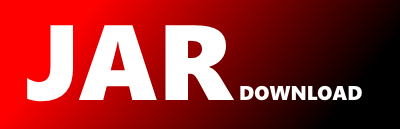
io.intercom.api.DataResource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of intercom-java Show documentation
Show all versions of intercom-java Show documentation
Java bindings for the Intercom API
The newest version!
package io.intercom.api;
import com.google.common.base.Strings;
import com.google.common.collect.Maps;
import java.net.URI;
import java.util.List;
import java.util.Map;
abstract class DataResource {
@SuppressWarnings("SameParameterValue")
public static T find(String id, String collectionPath, Class c) {
final HttpClient resource = new HttpClient(UriBuilder.newBuilder().path(collectionPath).path(id).build());
return resource.get(c);
}
public static T find(Map params, String collectionPath, Class c) {
final HttpClient resource = new HttpClient(UriBuilder.newBuilder().path(collectionPath).query(params).build());
return resource.get(c);
}
public static R create(T entity, String collectionPath, Class response) {
final HttpClient resource = new HttpClient(UriBuilder.newBuilder().path(collectionPath).build());
return resource.post(response, entity);
}
public static R create(T entity, List paths, Class response) {
final HttpClient resource = new HttpClient(UriBuilder.newBuilder().path(paths).build());
return resource.post(response, entity);
}
public static R update(T entity, String collectionPath, Class response) {
final HttpClient resource = new HttpClient(UriBuilder.newBuilder().path(collectionPath).build());
return resource.post(response, entity);
}
public static R post(T entity, URI path, Class response) {
final HttpClient resource = new HttpClient(path);
return resource.post(response, entity);
}
public static R updatePut(T entity, URI collectionPath, Class response) {
final HttpClient resource = new HttpClient(collectionPath);
return resource.put(response, entity);
}
@SuppressWarnings("SameParameterValue")
public static R update(T entity, String collectionPath, String id, Class response) {
final HttpClient resource = new HttpClient(UriBuilder.newBuilder().path(collectionPath).path(id).build());
return resource.post(response, entity);
}
public static T delete(String id, String collectionPath, Class c) {
final HttpClient resource = new HttpClient(UriBuilder.newBuilder().path(collectionPath).path(id).build());
return resource.delete(c);
}
public static T delete(Map params, String collectionPath, Class c) {
final HttpClient resource = new HttpClient(UriBuilder.newBuilder().path(collectionPath).query(params).build());
return resource.delete(c);
}
public static C list(Map params, String collectionPath, Class c) {
final HttpClient resource = new HttpClient(UriBuilder.newBuilder().path(collectionPath).query(params).build());
return resource.get(c);
}
public static C scroll(String scrollParam, String collectionPath, Class c) {
Map params = Maps.newHashMap();
if (!Strings.isNullOrEmpty(scrollParam)) {
params.put("scroll_param", scrollParam);
}
final HttpClient resource = new HttpClient(UriBuilder.newBuilder().path(collectionPath).path("scroll").query(params).build());
return resource.get(c);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy