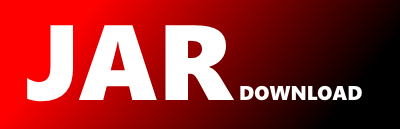
pl.edu.icm.unity.oauth.as.webauthz.OAuthSessionService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unity-server-oauth Show documentation
Show all versions of unity-server-oauth Show documentation
Client and server OAuth support
The newest version!
/*
* Copyright (c) 2021 Bixbit - Krzysztof Benedyczak. All rights reserved.
* See LICENCE.txt file for licensing information.
*/
package pl.edu.icm.unity.oauth.as.webauthz;
import java.util.Optional;
import org.apache.logging.log4j.Logger;
import org.springframework.stereotype.Component;
import com.nimbusds.oauth2.sdk.AuthorizationResponse;
import com.vaadin.flow.server.WrappedSession;
import io.imunity.vaadin.auth.server.ProxyAuthenticationFilter;
import io.imunity.vaadin.endpoint.common.consent_utils.LoginInProgressService;
import io.imunity.vaadin.endpoint.common.consent_utils.LoginInProgressService.SignInContextKey;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpSession;
import pl.edu.icm.unity.base.utils.Log;
import pl.edu.icm.unity.engine.api.authn.InvocationContext;
import pl.edu.icm.unity.engine.api.authn.LoginSession;
import pl.edu.icm.unity.engine.api.session.SessionManagement;
import pl.edu.icm.unity.oauth.as.OAuthAuthzContext;
/**
* Provides unified API to perform OAuth session bootstrap and cleanup.
*/
@Component
class OAuthSessionService
{
/**
* Under this key the OAuthContext object is stored in the session.
*/
private static final String SESSION_OAUTH_CONTEXT = "oauth2AuthnContextKey";
private static final Logger LOG = Log.getLogger(Log.U_SERVER_OAUTH, OAuthSessionService.class);
private static final LoginInProgressService LOGIN_IN_PROGRESS_SERVICE = new LoginInProgressService<>(
SESSION_OAUTH_CONTEXT);
static final String URL_PARAM_CONTEXT_KEY = LoginInProgressService.URL_PARAM_CONTEXT_KEY;
private final SessionManagement sessionMan;
OAuthSessionService(SessionManagement sessionMan)
{
this.sessionMan = sessionMan;
}
static void putExistingContextUnderNewKey(WrappedSession session, SignInContextKey existingKey, SignInContextKey newKey)
{
LOGIN_IN_PROGRESS_SERVICE.putExistingContextUnderNewKey(session, existingKey, newKey);
}
static LoginInProgressService.SignInContextKey setContext(HttpSession session, OAuthAuthzContext context)
{
return LOGIN_IN_PROGRESS_SERVICE.setContext(session, context);
}
static Optional getContext(HttpServletRequest req)
{
return LOGIN_IN_PROGRESS_SERVICE.getContext(req);
}
static OAuthAuthzContext getVaadinContext()
{
return LOGIN_IN_PROGRESS_SERVICE.getVaadinContext();
}
static boolean hasVaadinContext()
{
return LOGIN_IN_PROGRESS_SERVICE.hasVaadinContext();
}
void cleanupComplete(Optional session, boolean invalidateSSOSession)
{
cleanupBeforeResponseSent(session);
cleanupAfterResponseSent(session, invalidateSSOSession);
}
void cleanupBeforeResponseSent(Optional session)
{
session.ifPresent(ses -> cleanupBeforeResponseSent(ses));
}
void cleanupAfterResponseSent(Optional session, boolean invalidateSSOSession)
{
cleanupAfterResponseSent(session.orElse(null), invalidateSSOSession);
}
void cleanupBeforeResponseSent(LoginInProgressService.SignInContextSession session)
{
LOG.trace("Cleaning OAuth session auto-proxy state");
session.removeAttribute(ProxyAuthenticationFilter.AUTOMATED_LOGIN_FIRED);
}
void cleanupAfterResponseSent(LoginInProgressService.SignInContextSession session, boolean invalidateSSOSession)
{
LOG.trace("Cleaning OAuth session (sso logout={})", invalidateSSOSession);
LOGIN_IN_PROGRESS_SERVICE.cleanUpSignInContextAttribute(session);
if (session != null)
{
session.removeAttribute(AuthorizationResponse.class.getName());
}
if (invalidateSSOSession)
{
LoginSession loginSession = InvocationContext.getCurrent().getLoginSession();
sessionMan.removeSession(loginSession.getId(), true);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy