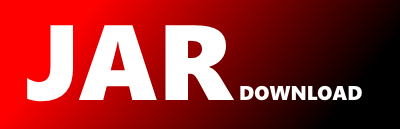
io.imunity.vaadin.endpoint.common.forms.URLQueryPrefillCreator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unity-server-vaadin-endpoint-common Show documentation
Show all versions of unity-server-vaadin-endpoint-common Show documentation
Common Vaadin endpoint components
The newest version!
/*
* Copyright (c) 2020 Bixbit - Krzysztof Benedyczak. All rights reserved.
* See LICENCE.txt file for licensing information.
*/
package io.imunity.vaadin.endpoint.common.forms;
import com.google.common.collect.Lists;
import org.apache.logging.log4j.Logger;
import org.springframework.stereotype.Component;
import pl.edu.icm.unity.base.attribute.Attribute;
import pl.edu.icm.unity.base.attribute.IllegalAttributeValueException;
import pl.edu.icm.unity.base.identity.IdentityParam;
import pl.edu.icm.unity.base.identity.IllegalIdentityValueException;
import pl.edu.icm.unity.base.registration.AttributeRegistrationParam;
import pl.edu.icm.unity.base.registration.BaseForm;
import pl.edu.icm.unity.base.registration.IdentityRegistrationParam;
import pl.edu.icm.unity.base.registration.URLQueryPrefillConfig;
import pl.edu.icm.unity.base.registration.invitation.PrefilledEntry;
import pl.edu.icm.unity.base.utils.Log;
import pl.edu.icm.unity.engine.api.translation.ExternalDataParser;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static java.util.Collections.emptyList;
@Component
public class URLQueryPrefillCreator
{
private static final Logger log = Log.getLogger(Log.U_SERVER_WEB, URLQueryPrefillCreator.class);
private final ExternalDataParser externalDataParser;
URLQueryPrefillCreator(ExternalDataParser externalDataParser)
{
this.externalDataParser = externalDataParser;
}
public PrefilledSet create(BaseForm form, Map> parameters)
{
return new PrefilledSet(getPrefilledIdentities(form.getIdentityParams(), parameters), null,
getPrefilledAttributes(form.getAttributeParams(), parameters), null);
}
private Map> getPrefilledAttributes(
List attributeParams, Map> parameters)
{
Map> prefilled = new HashMap<>();
for (int i = 0; i < attributeParams.size(); i++)
{
AttributeRegistrationParam attributeParam = attributeParams.get(i);
URLQueryPrefillConfig urlQueryPrefill = attributeParam.getUrlQueryPrefill();
if (urlQueryPrefill == null)
continue;
String value = parameters.getOrDefault(urlQueryPrefill.paramName, emptyList()).stream().findFirst().orElse(null);
if (value == null)
continue;
Attribute prefilledAttribute;
try
{
prefilledAttribute = externalDataParser.parseAsAttribute(
attributeParam.getAttributeType(),
attributeParam.getGroup(), Lists.newArrayList(value));
} catch (IllegalAttributeValueException e)
{
log.warn("Can't parse query parameter as pre-filled form attribute", e);
continue;
}
prefilled.put(i, new PrefilledEntry<>(prefilledAttribute, urlQueryPrefill.mode));
}
return prefilled;
}
private Map> getPrefilledIdentities(
List identityParams, Map> parameters)
{
Map> prefilled = new HashMap<>();
for (int i = 0; i < identityParams.size(); i++)
{
IdentityRegistrationParam param = identityParams.get(i);
URLQueryPrefillConfig urlQueryPrefill = param.getUrlQueryPrefill();
if (urlQueryPrefill == null)
continue;
String value = parameters.getOrDefault(urlQueryPrefill.paramName, emptyList()).stream().findFirst().orElse(null);
if (value == null)
continue;
IdentityParam prefilledEntry;
try
{
prefilledEntry = externalDataParser.parseAsIdentity(param.getIdentityType(), value);
} catch (IllegalIdentityValueException e)
{
log.warn("Can't parse query parameter as pre-filled form identity", e);
continue;
}
prefilled.put(i, new PrefilledEntry<>(prefilledEntry, urlQueryPrefill.mode));
}
return prefilled;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy