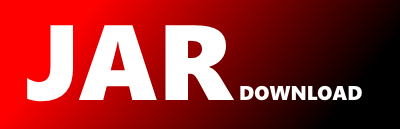
io.imunity.console.components.LocalizedRichTextEditorDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unity-server-console Show documentation
Show all versions of unity-server-console Show documentation
Task oriented web based administration UI
/*
* Copyright (c) 2021 Bixbit - Krzysztof Benedyczak. All rights reserved.
* See LICENCE.txt file for licensing information.
*/
package io.imunity.console.components;
import com.vaadin.flow.component.HasSize;
import com.vaadin.flow.component.customfield.CustomField;
import com.vaadin.flow.component.icon.Icon;
import com.vaadin.flow.component.icon.VaadinIcon;
import com.vaadin.flow.component.orderedlayout.FlexComponent;
import com.vaadin.flow.component.orderedlayout.HorizontalLayout;
import com.vaadin.flow.component.orderedlayout.VerticalLayout;
import io.imunity.vaadin.elements.VaadinElementReadOnlySetter;
import java.util.*;
import java.util.function.Function;
import java.util.stream.Collectors;
import static io.imunity.vaadin.elements.CSSVars.RICH_FIELD_BIG;
import static io.imunity.vaadin.elements.CssClassNames.POINTER;
import static io.imunity.vaadin.elements.CssClassNames.SMALL_GAP;
public class LocalizedRichTextEditorDetails extends CustomField
© 2015 - 2025 Weber Informatics LLC | Privacy Policy