*
* Copyright (c) 2021 Bixbit - Krzysztof Benedyczak. All rights reserved.
* See LICENCE.txt file for licensing information.
*/
package io.imunity.console.attribute;
import static io.imunity.vaadin.elements.CSSVars.BASE_MARGIN;
import static io.imunity.vaadin.elements.CSSVars.TEXT_FIELD_MEDIUM;
import static io.imunity.vaadin.elements.CssClassNames.FIELD_ICON_GAP;
import static io.imunity.vaadin.elements.CssClassNames.POINTER;
import java.util.Collection;
import com.vaadin.flow.component.customfield.CustomField;
import com.vaadin.flow.component.icon.Icon;
import com.vaadin.flow.component.icon.VaadinIcon;
import com.vaadin.flow.component.orderedlayout.HorizontalLayout;
import com.vaadin.flow.component.textfield.TextField;
import io.imunity.vaadin.endpoint.common.exceptions.FormValidationException;
import io.imunity.vaadin.endpoint.common.plugins.attributes.AttributeHandlerRegistry;
import pl.edu.icm.unity.base.attribute.Attribute;
import pl.edu.icm.unity.base.attribute.AttributeType;
import pl.edu.icm.unity.base.message.MessageSource;
public class AttributeFieldWithEdit extends CustomField
{
private final MessageSource msg;
private final AttributeHandlerRegistry attrHandlerRegistry;
private final Collection attributeTypes;
protected String group;
private final TextField attributeTF;
private final boolean valuesRequired;
private Attribute attribute;
private AttributeType fixedAttributeType;
public AttributeFieldWithEdit(MessageSource msg, String caption,
AttributeHandlerRegistry attrHandlerRegistry,
Collection attributeTypes, String group, Attribute initial,
boolean valuesRequired)
{
this.msg = msg;
this.attrHandlerRegistry = attrHandlerRegistry;
this.group = group;
this.valuesRequired = valuesRequired;
this.attributeTypes = attributeTypes;
setLabel(caption);
this.attribute = initial;
attributeTF = new TextField();
attributeTF.setValue(msg.getMessage("AttributeField.noAttribute"));
attributeTF.setEnabled(false);
attributeTF.addValueChangeListener(this::fireEvent);
attributeTF.setWidth(TEXT_FIELD_MEDIUM.value());
Icon icon = VaadinIcon.EDIT.create();
icon.setTooltipText(msg.getMessage("AttributeField.edit"));
icon.addClassNames(POINTER.getName(), FIELD_ICON_GAP.getName());
icon.getStyle().set("margin-top", BASE_MARGIN.value());
icon.addClickListener(e -> editAttribute());
HorizontalLayout hl = new HorizontalLayout();
hl.setSpacing(false);
hl.add(attributeTF, icon);
add(hl);
}
private void editAttribute()
{
AttributeEditor theEditor = fixedAttributeType == null ?
new AttributeEditor(msg, attributeTypes, null, group, attrHandlerRegistry, valuesRequired) :
new AttributeEditor(msg, fixedAttributeType, null, group, attrHandlerRegistry);
if (attribute != null)
theEditor.setInitialAttribute(attribute);
AttributeEditDialog dialog = new AttributeEditDialog(msg,
msg.getMessage("AttributeField.edit"), newAttribute ->
{
setAttribute(newAttribute);
return true;
}, theEditor);
dialog.open();
}
private void checkAttributeSet() throws FormValidationException
{
if (attribute == null)
{
attributeTF.setErrorMessage(
msg.getMessage("AttributeField.attributeRequired"));
attributeTF.setInvalid(true);
throw new FormValidationException();
}
attributeTF.setInvalid(false);
attributeTF.setErrorMessage(null);
}
public Attribute getAttribute() throws FormValidationException
{
checkAttributeSet();
return attribute;
}
public void setAttribute(Attribute attribute)
{
this.attribute = attribute;
String attrRep = attrHandlerRegistry.getSimplifiedAttributeRepresentation(attribute);
attributeTF.setEnabled(true);
attributeTF.setValue(attrRep);
attributeTF.setEnabled(false);
attributeTF.setErrorMessage(null);
}
public void setFixedType(AttributeType attributeType)
{
this.fixedAttributeType = attributeType;
}
@Override
public Attribute getValue()
{
try
{
return getAttribute();
} catch (FormValidationException e)
{
return null;
}
}
@Override
protected Attribute generateModelValue()
{
return getValue();
}
@Override
protected void setPresentationValue(Attribute attribute)
{
setAttribute(attribute);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy