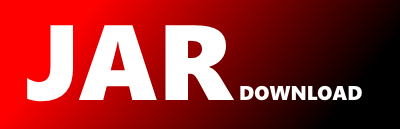
io.imunity.console.views.services.base.NewServiceViewBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unity-server-console Show documentation
Show all versions of unity-server-console Show documentation
Task oriented web based administration UI
/**
* Copyright (c) 2019 Bixbit - Krzysztof Benedyczak. All rights reserved.
* See LICENCE.txt file for licensing information.
*/
package io.imunity.console.views.services.base;
import com.vaadin.flow.component.UI;
import com.vaadin.flow.component.orderedlayout.Scroller;
import com.vaadin.flow.component.orderedlayout.VerticalLayout;
import com.vaadin.flow.router.BeforeEvent;
import com.vaadin.flow.router.OptionalParameter;
import io.imunity.console.components.InfoBanner;
import io.imunity.console.views.ConsoleViewComponent;
import io.imunity.console.views.EditViewActionLayoutFactory;
import io.imunity.vaadin.elements.BreadCrumbParameter;
import io.imunity.vaadin.elements.NotificationPresenter;
import io.imunity.vaadin.endpoint.common.api.SubViewSwitcher;
import io.imunity.vaadin.auth.services.ServiceDefinition;
import io.imunity.vaadin.auth.services.ServiceEditorComponent.ServiceEditorTab;
import io.imunity.vaadin.endpoint.common.sub_view_switcher.DefaultSubViewSwitcher;
import pl.edu.icm.unity.base.message.MessageSource;
import io.imunity.vaadin.endpoint.common.exceptions.FormValidationException;
import io.imunity.vaadin.endpoint.common.exceptions.ControllerException;
import java.util.Optional;
import static io.imunity.vaadin.elements.CssClassNames.AVOID_MAIN_LAYOUT_Y_SCROLLER;
/**
*
* @author P.Piernik
*
*/
public abstract class NewServiceViewBase extends ConsoleViewComponent
{
private final ServiceControllerBase controller;
private final NotificationPresenter notificationPresenter;
private final Class extends ConsoleViewComponent> mainServicesViewName;
private final MessageSource msg;
private BreadCrumbParameter breadCrumbParameter;
private MainServiceEditor editor;
private VerticalLayout mainView;
private VerticalLayout unsavedInfoBanner;
public NewServiceViewBase(MessageSource msg, ServiceControllerBase controller,
Class extends ConsoleViewComponent> mainServicesViewName, NotificationPresenter notificationPresenter)
{
this.msg = msg;
this.controller = controller;
this.mainServicesViewName = mainServicesViewName;
this.notificationPresenter = notificationPresenter;
}
@Override
public void setParameter(BeforeEvent event, @OptionalParameter String param)
{
breadCrumbParameter = new BreadCrumbParameter(null, msg.getMessage("new"));
getContent().removeAll();
mainView = new VerticalLayout();
unsavedInfoBanner = new InfoBanner(msg::getMessage);
try
{
editor = controller.getEditor(null, ServiceEditorTab.GENERAL, createSubViewSwitcher());
} catch (ControllerException e)
{
notificationPresenter.showError(e.getCaption(), e.getMessage());
UI.getCurrent().navigate(mainServicesViewName);
return;
}
mainView.setMargin(false);
mainView.add(editor);
mainView.add(EditViewActionLayoutFactory.createActionLayout(msg, false, mainServicesViewName, this::onConfirm));
getContent().add(unsavedInfoBanner);
Scroller scroller = new Scroller(mainView);
scroller.addClassName(AVOID_MAIN_LAYOUT_Y_SCROLLER.getName());
getContent().add(scroller);
}
private void onConfirm()
{
ServiceDefinition service;
try
{
service = editor.getService();
} catch (FormValidationException e)
{
String description = e.getMessage() == null ? msg.getMessage("Generic.formErrorHint") : e.getMessage();
notificationPresenter.showError(msg.getMessage("NewServiceView.invalidConfiguration"), description);
return;
}
try
{
controller.deploy(service);
} catch (ControllerException e)
{
notificationPresenter.showError(e.getCaption(), e.getMessage());
return;
}
UI.getCurrent().navigate(mainServicesViewName);
}
@Override
public Optional getDynamicParameter()
{
return Optional.ofNullable(breadCrumbParameter);
}
private SubViewSwitcher createSubViewSwitcher()
{
return new DefaultSubViewSwitcher(this, mainView, unsavedInfoBanner, breadCrumbParameter, this::setBreadCrumbParameter);
}
private void setBreadCrumbParameter(BreadCrumbParameter parameter)
{
breadCrumbParameter = parameter;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy