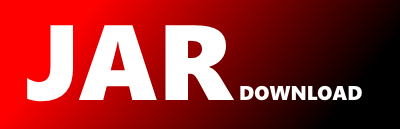
io.inverno.mod.boot.BootNetServerConfigurationLoader Maven / Gradle / Ivy
package io.inverno.mod.boot;
import io.inverno.mod.configuration.ConfigurationLoaderSupport;
import java.lang.Boolean;
import java.lang.Integer;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.function.Supplier;
import javax.annotation.processing.Generated;
@Generated(value="io.inverno.mod.configuration.compiler.internal.ConfigurationCompilerPlugin", date = "2024-03-06T16:16:01.416332013+01:00[Europe/Paris]")
public final class BootNetServerConfigurationLoader extends ConfigurationLoaderSupport {
public BootNetServerConfigurationLoader() {
super(
new String[] {
"io.inverno.mod.boot.bootNetServerConfiguration.reuse_address",
"io.inverno.mod.boot.bootNetServerConfiguration.reuse_port",
"io.inverno.mod.boot.bootNetServerConfiguration.keep_alive",
"io.inverno.mod.boot.bootNetServerConfiguration.tcp_no_delay",
"io.inverno.mod.boot.bootNetServerConfiguration.tcp_quickack",
"io.inverno.mod.boot.bootNetServerConfiguration.tcp_cork",
"io.inverno.mod.boot.bootNetServerConfiguration.linger",
"io.inverno.mod.boot.bootNetServerConfiguration.rcv_buffer",
"io.inverno.mod.boot.bootNetServerConfiguration.snd_buffer",
"io.inverno.mod.boot.bootNetServerConfiguration.ip_tos",
"io.inverno.mod.boot.bootNetServerConfiguration.accept_backlog",
"io.inverno.mod.boot.bootNetServerConfiguration.tcp_fast_open"
},
results -> bootNetServerConfiguration_configurator -> {
results.get(0).getResult().ifPresent(property -> bootNetServerConfiguration_configurator.reuse_address(property.asBoolean().orElseGet(() -> (new boolean[1])[0])));
results.get(1).getResult().ifPresent(property -> bootNetServerConfiguration_configurator.reuse_port(property.asBoolean().orElseGet(() -> (new boolean[1])[0])));
results.get(2).getResult().ifPresent(property -> bootNetServerConfiguration_configurator.keep_alive(property.asBoolean().orElseGet(() -> (new boolean[1])[0])));
results.get(3).getResult().ifPresent(property -> bootNetServerConfiguration_configurator.tcp_no_delay(property.asBoolean().orElseGet(() -> (new boolean[1])[0])));
results.get(4).getResult().ifPresent(property -> bootNetServerConfiguration_configurator.tcp_quickack(property.asBoolean().orElseGet(() -> (new boolean[1])[0])));
results.get(5).getResult().ifPresent(property -> bootNetServerConfiguration_configurator.tcp_cork(property.asBoolean().orElseGet(() -> (new boolean[1])[0])));
results.get(6).getResult().ifPresent(property -> bootNetServerConfiguration_configurator.linger(property.as(Integer.class).orElse(null)));
results.get(7).getResult().ifPresent(property -> bootNetServerConfiguration_configurator.rcv_buffer(property.as(Integer.class).orElse(null)));
results.get(8).getResult().ifPresent(property -> bootNetServerConfiguration_configurator.snd_buffer(property.as(Integer.class).orElse(null)));
results.get(9).getResult().ifPresent(property -> bootNetServerConfiguration_configurator.ip_tos(property.as(Integer.class).orElse(null)));
results.get(10).getResult().ifPresent(property -> bootNetServerConfiguration_configurator.accept_backlog(property.as(Integer.class).orElse(null)));
results.get(11).getResult().ifPresent(property -> bootNetServerConfiguration_configurator.tcp_fast_open(property.as(Integer.class).orElse(null)));
},
BootNetServerConfigurationLoader::load,
BootNetServerConfigurationLoader.Configuration::new
);
}
public static BootNetServerConfiguration load(Consumer configurer) {
if(configurer == null) {
return new BootNetServerConfigurationLoader.Configuration();
}
BootNetServerConfigurationLoader.Configurator configurator = new BootNetServerConfigurationLoader.Configurator();
configurer.accept(configurator);
return new BootNetServerConfigurationLoader.Configuration(configurator.reuse_address, configurator.reuse_port, configurator.keep_alive, configurator.tcp_no_delay, configurator.tcp_quickack, configurator.tcp_cork, configurator.linger, configurator.rcv_buffer, configurator.snd_buffer, configurator.ip_tos, configurator.accept_backlog, configurator.tcp_fast_open);
}
public static BootNetServerConfiguration load(BootNetServerConfiguration configuration, Consumer configurer) {
if(configurer == null) {
return configuration;
}
Consumer rootConfigurer = configurator -> configurator
.reuse_address(configuration.reuse_address())
.reuse_port(configuration.reuse_port())
.keep_alive(configuration.keep_alive())
.tcp_no_delay(configuration.tcp_no_delay())
.tcp_quickack(configuration.tcp_quickack())
.tcp_cork(configuration.tcp_cork())
.linger(configuration.linger())
.rcv_buffer(configuration.rcv_buffer())
.snd_buffer(configuration.snd_buffer())
.ip_tos(configuration.ip_tos())
.accept_backlog(configuration.accept_backlog())
.tcp_fast_open(configuration.tcp_fast_open());
BootNetServerConfigurationLoader.Configurator configurator = new BootNetServerConfigurationLoader.Configurator();
