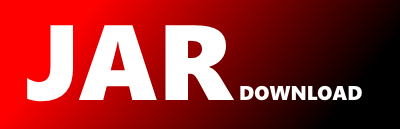
io.imunity.vaadin.endpoint.common.BaseSpringInstantiator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unity-server-vaadin-endpoint-common Show documentation
Show all versions of unity-server-vaadin-endpoint-common Show documentation
Common Vaadin endpoint components
The newest version!
/*
* Copyright (c) 2021 Bixbit - Krzysztof Benedyczak. All rights reserved.
* See LICENCE.txt file for licensing information.
*/
package io.imunity.vaadin.endpoint.common;
import java.util.stream.Stream;
import org.springframework.beans.BeanInstantiationException;
import org.springframework.context.ApplicationContext;
import com.vaadin.flow.component.Component;
import com.vaadin.flow.di.DefaultInstantiator;
import com.vaadin.flow.server.VaadinService;
import com.vaadin.flow.server.VaadinServiceInitListener;
import pl.edu.icm.unity.base.message.MessageSource;
public class BaseSpringInstantiator extends DefaultInstantiator
{
private final ApplicationContext context;
public BaseSpringInstantiator(VaadinService service, ApplicationContext context)
{
super(service);
this.context = context;
}
@Override
public Stream getServiceInitListeners()
{
return Stream.concat(super.getServiceInitListeners(), Stream.of(
new RedirectUrlSessionStorageServiceInitListener(),
new CustomErrorPageInitializer(context.getBean(MessageSource.class)),
new CustomStylesInitializer(context.getBean(DefaultCssFileLoader.class),
context.getBean(GlobalCustomCssFileLoader.class)))
);
}
@Override
public T createComponent(Class componentClass)
{
return context.getAutowireCapableBeanFactory().createBean(componentClass);
}
@Override
public T getOrCreate(Class type)
{
if (context.getBeanNamesForType(type).length == 1)
{
return context.getBean(type);
} else if (context.getBeanNamesForType(type).length > 1)
{
return createBean(type);
} else
{
return context.getAutowireCapableBeanFactory().createBean(type);
}
}
private T createBean(Class type)
{
try {
return context.getAutowireCapableBeanFactory().createBean(type);
} catch (BeanInstantiationException e) {
throw new BeanInstantiationException(e.getBeanClass(), "Probably more than one suitable beans for in the context.", e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy