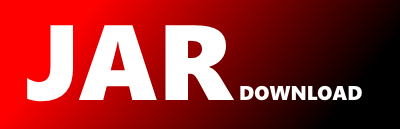
io.imunity.vaadin.endpoint.common.Toolbar Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unity-server-vaadin-endpoint-common Show documentation
Show all versions of unity-server-vaadin-endpoint-common Show documentation
Common Vaadin endpoint components
The newest version!
/*
* Copyright (c) 2019 Bixbit - Krzysztof Benedyczak. All rights reserved.
* See LICENCE.txt file for licensing information.
*/
package io.imunity.vaadin.endpoint.common;
import com.google.common.html.HtmlEscapers;
import com.vaadin.flow.component.button.Button;
import com.vaadin.flow.component.orderedlayout.FlexComponent;
import com.vaadin.flow.component.orderedlayout.HorizontalLayout;
import io.imunity.vaadin.elements.SearchField;
import io.imunity.vaadin.elements.grid.ActionMenuWithHandlerSupport;
import io.imunity.vaadin.elements.grid.SingleActionHandler;
import java.util.*;
import java.util.function.Consumer;
/**
* Component with a list of small buttons. Buttons are bound to actions via
* {@link SingleActionHandler}.
*
* Additionally toolbar's buttons have their state enabled or disabled depending
* whether the toolbar's target is set or not.
*
* @author P.Piernik
*/
public class Toolbar extends HorizontalLayout
{
private Set target;
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy