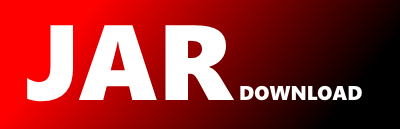
io.inverno.mod.http.server.HttpServerConfiguration Maven / Gradle / Ivy
Show all versions of inverno-http-server Show documentation
/*
* Copyright 2020 Jeremy KUHN
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.inverno.mod.http.server;
import io.inverno.mod.configuration.Configuration;
import io.inverno.mod.http.server.ws.WebSocketExchange;
import java.net.URI;
/**
*
* HTTP server module configuration.
*
*
* @author Jeremy Kuhn
* @since 1.0
*/
@Configuration( name = "configuration" )
public interface HttpServerConfiguration {
/**
*
* The host name of the server socket address.
*
*
*
* Defaults to {@code 0.0.0.0}.
*
*
* @return the server host name
*/
default String server_host() {
return "0.0.0.0";
}
/**
*
* The port of the server socket address.
*
*
*
* Defaults to {@code 8080}.
*
*
* @return the server port
*/
default int server_port() {
return 8080;
}
/**
*
* The number of event loops to allocate to the server.
*
*
*
* If not specified, the number of thread allocated to the root event loop group shall be used.
*
*
* @return the number of threads to allocate
*/
Integer server_event_loop_group_size();
/**
*
* Enables/Disables HTTP compression.
*
*
*
* Defaults to {@code false}.
*
*
* @return true if compression is enabled, false otherwise
*/
default boolean compression_enabled() {
return false;
}
/**
*
* Enables/Disables HTTP decompression.
*
*
*
* Defaults to {@code false}.
*
*
* @return true if decompression is enabled, false otherwise
*/
default boolean decompression_enabled() {
return false;
}
/**
*
* The threshold beyond which the response body should be compressed.
*
*
*
* Defaults to {@code 0} which means all responses are compressed.
*
*
* @return the compression content size threshold
*/
default int compression_contentSizeThreshold() {
return 0;
}
/**
*
* Brotly compression quality.
*
*
*
* Defaults to {@code 4}.
*
*
* @return the brotli compression quality
*/
default int compression_brotli_quality() {
return 4;
}
/**
*
* Brotly compression window.
*
*
*
* Defaults to {@code -1}.
*
*
* @return the brotli compression window
*/
default int compression_brotli_window() {
return -1;
}
/**
*
* Brotly compression mode (0=GENERIC, 1=TEXT, 2=FONT).
*
*
*
* Defaults to {@code 1} (TEXT).
*
*
* @return the brotli compression mode
*/
default int compression_brotli_mode() {
return 1;
}
/**
*
* Deflate compression level.
*
*
*
* Defaults to {@code 6}.
*
*
* @return the deflate compression level
*/
default int compression_deflate_compressionLevel() {
return 6;
}
/**
*
* Deflate compression window bits.
*
*
*
* Defaults to {@code 15}.
*
*
* @return the deflate compression window bits
*/
default int compression_deflate_windowBits() {
return 15;
}
/**
*
* Deflate compression memory level.
*
*
*
* Defaults to {@code 8}.
*
*
* @return the deflate compression memory level bits
*/
default int compression_deflate_memLevel() {
return 8;
}
/**
*
* Gzip compression level.
*
*
*
* Defaults to {@code 6}.
*
*
* @return the gzip compression level
*/
default int compression_gzip_compressionLevel() {
return 6;
}
/**
*
* Gzip compression window bits.
*
*
*
* Defaults to {@code 15}.
*
*
* @return the gzip compression window bits
*/
default int compression_gzip_windowBits() {
return 15;
}
/**
*
* Gzip compression memory level.
*
*
*
* Defaults to {@code 8}.
*
*
* @return the gzip compression memory level
*/
default int compression_gzip_memLevel() {
return 8;
}
/**
*
* Zstandard compression block size (in bytes).
*
*
*
* Defaults to 64KB.
*
*
* @return the zstd compression block size
*/
default int compression_zstd_blockSize() {
return 1 << 16;
}
/**
*
* Zstandard compression level.
*
*
*
* Defaults to {@code 3}.
*
*
* @return the zstd compression level
*/
default int compression_zstd_compressionLevel() {
return 3;
}
/**
*
* Zstandard compression max encode size (in bytes).
*
*
*
* Defaults to 32MB.
*
*
* @return the zstd compression max encode size
*/
default int compression_zstd_maxEncodeSize() {
return 1 << 10 + 0x0F;
}
/**
*
* The path to the key store.
*
*
* @return the key store URI
*/
URI key_store();
/**
*
* The type of key store.
*
*
*
* Defaults to {@code JKS}.
*
*
* @return the key store type
*/
default String key_store_type() {
return "JKS";
}
/**
*
* The password of the key store.
*
*
* @return a password
*/
String key_store_password();
/**
*
* Enables/Disables HTTPS.
*
*
*
* Defaults to {@code false}.
*
*
* @return true if the option is enabled, false otherwise
*/
default boolean tls_enabled() {
return false;
}
/**
*
* TLS handshake timeout (ms).
*
*
*
* Defaults to {@code 10000}.
*
*
* @return the TLS handshake timeout
*/
default long tls_handshake_timeout() {
return 10000;
}
/**
*
* The alias of the key in the key store.
*
*
* @return a key alias
*/
// String key_alias();
/**
*
* The list of ciphers to include.
*
*
* @return a list of ciphers
*/
String[] tls_ciphers_includes();
/**
*
* The list of ciphers to exclude.
*
*
* @return a list of ciphers
*/
String[] tls_ciphers_excludes();
/**
*
* Enables/Disables H2C.
*
*
*
* This only applies when SSL is disabled, otherwise {@link #h2_enabled()} is
* considered.
*
*
*
* Defaults to {@code false}.
*
*
* @return true if the option is enabled, false otherwise
*/
default boolean h2c_enabled() {
return false;
}
/**
*
* Enables/Disables HTTP/2.
*
*
*
* This only applies when SSL is enabled, otherwise {@link #h2c_enabled()} is
* considered.
*
*
*
* Defaults to {@code true}.
*
*
* @return true if the option is enabled, false otherwise
*/
default boolean h2_enabled() {
return true;
}
/**
*
* The HTTP/2 header table size.
*
*
*
* Defaults to {@code 4096}.
*
*
* @return the header table size
*/
default Long http2_header_table_size() {
return 4096l;
}
/**
*
* HTTP/2 max concurrent streams.
*
*
*
* Defaults to {@code 100}.
*
*
* @return max concurrent streams
*/
default Integer http2_max_concurrent_streams() {
return 100;
}
/**
*
* HTTP/2 initial window size.
*
*
*
* Defaults to {@code 65535}.
*
*
* @return initial window size
*/
default Integer http2_initial_window_size() {
return 65535;
}
/**
*
* HTTP/2 max frame size.
*
*
*
* Defaults to {@code 16384}.
*
*
* @return max frame size
*/
default Integer http2_max_frame_size() {
return 16384;
}
/**
*
* HTTP/2 max header list size.
*
*
*
* Defaults to {@link Integer#MAX_VALUE}.
*
*
* @return max header list size
*/
default Integer http2_max_header_list_size() {
return Integer.MAX_VALUE;
}
/**
*
* WebSocket handshake timeout (ms).
*
*
*
* Defaults to {@code -1} (i.e. no timeout).
*
*
* @return the WebSocket handshake timeout
*/
default long ws_handshake_timeout() {
return -1l;
}
/**
*
* WebSocket close timeout (ms).
*
*
*
* Defaults to {@code -1} (i.e. no timeout).
*
*
* @return the WebSocket close timeout
*/
default long ws_close_timeout() {
return -1l;
}
/**
*
* WebSocket max frame size in bytes.
*
*
*
* Defaults to {@code 65536}.
*
*
* @return the WebSocket max frame size
*/
default Integer ws_max_frame_size() {
return 65536;
}
/**
*
* Accepts/Rejects unproperly masked WebSocket frames.
*
*
*
* Defaults to {@code false}.
*
*
* @return true to allow unproperly masked frames, false otherwise
*/
default boolean ws_allow_mask_mismatch() {
return false;
}
/**
*
* Enables/Disables WebSocket per frame compression.
*
*
*
* Defaults to {@code false}.
*
*
* @return true if WebSocket per frame is enabled, false otherwise
*/
default boolean ws_frame_compression_enabled() {
return false;
}
/**
*
* WebSocket per frame compression level.
*
*
*
* Defaults to {@code 6}.
*
*
* @return the WebSocket frame compression level
*/
default int ws_frame_compression_level() {
return 6;
}
/**
*
* Enables/Disables WebSocket per message compression.
*
*
*
* Defaults to {@code false}.
*
*
* @return true if WebSocket per message is enabled, false otherwise
*/
default boolean ws_message_compression_enabled() {
return false;
}
/**
*
* WebSocket per message compression level.
*
*
*
* Defaults to {@code 6}.
*
*
* @return the WebSocket message compression level
*/
default int ws_message_compression_level() {
return 6;
}
/**
*
* Allows WebSocket client to customize the server inflater window size.
*
*
*
* Defaults to {@code false}.
*
*
* @return true to allow clients to customize the server inflater window size, false otherwise
*/
default boolean ws_message_allow_server_window_size() {
return false;
}
/**
*
* The prefered client window size to use if client inflater is customizable.
*
*
*
* Defaults to {@code 15}.
*
*
* @return the prefered client window size
*/
default int ws_message_prefered_client_window_size() {
return 15;
}
/**
*
* Allows WebSocket client to activate server_no_context_takeover.
*
*
*
* Defaults to {@code false}.
*
*
* @return true to allow clients to activate server_no_context_takeover, false otherwise
*/
default boolean ws_message_allow_server_no_context() {
return false;
}
/**
*
* Indicates if server prefers to activate client_no_context_takeover if client supports it.
*
*
*
* Defaults to {@code false}.
*
*
* @return true to activate client_no_context_takeover if client supports it, false otherwise
*/
default boolean ws_message_preferred_client_no_context() {
return false;
}
/**
*
* Indicates whether a WebSocket should be closed when the outbound frames publisher completes.
*
*
*
* Note that this behaviour can be overridden by invoking {@link WebSocketExchange.Outbound#closeOnComplete(boolean) } on the WebSocket exchange's outbound.
*
*
*
* Defaults to {@code false}
*
*
* @return true to close the WebSocket when the outbound frames publisher completes, false otherwise.
*/
default boolean ws_close_on_outbound_complete() {
return true;
}
/**
*
* The time in milliseconds to wait after a close frame has been sent for a close frame to be received before closing the WebSocket unilaterally.
*
*
*
* Defaults to {@code 60000}.
*
*
* @return the inbound close frame timeout
*/
default long ws_inbound_close_frame_timeout() {
return 60000l;
}
}