rootConfigurer.andThen(configurer).accept(configurator);
return new BootNetServerConfigurationLoader.Configuration(configurator.reuse_address, configurator.reuse_port, configurator.keep_alive, configurator.tcp_no_delay, configurator.tcp_quickack, configurator.tcp_cork, configurator.linger, configurator.rcv_buffer, configurator.snd_buffer, configurator.ip_tos, configurator.accept_backlog, configurator.tcp_fast_open);
}
private static final class Configuration implements BootNetServerConfiguration {
private boolean reuse_address = BootNetServerConfiguration.super.reuse_address();
private boolean reuse_port = BootNetServerConfiguration.super.reuse_port();
private boolean keep_alive = BootNetServerConfiguration.super.keep_alive();
private boolean tcp_no_delay = BootNetServerConfiguration.super.tcp_no_delay();
private boolean tcp_quickack = BootNetServerConfiguration.super.tcp_quickack();
private boolean tcp_cork = BootNetServerConfiguration.super.tcp_cork();
private Integer linger;
private Integer rcv_buffer;
private Integer snd_buffer;
private Integer ip_tos;
private Integer accept_backlog = BootNetServerConfiguration.super.accept_backlog();
private Integer tcp_fast_open;
private Configuration() {}
public Configuration(Supplier reuse_address, Supplier reuse_port, Supplier keep_alive, Supplier tcp_no_delay, Supplier tcp_quickack, Supplier tcp_cork, Supplier linger, Supplier rcv_buffer, Supplier snd_buffer, Supplier ip_tos, Supplier accept_backlog, Supplier tcp_fast_open) {
Optional.ofNullable(reuse_address).ifPresent(s -> this.reuse_address = s.get());
Optional.ofNullable(reuse_port).ifPresent(s -> this.reuse_port = s.get());
Optional.ofNullable(keep_alive).ifPresent(s -> this.keep_alive = s.get());
Optional.ofNullable(tcp_no_delay).ifPresent(s -> this.tcp_no_delay = s.get());
Optional.ofNullable(tcp_quickack).ifPresent(s -> this.tcp_quickack = s.get());
Optional.ofNullable(tcp_cork).ifPresent(s -> this.tcp_cork = s.get());
Optional.ofNullable(linger).ifPresent(s -> this.linger = s.get());
Optional.ofNullable(rcv_buffer).ifPresent(s -> this.rcv_buffer = s.get());
Optional.ofNullable(snd_buffer).ifPresent(s -> this.snd_buffer = s.get());
Optional.ofNullable(ip_tos).ifPresent(s -> this.ip_tos = s.get());
Optional.ofNullable(accept_backlog).ifPresent(s -> this.accept_backlog = s.get());
Optional.ofNullable(tcp_fast_open).ifPresent(s -> this.tcp_fast_open = s.get());
}
public boolean reuse_address() {
return this.reuse_address;
}
public boolean reuse_port() {
return this.reuse_port;
}
public boolean keep_alive() {
return this.keep_alive;
}
public boolean tcp_no_delay() {
return this.tcp_no_delay;
}
public boolean tcp_quickack() {
return this.tcp_quickack;
}
public boolean tcp_cork() {
return this.tcp_cork;
}
public Integer linger() {
return this.linger;
}
public Integer rcv_buffer() {
return this.rcv_buffer;
}
public Integer snd_buffer() {
return this.snd_buffer;
}
public Integer ip_tos() {
return this.ip_tos;
}
public Integer accept_backlog() {
return this.accept_backlog;
}
public Integer tcp_fast_open() {
return this.tcp_fast_open;
}
}
public static final class Configurator {
private Supplier reuse_address;
private Supplier reuse_port;
private Supplier keep_alive;
private Supplier tcp_no_delay;
private Supplier tcp_quickack;
private Supplier tcp_cork;
private Supplier linger;
private Supplier rcv_buffer;
private Supplier snd_buffer;
private Supplier ip_tos;
private Supplier accept_backlog;
private Supplier tcp_fast_open;
private Configurator() {}
public Configurator reuse_address(boolean reuse_address) {
this.reuse_address = () -> reuse_address;
return this;
}
public Configurator reuse_port(boolean reuse_port) {
this.reuse_port = () -> reuse_port;
return this;
}
public Configurator keep_alive(boolean keep_alive) {
this.keep_alive = () -> keep_alive;
return this;
}
public Configurator tcp_no_delay(boolean tcp_no_delay) {
this.tcp_no_delay = () -> tcp_no_delay;
return this;
}
public Configurator tcp_quickack(boolean tcp_quickack) {
this.tcp_quickack = () -> tcp_quickack;
return this;
}
public Configurator tcp_cork(boolean tcp_cork) {
this.tcp_cork = () -> tcp_cork;
return this;
}
public Configurator linger(Integer linger) {
this.linger = () -> linger;
return this;
}
public Configurator rcv_buffer(Integer rcv_buffer) {
this.rcv_buffer = () -> rcv_buffer;
return this;
}
public Configurator snd_buffer(Integer snd_buffer) {
this.snd_buffer = () -> snd_buffer;
return this;
}
public Configurator ip_tos(Integer ip_tos) {
this.ip_tos = () -> ip_tos;
return this;
}
public Configurator accept_backlog(Integer accept_backlog) {
this.accept_backlog = () -> accept_backlog;
return this;
}
public Configurator tcp_fast_open(Integer tcp_fast_open) {
this.tcp_fast_open = () -> tcp_fast_open;
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy