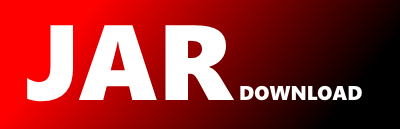
io.inverno.mod.security.http.AuthenticationErrorInterceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of inverno-security-http Show documentation
Show all versions of inverno-security-http Show documentation
Inverno security HTTP module
The newest version!
/*
* Copyright 2022 Jeremy Kuhn
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.inverno.mod.security.http;
import io.inverno.mod.http.base.ExchangeContext;
import io.inverno.mod.http.base.HttpException;
import io.inverno.mod.http.base.Status;
import io.inverno.mod.http.server.ErrorExchange;
import io.inverno.mod.http.server.ExchangeInterceptor;
import reactor.core.publisher.Mono;
/**
*
* Base implemenation for authentication error interceptors.
*
*
*
* An authentication error interceptor intercepts {@code UNAUTHORIZED(401)} errors in order to provide authentication instructions to the requester in the error response. Such interceptor is typically
* used to initiate the authentication process with the requester.
*
*
* @author Jeremy Kuhn
* @since 1.5
*
* @param the context type
* @param the error echange type
*/
public abstract class AuthenticationErrorInterceptor> implements ExchangeInterceptor {
/**
* Flag indicating whether the interceptor should claim the exchange (i.e. terminates the exchange by returning an empty response).
*/
protected final boolean terminal;
/**
*
* Creates a non-terminating authentication error interceptor.
*
*/
protected AuthenticationErrorInterceptor() {
this(false);
}
/**
*
* Creates an authentication error interceptor.
*
*
*
* If terminal, the interceptor claims the exchange and return an empty response resulting in no further processing.
*
*
* @param terminal true to terminate the exchange, false otherwise
*/
protected AuthenticationErrorInterceptor(boolean terminal) {
this.terminal = terminal;
}
@Override
public Mono extends B> intercept(B exchange) {
if(this.terminal) {
return Mono.fromRunnable(() -> {
Throwable error = exchange.getError();
if(error instanceof HttpException && ((HttpException)error).getStatusCode() == Status.UNAUTHORIZED.getCode()) {
this.interceptUnauthorized(exchange);
exchange.response().body().empty();
}
});
}
else {
return Mono.fromSupplier(() -> {
Throwable error = exchange.getError();
if(error instanceof HttpException && ((HttpException)error).getStatusCode() == Status.UNAUTHORIZED.getCode()) {
this.interceptUnauthorized(exchange);
}
return exchange;
});
}
}
/**
*
* Intercepts an unauthorized exchange.
*
*
* @param exchange the unauthorized exchange to intercept
*
* @throws HttpException if there was an error intercepting the exchange
*/
protected abstract void interceptUnauthorized(B exchange) throws HttpException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy