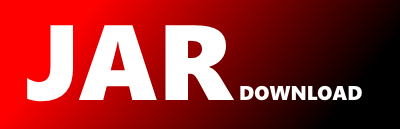
io.imunity.vaadin.endpoint.common.forms.PrefilledSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unity-server-vaadin-endpoint-common Show documentation
Show all versions of unity-server-vaadin-endpoint-common Show documentation
Common Vaadin endpoint components
The newest version!
/*
* Copyright (c) 2018 Bixbit - Krzysztof Benedyczak. All rights reserved.
* See LICENCE.txt file for licensing information.
*/
package io.imunity.vaadin.endpoint.common.forms;
import pl.edu.icm.unity.base.attribute.Attribute;
import pl.edu.icm.unity.base.identity.IdentityParam;
import pl.edu.icm.unity.base.registration.GroupSelection;
import pl.edu.icm.unity.base.registration.invitation.PrefilledEntry;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
/**
* Used by {@link BaseRequestEditor} to create UI controls. Contains
* informations that should be set as initial state for a request.
*
* @author P.Piernik
*
*/
public class PrefilledSet
{
public final Map> identities;
public final Map> groupSelections;
public final Map allowedGroups;
public final Map> attributes;
public PrefilledSet(Map> identities,
Map> groupSelections,
Map> attributes, Map allowedGroups)
{
this.identities = Collections.unmodifiableMap(identities != null ? identities : new HashMap<>());
this.groupSelections = Collections
.unmodifiableMap(groupSelections != null ? groupSelections : new HashMap<>());
this.attributes = Collections.unmodifiableMap(attributes != null ? attributes : new HashMap<>());
this.allowedGroups = Collections
.unmodifiableMap(allowedGroups != null ? allowedGroups : new HashMap<>());
}
public PrefilledSet()
{
this(null, null, null, null);
}
/**
* @return a new object which has union of pre-filled settings of this and argument object. In case of conflicts
* this object's values stay intact.
*/
public PrefilledSet mergeWith(PrefilledSet other)
{
return new PrefilledSet(mergeIdentities(other.identities),
mergeGroups(other.groupSelections),
mergeAttributes(other.attributes),
mergeAllowedGroups(other.allowedGroups));
}
private Map mergeAllowedGroups(Map otherAllowedGroups)
{
Map ret = new HashMap<>(otherAllowedGroups);
ret.putAll(allowedGroups);
return ret;
}
private Map> mergeAttributes(
Map> otherAttributes)
{
Map> ret = new HashMap<>(otherAttributes);
ret.putAll(attributes);
return ret;
}
private Map> mergeGroups(
Map> otherGroupSelections)
{
Map> ret = new HashMap<>(otherGroupSelections);
ret.putAll(groupSelections);
return ret;
}
private Map> mergeIdentities(
Map> otherIdentities)
{
Map> ret = new HashMap<>(otherIdentities);
ret.putAll(identities);
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy