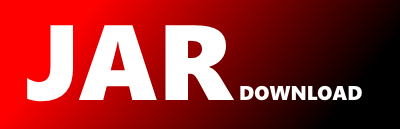
io.inverno.mod.http.server.Response Maven / Gradle / Ivy
/*
* Copyright 2020 Jeremy KUHN
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.inverno.mod.http.server;
import io.inverno.mod.http.base.BaseResponse;
import io.inverno.mod.http.base.OutboundHeaders;
import io.inverno.mod.http.base.OutboundResponseHeaders;
import io.inverno.mod.http.base.OutboundSetCookies;
import java.util.function.Consumer;
/**
*
* Represents a server response in a server exchange.
*
*
* @author Jeremy Kuhn
* @since 1.0
*
* @see Exchange
*/
public interface Response extends BaseResponse {
/**
*
* Determines whether the response headers have been sent to the client.
*
*
*
* Any attempts to specify new headers in the response, once headers haven been sent to the client, will result in an {@link IllegalStateException} being thrown.
*
*
* @return true if headers have been sent, false otherwise
*/
boolean isHeadersWritten();
/**
*
* Configures the HTTP headers to send in the response.
*
*
* @param headersConfigurer a response headers configurer
*
* @return the response
*
* @throws IllegalStateException if response headers have already been sent to the client
*/
Response headers(Consumer headersConfigurer) throws IllegalStateException;
/**
*
* Configures the HTTP trailers to send in the response.
*
*
* @param trailersConfigurer a response trailers configurer
*
* @return the response
*/
Response trailers(Consumer> trailersConfigurer);
/**
*
* Configures the cookies to set in the response headers.
*
*
* @param cookiesConfigurer a response cookies configurer
*
* @return the response
*
* @throws IllegalStateException if response headers have already been sent to the client
*
* @deprecated use {@link #headers(java.util.function.Consumer) } and {@link OutboundResponseHeaders#cookies(java.util.function.Consumer) }
*/
@Deprecated
Response cookies(Consumer cookiesConfigurer) throws IllegalStateException;
/**
*
* Sends an interim 100 continue response to the client so it can send the rest of the request.
*
*
*
* This method should only be used when the request contains header {@code expect: 100-continue}.
*
*
* @return the response
*/
Response sendContinue();
/**
*
* Returns the response body used to produce response payload.
*
*
* @return a response body
*/
ResponseBody body();
}