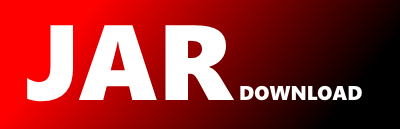
pl.edu.icm.unity.home.console.HomeServiceController Maven / Gradle / Ivy
/*
* Copyright (c) 2019 Bixbit - Krzysztof Benedyczak. All rights reserved.
* See LICENCE.txt file for licensing information.
*/
package pl.edu.icm.unity.home.console;
import java.util.stream.Collectors;
import org.springframework.stereotype.Component;
import pl.edu.icm.unity.MessageSource;
import pl.edu.icm.unity.engine.api.AttributeTypeManagement;
import pl.edu.icm.unity.engine.api.AuthenticationFlowManagement;
import pl.edu.icm.unity.engine.api.AuthenticatorManagement;
import pl.edu.icm.unity.engine.api.EndpointManagement;
import pl.edu.icm.unity.engine.api.EnquiryManagement;
import pl.edu.icm.unity.engine.api.RealmsManagement;
import pl.edu.icm.unity.engine.api.RegistrationsManagement;
import pl.edu.icm.unity.engine.api.authn.AuthenticatorSupportService;
import pl.edu.icm.unity.engine.api.bulk.BulkGroupQueryService;
import pl.edu.icm.unity.engine.api.config.UnityServerConfiguration;
import pl.edu.icm.unity.engine.api.files.FileStorageService;
import pl.edu.icm.unity.engine.api.files.URIAccessService;
import pl.edu.icm.unity.engine.api.server.NetworkServer;
import pl.edu.icm.unity.exceptions.EngineException;
import pl.edu.icm.unity.home.UserHomeEndpointFactory;
import pl.edu.icm.unity.webadmin.utils.ProjectManagementHelper;
import pl.edu.icm.unity.webui.common.file.ImageAccessService;
import pl.edu.icm.unity.webui.common.webElements.SubViewSwitcher;
import pl.edu.icm.unity.webui.console.services.DefaultServicesControllerBase;
import pl.edu.icm.unity.webui.console.services.ServiceController;
import pl.edu.icm.unity.webui.console.services.ServiceEditor;
import pl.edu.icm.unity.webui.providers.HomeUITabProvider;
@Component
class HomeServiceController extends DefaultServicesControllerBase implements ServiceController
{
private RealmsManagement realmsMan;
private AuthenticationFlowManagement flowsMan;
private AuthenticatorManagement authMan;
private HomeUITabProvider tabProvider;
private AttributeTypeManagement atMan;
private BulkGroupQueryService bulkService;
private ProjectManagementHelper projectManHelper;
private EnquiryManagement enquiryMan;
private RegistrationsManagement registrationMan;
private URIAccessService uriAccessService;
private FileStorageService fileStorageService;
private UnityServerConfiguration serverConfig;
private AuthenticatorSupportService authenticatorSupportService;
private NetworkServer server;
private ImageAccessService imageAccessService;
HomeServiceController(MessageSource msg, EndpointManagement endpointMan, RealmsManagement realmsMan,
AuthenticationFlowManagement flowsMan, AuthenticatorManagement authMan,
HomeUITabProvider tabProvider, AttributeTypeManagement atMan, BulkGroupQueryService bulkService,
ProjectManagementHelper projectManagementHelper, EnquiryManagement enquiryMan,
RegistrationsManagement registrationMan, URIAccessService uriAccessService,
FileStorageService fileStorageService, UnityServerConfiguration serverConfig,
AuthenticatorSupportService authenticatorSupportService, NetworkServer server,
ImageAccessService imageAccessService)
{
super(msg, endpointMan);
this.realmsMan = realmsMan;
this.flowsMan = flowsMan;
this.authMan = authMan;
this.tabProvider = tabProvider;
this.atMan = atMan;
this.bulkService = bulkService;
this.projectManHelper = projectManagementHelper;
this.enquiryMan = enquiryMan;
this.registrationMan = registrationMan;
this.uriAccessService = uriAccessService;
this.fileStorageService = fileStorageService;
this.serverConfig = serverConfig;
this.authenticatorSupportService = authenticatorSupportService;
this.server = server;
this.imageAccessService = imageAccessService;
}
@Override
public String getSupportedEndpointType()
{
return UserHomeEndpointFactory.NAME;
}
@Override
public ServiceEditor getEditor(SubViewSwitcher subViewSwitcher) throws EngineException
{
return new HomeServiceEditor(msg, uriAccessService, imageAccessService, fileStorageService, serverConfig,
realmsMan.getRealms().stream().map(r -> r.getName()).collect(Collectors.toList()),
flowsMan.getAuthenticationFlows().stream().collect(Collectors.toList()),
authMan.getAuthenticators(null).stream().collect(Collectors.toList()),
tabProvider.getId(),
atMan.getAttributeTypes().stream().map(a -> a.getName()).collect(Collectors.toList()),
bulkService.getGroupAndSubgroups(bulkService.getBulkStructuralData("/")).values()
.stream().map(g -> g.getGroup()).collect(Collectors.toList()),
projectManHelper.getAllProjectManEndpoints().stream().map(e -> e.getName())
.collect(Collectors.toList()),
enquiryMan.getEnquires().stream().map(e -> e.getName()).collect(Collectors.toList()),
registrationMan.getForms().stream().filter(r -> r.isPubliclyAvailable())
.map(r -> r.getName()).collect(Collectors.toList()),
endpointMan.getEndpoints().stream().map(e -> e.getContextAddress())
.collect(Collectors.toList()), server.getUsedContextPaths(),
authenticatorSupportService);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy