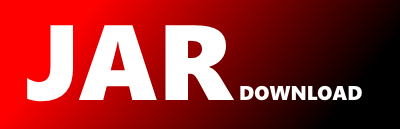
io.imunity.rest.mappers.registration.invite.PrefilledEntryMapper Maven / Gradle / Ivy
/*
* Copyright (c) 2021 Bixbit - Krzysztof Benedyczak. All rights reserved.
* See LICENCE.txt file for licensing information.
*/
package io.imunity.rest.mappers.registration.invite;
import java.util.function.Function;
import io.imunity.rest.api.types.registration.invite.RestPrefilledEntry;
import pl.edu.icm.unity.base.registration.invitation.PrefilledEntry;
import pl.edu.icm.unity.base.registration.invitation.PrefilledEntryMode;
public class PrefilledEntryMapper
{
public static RestPrefilledEntry map(PrefilledEntry prefilledEntry, Function entryMapper)
{
return new RestPrefilledEntry.Builder().withEntry(entryMapper.apply(prefilledEntry.getEntry()))
.withMode(prefilledEntry.getMode()
.name())
.build();
}
public static RestPrefilledEntry map(PrefilledEntry prefilledEntry, T entry)
{
return new RestPrefilledEntry.Builder().withEntry(entry)
.withMode(prefilledEntry.getMode()
.name())
.build();
}
public static PrefilledEntry map(RestPrefilledEntry prefilledEntry, Function entryMapper)
{
return new PrefilledEntry(entryMapper.apply(prefilledEntry.entry),
PrefilledEntryMode.valueOf(prefilledEntry.mode));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy