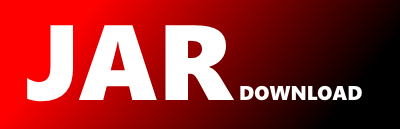
io.imunity.console.views.authentication.input_profiles.wizard.ProfileStepComponent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unity-server-console Show documentation
Show all versions of unity-server-console Show documentation
Task oriented web based administration UI
/*
* Copyright (c) 2013 ICM Uniwersytet Warszawski All rights reserved.
* See LICENCE.txt file for licensing information.
*/
package io.imunity.console.views.authentication.input_profiles.wizard;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Map;
import com.vaadin.flow.component.Unit;
import com.vaadin.flow.component.dnd.DragSource;
import com.vaadin.flow.component.grid.Grid;
import com.vaadin.flow.component.grid.GridVariant;
import com.vaadin.flow.component.html.Div;
import com.vaadin.flow.component.html.Span;
import com.vaadin.flow.component.orderedlayout.HorizontalLayout;
import com.vaadin.flow.component.orderedlayout.VerticalLayout;
import com.vaadin.flow.component.splitlayout.SplitLayout;
import io.imunity.vaadin.endpoint.common.WebSession;
import io.imunity.vaadin.endpoint.common.mvel.DragDropBean;
import io.imunity.vaadin.endpoint.common.mvel.DragStartEvent;
import io.imunity.vaadin.endpoint.common.mvel.DragEndEvent;
import io.imunity.console.tprofile.EditorContext;
import io.imunity.console.tprofile.TranslationProfileEditor;
import pl.edu.icm.unity.base.message.MessageSource;
import pl.edu.icm.unity.engine.api.authn.remote.RemotelyAuthenticatedInput;
import pl.edu.icm.unity.engine.api.translation.in.InputTranslationContextFactory;
/**
* UI Component used by {@link ProfileStep}.
*
* @author P.Piernik
*/
class ProfileStepComponent extends HorizontalLayout
{
private final MessageSource msg;
private final TranslationProfileEditor editor;
private SplitLayout splitPanelLayout;
private VerticalLayout leftPanel;
private Grid attributesTable;
private Span dragdropHint;
private VerticalLayout rightPanel;
/**
* The constructor should first build the main layout, set the composition root
* and then do any custom initialization.
*
* The constructor will not be automatically regenerated by the visual editor.
*/
ProfileStepComponent(MessageSource msg, TranslationProfileEditor editor)
{
this.msg = msg;
this.editor = editor;
editor.setContext(EditorContext.WIZARD);
buildMainLayout();
rightPanel.removeAll();
rightPanel.add(editor);
dragdropHint.getElement()
.setProperty("innerHTML", msg.getMessage("Wizard.ProfileStepComponent.dragdropHint"));
}
public void handle(RemotelyAuthenticatedInput authnInput)
{
initializeAttributesTable(authnInput);
editor.setRemoteAuthnInput(authnInput);
}
private void initializeAttributesTable(RemotelyAuthenticatedInput input)
{
Collection values = getTableContent(input);
attributesTable.setItems(values);
attributesTable.setAllRowsVisible(true);
}
private Collection getTableContent(RemotelyAuthenticatedInput input)
{
Collection items = new ArrayList<>();
Map exprValMap = InputTranslationContextFactory.createExpresionValueMap(input);
for (Map.Entry exprE : exprValMap.entrySet())
{
items.add(new DragDropBean(exprE.getKey(), exprE.getValue()));
}
return items;
}
private void buildMainLayout()
{
setSizeFull();
splitPanelLayout = buildSplitPanelLayout();
add(splitPanelLayout);
}
private SplitLayout buildSplitPanelLayout()
{
// common part: create layout
splitPanelLayout = new SplitLayout();
splitPanelLayout.setSizeFull();
splitPanelLayout.setSplitterPosition(60);
// rightPanel
rightPanel = new VerticalLayout();
rightPanel.setSpacing(false);
rightPanel.setSizeFull();
rightPanel.setMaxHeight(44,Unit.EM);
splitPanelLayout.addToPrimary(rightPanel);
// leftPanel
leftPanel = buildLeftPanel();
splitPanelLayout.addToSecondary(leftPanel);
return splitPanelLayout;
}
private VerticalLayout buildLeftPanel()
{
// common part: create layout
leftPanel = new VerticalLayout();
leftPanel.setSpacing(false);
leftPanel.setSizeFull();
leftPanel.setMaxHeight(44,Unit.EM);
// dragdropHint
dragdropHint = new Span();
leftPanel.add(dragdropHint);
// attributesTable
attributesTable = new Grid<>();
attributesTable.addThemeVariants(GridVariant.LUMO_COMPACT);
attributesTable.addComponentColumn(d -> getElementDrag(d))
.setHeader(msg.getMessage("Wizard.ProfileStepComponent.expression")).setResizable(true).setAutoWidth(true);
attributesTable.addColumn(DragDropBean::getValue)
.setHeader(msg.getMessage("Wizard.ProfileStepComponent.value")).setResizable(true).setAutoWidth(true);
leftPanel.add(attributesTable);
return leftPanel;
}
private Div getElementDrag(DragDropBean text)
{
Div box = new Div();
box.setText(text.getExpression());
DragSource boxDragSource = DragSource.create(box);
boxDragSource.setDraggable(true);
boxDragSource.setDragData(text);
boxDragSource.addDragStartListener(e -> WebSession.getCurrent()
.getEventBus()
.fireEvent(new DragStartEvent()));
boxDragSource.addDragEndListener(e -> WebSession.getCurrent()
.getEventBus()
.fireEvent(new DragEndEvent()));
return box;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy