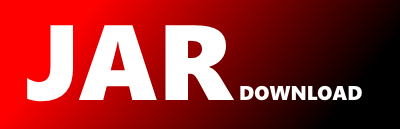
io.inversion.cosmosdb.CosmosDb Maven / Gradle / Ivy
/*
* Copyright (c) 2015-2020 Rocket Partners, LLC
* https://github.com/inversion-api
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.inversion.cosmosdb;
import com.microsoft.azure.documentdb.*;
import io.inversion.Index;
import io.inversion.*;
import io.inversion.json.JSMap;
import io.inversion.rql.Term;
import io.inversion.utils.Utils;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class CosmosDb extends Db {
public static final String INDEX_TYPE_PARTITION_KEY = "PartitionKey";
protected String uri = null;
protected String db = "";
protected String key = null;
transient protected DocumentClient documentClient = null;
boolean allowCrossPartitionQueries = false;
transient ConnectionPolicy connectionPolicy = null;
public CosmosDb() {
this.withType("cosmosdb");
}
public CosmosDb(String name) {
this();
withName(name);
}
public static DocumentClient buildDocumentClient(String uri, String key, ConnectionPolicy connectionPolicy) {
if (Utils.empty(uri) || Utils.empty(key)) {
String error = "";
error += "Unable to connect to Cosmos DB because conf values for 'uri' or 'key' can not be found. ";
error += "If this is a development environment, you should probably add these key/value pairs to a '.env' properties file in your working directory. ";
error += "If this is a production deployment, you should probably set these as environment variables on your container.";
error += "You could call CosmosDocumentDb.withUri() and CosmosDocumentDb.withKey() directly in your code but compiling these ";
error += "values into your code is strongly discouraged as a poor security practice.";
throw ApiException.new500InternalServerError(error);
}
connectionPolicy = ConnectionPolicy.GetDefault();
DocumentClient client = new DocumentClient(uri, key, connectionPolicy, ConsistencyLevel.Session);
return client;
}
/**
* Finds the resource keys on the other side of the relationship
*
* @param collection the collection to query
* @param columnMappedTerms the query terms
* @return Map key=sourceResourceKey, value=relatedResourceKey
* @throws ApiException if selection fails for any reason
*/
@Override
public Results doSelect(Collection collection, List columnMappedTerms) throws ApiException {
Index partitionIdx = collection.getIndexByType(INDEX_TYPE_PARTITION_KEY);
if (partitionIdx != null) {
Map values = new HashMap<>();
for (Property prop : partitionIdx.getProperties()) {
String colName = prop.getColumnName();
for (Term term : columnMappedTerms) {
if (term.hasChildLeafToken("eq") && colName.equals(term.getToken(0))) {
values.put(colName, term.getToken(1));
break;
}
}
}
for (Term term : columnMappedTerms) {
if (term.hasToken("_key")) {
String indexName = term.getToken(0);
Index idx = collection.getIndex(indexName);
Map key = collection.decodeKeyToColumnNames(idx, term.getToken(1));
// Rows.Row key = collection.decodeResourceKey(term.getToken(0));
for (Property prop : partitionIdx.getProperties()) {
String colName = prop.getColumnName();
if (key.containsKey(colName))
values.put(colName, key.get(colName));
}
}
}
//if the query supplied the parts necessary to construct the
if (values.size() == partitionIdx.size()) {
//-- remove any explicit partitionKey query params supplied by the users
columnMappedTerms.removeIf(term -> term.hasToken("eq") && partitionIdx.getName().equals(term.getToken(0)));
String partitionKey = io.inversion.Collection.encodeKey(values, partitionIdx, false);
columnMappedTerms.add(Term.term(null, "eq", partitionIdx.getName(), partitionKey));
}
}
CosmosSqlQuery query = new CosmosSqlQuery(this, collection, columnMappedTerms);
return query.doSelect();
}
@Override
public List doUpsert(Collection table, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy