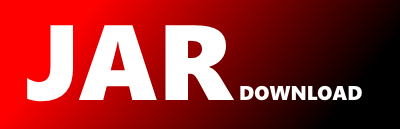
pl.edu.icm.unity.oauth.as.console.OAuthServiceEditor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unity-server-oauth Show documentation
Show all versions of unity-server-oauth Show documentation
Client and server OAuth support
The newest version!
/*
* Copyright (c) 2021 Bixbit - Krzysztof Benedyczak. All rights reserved.
* See LICENCE.txt file for licensing information.
*/
package pl.edu.icm.unity.oauth.as.console;
import io.imunity.console.utils.tprofile.OutputTranslationProfileFieldFactory;
import io.imunity.vaadin.elements.NotificationPresenter;
import io.imunity.vaadin.endpoint.common.api.HtmlTooltipFactory;
import io.imunity.vaadin.endpoint.common.api.SubViewSwitcher;
import io.imunity.vaadin.auth.services.ServiceDefinition;
import io.imunity.vaadin.auth.services.ServiceEditor;
import io.imunity.vaadin.auth.services.ServiceEditorComponent;
import io.imunity.vaadin.auth.services.idp.IdpEditorUsersTab;
import io.imunity.vaadin.auth.services.idp.IdpUser;
import io.imunity.vaadin.auth.services.idp.PolicyAgreementsTab;
import io.imunity.vaadin.auth.services.tabs.WebServiceAuthenticationTab;
import io.imunity.vaadin.endpoint.common.forms.VaadinLogoImageLoader;
import pl.edu.icm.unity.base.authn.AuthenticationFlowDefinition;
import pl.edu.icm.unity.base.group.Group;
import pl.edu.icm.unity.base.identity.IdentityType;
import pl.edu.icm.unity.base.message.MessageSource;
import pl.edu.icm.unity.engine.api.PKIManagement;
import pl.edu.icm.unity.engine.api.authn.AuthenticatorInfo;
import pl.edu.icm.unity.engine.api.authn.AuthenticatorSupportService;
import pl.edu.icm.unity.engine.api.config.UnityServerConfiguration;
import pl.edu.icm.unity.engine.api.files.FileStorageService;
import pl.edu.icm.unity.engine.api.policyDocument.PolicyDocumentWithRevision;
import pl.edu.icm.unity.oauth.as.OAuthScopesService;
import pl.edu.icm.unity.oauth.as.token.OAuthTokenEndpoint;
import pl.edu.icm.unity.oauth.as.webauthz.OAuthAuthzWebEndpoint;
import io.imunity.vaadin.endpoint.common.exceptions.FormValidationException;
import java.util.Collection;
import java.util.List;
import java.util.Set;
import java.util.function.Function;
class OAuthServiceEditor implements ServiceEditor
{
private final MessageSource msg;
private final List allRealms;
private final List flows;
private final List authenticators;
private OAuthServiceEditorComponent editor;
private final List allAttributes;
private final List allGroups;
private final List allIdpUsers;
private final Function> systemClientsSupplier;
private final List registrationForms;
private final Set credentials;
private final VaadinLogoImageLoader imageService;
private final FileStorageService fileStorageService;
private final UnityServerConfiguration serverConfig;
private final AuthenticatorSupportService authenticatorSupportService;
private final String serverPrefix;
private final Set serverContextPaths;
private final Collection idTypes;
private final SubViewSwitcher subViewSwitcher;
private final OutputTranslationProfileFieldFactory outputTranslationProfileFieldFactory;
private final List usedPaths;
private final List allUsernames;
private final NotificationPresenter notificationPresenter;
private final Collection policyDocuments;
private final OAuthScopesService scopeService;
private final Set validators;
private final Set certificates;
private final HtmlTooltipFactory htmlTooltipFactory;
private final PKIManagement pkiManagement;
OAuthServiceEditor(MessageSource msg,
SubViewSwitcher subViewSwitcher,
OutputTranslationProfileFieldFactory outputTranslationProfileFieldFactory,
PKIManagement pkiManagement,
String serverPrefix,
Set serverContextPaths,
VaadinLogoImageLoader imageService,
NotificationPresenter notificationPresenter,
FileStorageService fileStorageService,
UnityServerConfiguration serverConfig,
List allRealms,
List flows,
List authenticators,
List allAttributes,
List allGroups,
List allIdpUsers,
Function> systemClientsSupplier,
List allUsernames,
List registrationForms,
Set credentials,
AuthenticatorSupportService authenticatorSupportService,
Collection idTypes,
List usedPaths,
Collection policyDocuments,
OAuthScopesService scopeService,
Set validators,
Set certificates, HtmlTooltipFactory htmlTooltipFactory)
{
this.msg = msg;
this.pkiManagement = pkiManagement;
this.notificationPresenter = notificationPresenter;
this.allRealms = allRealms;
this.authenticators = authenticators;
this.flows = flows;
this.allAttributes = allAttributes;
this.allGroups = allGroups;
this.registrationForms = registrationForms;
this.imageService = imageService;
this.fileStorageService = fileStorageService;
this.serverConfig = serverConfig;
this.authenticatorSupportService = authenticatorSupportService;
this.credentials = credentials;
this.serverPrefix = serverPrefix;
this.serverContextPaths = serverContextPaths;
this.idTypes = idTypes;
this.subViewSwitcher = subViewSwitcher;
this.outputTranslationProfileFieldFactory = outputTranslationProfileFieldFactory;
this.usedPaths = usedPaths;
this.allIdpUsers = allIdpUsers;
this.systemClientsSupplier = systemClientsSupplier;
this.allUsernames = allUsernames;
this.policyDocuments = policyDocuments;
this.scopeService = scopeService;
this.validators = validators;
this.certificates = certificates;
this.htmlTooltipFactory = htmlTooltipFactory;
}
@Override
public ServiceEditorComponent getEditor(ServiceDefinition endpoint)
{
OAuthEditorGeneralTab generalTab = new OAuthEditorGeneralTab(msg, pkiManagement, htmlTooltipFactory, serverPrefix, serverContextPaths,
subViewSwitcher, outputTranslationProfileFieldFactory, endpoint != null, credentials, idTypes,
allAttributes, usedPaths, scopeService.getSystemScopes(), validators, certificates);
OAuthEditorClientsTab clientsTab = new OAuthEditorClientsTab(msg, serverConfig,
subViewSwitcher, flows, authenticators, allRealms, allUsernames, generalTab::getScopes,
OAuthTokenEndpoint.TYPE.getSupportedBinding(), notificationPresenter);
WebServiceAuthenticationTab webAuthTab = new WebServiceAuthenticationTab(msg, serverConfig,
authenticatorSupportService, flows, authenticators, allRealms, registrationForms,
OAuthAuthzWebEndpoint.Factory.TYPE.getSupportedBinding(),
msg.getMessage("IdpServiceEditorBase.authentication"));
IdpEditorUsersTab usersTab = new IdpEditorUsersTab(msg, allGroups, allIdpUsers,
allAttributes);
PolicyAgreementsTab policyAgreementTab = new PolicyAgreementsTab(msg, policyDocuments);
editor = new OAuthServiceEditorComponent(msg, pkiManagement, generalTab, clientsTab, usersTab, webAuthTab, policyAgreementTab,
fileStorageService, imageService, scopeService, endpoint, allGroups, systemClientsSupplier);
return editor;
}
@Override
public ServiceDefinition getEndpointDefiniton() throws FormValidationException
{
return editor.getServiceDefiniton();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy