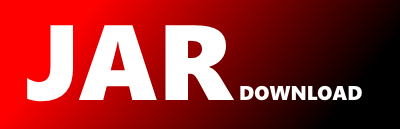
commonMain.org.koin.compose.viewmodel.dsl.ScopeViewModelOf.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of koin-compose-viewmodel-jvm Show documentation
Show all versions of koin-compose-viewmodel-jvm Show documentation
KOIN - Kotlin simple Dependency Injection Framework
The newest version!
/*
* Copyright 2017-2023 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.koin.compose.viewmodel.dsl
import androidx.lifecycle.ViewModel
import org.koin.core.annotation.KoinInternalApi
import org.koin.core.definition.KoinDefinition
import org.koin.core.module.dsl.DefinitionOptions
import org.koin.core.module.dsl.new
import org.koin.core.module.dsl.onOptions
import org.koin.dsl.ScopeDSL
//TODO DEPRECATE in favor of koin-viewmodel to mutualise ViewModel DSL for Android and Compose
/**
* Declare a [ScopeDSL.viewModel] definition by resolving a constructor reference for the dependency.
* The resolution is done at compile time by leveraging inline functions, no reflection is required.
*
* Example:
* ```
* class MyViewModel : ViewModel()
*
* val myModule = module {
* viewModelOf(::MyViewModel)
* }
* ```
*
* @author Arnaud Giuliani
*
* @see new - function operator
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: () -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
/**
* @see viewModelOf
*/
@Deprecated("Moved ViewModel DSL package. Remove old imports and use org.koin.core.module.dsl.*")
inline fun ScopeDSL.viewModelOf(
crossinline constructor: (T1, T2, T3, T4, T5, T6, T7, T8, T9, T10, T11, T12, T13, T14, T15, T16, T17, T18, T19, T20, T21, T22) -> R,
noinline options: DefinitionOptions? = null,
): KoinDefinition = viewModel { new(constructor) }.onOptions(options)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy