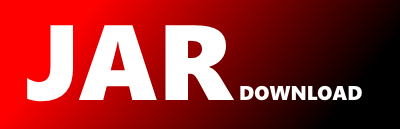
io.inverno.mod.http.server.Exchange Maven / Gradle / Ivy
/*
* Copyright 2020 Jeremy KUHN
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.inverno.mod.http.server;
import io.inverno.mod.http.base.BaseExchange;
import io.inverno.mod.http.base.ExchangeContext;
import io.inverno.mod.http.server.ws.WebSocket;
import io.inverno.mod.http.server.ws.WebSocketExchange;
import java.util.Optional;
import reactor.core.publisher.Mono;
/**
*
* Represents an HTTP server exchange (request/response) between a client and a server.
*
*
*
* An HTTP server exchange is created when a {@link Request} is received from a client by the HTTP server which then processes the exchange in the {@link ServerController}.
*
*
*
* When creating the exchange, the HTTP server also invokes {@link ServerController#createContext()} to create an exchange context and attach it to the new server exchange.
*
*
* @author Jeremy Kuhn
* @since 1.0
*
* @see Request
* @see Response
* @see ExchangeContext
*
* @param the type of the exchange context
*/
public interface Exchange extends BaseExchange {
@Override
Request request();
@Override
Response response();
/**
*
* Upgrades the exchange to a WebSocket exchange.
*
*
*
* If the exchange cannot upgrade to the WebSocket protocol, an empty optional shall be returned. For instance, if the state of the exchange prevents the upgrade (e.g. error exchange) or if the
* underlying HTTP protocol does not support the upgrade operation. Currently only HTTP/1.1 can upgrade to the WebSocket protocol.
*
*
* @param subProtocols a list of supported subprotocols negotiated during the handshake
*
* @return an optional returning the WebSocket or an empty optional if the upgrade is not possible
*/
Optional extends WebSocket>> webSocket(String... subProtocols);
/**
*
* Specifies a finalizer which completes once the exchange is fully processed.
*
*
*
* An exchange is considered fully processed when the last chunk of the response has been fully sent to the client or following a terminal error.
*
*
*
* Note that using a finalizer actually impacts HTTP pipelining since the server wait for the response to be fully sent to the client before processing subsequent requests.
*
*
*
* When invoked multiple time this method chains the finalizer one after the other.
*
*
* @param finalizer a finalizer
*/
void finalizer(Mono finalizer);
}