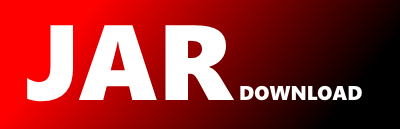
io.interact.jedis.pojo.CacheService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jedis-pojo Show documentation
Show all versions of jedis-pojo Show documentation
Simple self-loading pojo cache service based on Jedis.
package io.interact.jedis.pojo;
import java.util.function.Supplier;
/**
* A self-loading cache service based on Redis.
*
* @author Bas Cancrinus - interact.io
*/
public interface CacheService {
/**
* Put the supplied key-value pair in cache.
*
* @param key
* Key to cached value.
* @param value
* Value to cache. * @param ttl Set the cached TTL to the
* supplied value or don't set the TTL when the supplied value is
* null.
* @param ttl
* Set the cached TTL to the supplied value or don't set the TTL
* when the supplied value is null.
*/
void put(String key, Object value, Integer ttl);
/**
* Convenience method that calls
* {@link CacheService#put(String, Object, Integer)} without a TTL.
*
* @param key
* Key to cached value.
* @param value
* Value to cache. * @param ttl Set the cached TTL to the
* supplied value or don't set the TTL when the supplied value is
* null.
*/
void put(String key, Object value);
/**
* Convenience method that calls
* {@link CacheService#get(String, Supplier, Class, Integer)} without a TTL.
*
* @param
* The pojo that is loaded or cached.
* @param key
* Key used to lookup and save.
* @param loader
* Supplies the value when it is not cached.
* @param out
* Class of the cached or loaded value.
* @return Loaded or cached value.
*/
T get(String key, Supplier loader, Class out);
/**
* Get cached value by the supplied key. When that value is not found, then
* get the value from the supplied loader and put it in the cache with the
* supplied TTL in seconds.
*
* @param
* The pojo that is loaded or cached.
* @param key
* Key used to lookup and save.
* @param loader
* Supplies the value when it is not cached.
* @param out
* Class of the cached or loaded value.
* @param ttl
* Set the cached TTL to the supplied value or don't set the TTL
* when the supplied value is null.
* @return Loaded or cached value.
*/
T get(String key, Supplier loader, Class out, Integer ttl);
/**
* Evict value by the supplied key from cache.
*
* @param key
* Key of the cached value to evict.
*/
void evict(String key);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy