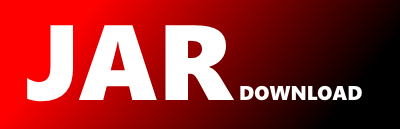
commonMain.io.islandtime._DateProperties.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core-metadata Show documentation
Show all versions of core-metadata Show documentation
A multiplatform library for working with dates and times
The newest version!
//
// This file is auto-generated by 'tools:code-generator'
//
@file:JvmMultifileClass
@file:JvmName("DateTimesKt")
package io.islandtime
import io.islandtime.calendar.WeekSettings
import io.islandtime.calendar.weekSettings
import io.islandtime.internal.lengthOfWeekBasedYear
import io.islandtime.internal.weekBasedYearImpl
import io.islandtime.internal.weekOfMonthImpl
import io.islandtime.internal.weekOfWeekBasedYearImpl
import io.islandtime.internal.weekOfYearImpl
import io.islandtime.locale.Locale
import io.islandtime.measures.IntDays
import io.islandtime.measures.IntWeeks
import io.islandtime.measures.days
import io.islandtime.ranges.DateRange
import io.islandtime.ranges.DateTimeInterval
import io.islandtime.ranges.OffsetDateTimeInterval
import io.islandtime.ranges.ZonedDateTimeInterval
import io.islandtime.ranges.until
import kotlin.Boolean
import kotlin.Int
import kotlin.jvm.JvmMultifileClass
import kotlin.jvm.JvmName
/**
* The range defining the ISO week that this date falls within.
*
* The ISO week starts on Monday and ends on Sunday.
*/
val Date.week: DateRange
get() = startOfWeek.let { it..it + 6.days }
/**
* The range defining the week that this date falls within. The first day of the week will be
* determined by the provided [settings].
*/
fun Date.week(settings: WeekSettings): DateRange = startOfWeek(settings).let { it..it + 6.days }
/**
* The range defining the week that this date falls within. The first day of the week will be the
* default associated with the provided [locale].
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*/
fun Date.week(locale: Locale): DateRange = startOfWeek(locale).let { it..it + 6.days }
/**
* The week of the month, from 0-6, calculated using the ISO week definition.
*/
val Date.weekOfMonth: Int
get() = weekOfMonthImpl(WeekSettings.ISO)
/**
* The week of the month, from 0-6, calculated using the week definition in [settings].
*/
fun Date.weekOfMonth(settings: WeekSettings): Int = weekOfMonthImpl(settings)
/**
* The week of the month, from 0-6, calculated using the default week definition associated with the
* provided [locale].
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*/
fun Date.weekOfMonth(locale: Locale): Int = weekOfMonthImpl(locale.weekSettings)
/**
* The week of the year, calculated using the ISO week definition. If the week number is associated
* with the preceding year, `0` will be returned.
*
* To obtain the week number used in the ISO week date system, use [weekOfWeekBasedYear] instead.
*
* @see weekOfWeekBasedYear
*/
val Date.weekOfYear: Int
get() = weekOfYearImpl(WeekSettings.ISO)
/**
* The week of the year, calculated using the week definition in [settings]. If the week number is
* associated with the preceding year, `0` will be returned.
*
* To obtain the week number of the week-based year, use [weekOfWeekBasedYear] instead.
*
* @see weekOfWeekBasedYear
*/
fun Date.weekOfYear(settings: WeekSettings): Int = weekOfYearImpl(settings)
/**
* The week of the year, calculated using the week definition associated with the provided [locale].
* If the week number is associated with the preceding year, `0` will be returned.
*
* To obtain the week number of the week-based year, use [weekOfWeekBasedYear] instead.
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*
* @see weekOfWeekBasedYear
*/
fun Date.weekOfYear(locale: Locale): Int = weekOfYearImpl(locale.weekSettings)
/**
* The week-based year used in the ISO
* [week date system](https://en.wikipedia.org/wiki/ISO_week_date). This value differs from the regular
* ISO year when the week number falls in the preceding or following year.
*
* @see weekOfWeekBasedYear
*/
val Date.weekBasedYear: Int
get() = weekBasedYearImpl(WeekSettings.ISO)
/**
* The week-based year, calculated using the week definition in [settings]. This value differs from
* the regular ISO year when the week number falls in the preceding or following year.
*
* @see weekOfWeekBasedYear
*/
fun Date.weekBasedYear(settings: WeekSettings): Int = weekBasedYearImpl(settings)
/**
* The week-based year, calculated using the week definition associated with the provided [locale].
* This value differs from the regular ISO year when the week number falls in the preceding or
* following year.
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*
* @see weekOfWeekBasedYear
*/
fun Date.weekBasedYear(locale: Locale): Int = weekBasedYearImpl(locale.weekSettings)
/**
* The week number used in the ISO [week date system](https://en.wikipedia.org/wiki/ISO_week_date).
*
* @see weekBasedYear
*/
val Date.weekOfWeekBasedYear: Int
get() = weekOfWeekBasedYearImpl(WeekSettings.ISO)
/**
* The week number of the week-based year, calculated using the week definition in [settings].
*
* @see weekBasedYear
*/
fun Date.weekOfWeekBasedYear(settings: WeekSettings): Int = weekOfWeekBasedYearImpl(settings)
/**
* The week number of the week-based year, calculated using the week definition associated with the
* provided [locale].
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*
* @see weekBasedYear
*/
fun Date.weekOfWeekBasedYear(locale: Locale): Int = weekOfWeekBasedYearImpl(locale.weekSettings)
/**
* Checks if this date falls within a leap year.
*/
val Date.isInLeapYear: Boolean
get() = isLeapYear(year)
/**
* Checks if this date is February 29.
*/
val Date.isLeapDay: Boolean
get() = month == Month.FEBRUARY && dayOfMonth == 29
/**
* The length of this date's month in days.
*/
val Date.lengthOfMonth: IntDays
get() = month.lengthIn(year)
/**
* The length of this date's year in days.
*/
val Date.lengthOfYear: IntDays
get() = lengthOfYear(year)
/**
* The length of the ISO week-based year that this date falls in, either 52 or 53 weeks.
*/
val Date.lengthOfWeekBasedYear: IntWeeks
get() = lengthOfWeekBasedYear(weekBasedYear)
/**
* The next date after this one that falls on [dayOfWeek].
*/
fun Date.next(dayOfWeek: DayOfWeek): Date {
val dayDiff = this.dayOfWeek.ordinal - dayOfWeek.ordinal
return if (dayDiff >= 0) {
this + (7 - dayDiff).days
} else {
this + (-dayDiff).days
}
}
/**
* The next date that falls on [dayOfWeek], or this date if it falls on the same day.
*/
fun Date.nextOrSame(dayOfWeek: DayOfWeek): Date = if (dayOfWeek == this.dayOfWeek) this else
next(dayOfWeek)
/**
* The last date before this one that falls on [dayOfWeek].
*/
fun Date.previous(dayOfWeek: DayOfWeek): Date {
val dayDiff = dayOfWeek.ordinal - this.dayOfWeek.ordinal
return if (dayDiff >= 0) {
this - (7 - dayDiff).days
} else {
this - (-dayDiff).days
}
}
/**
* The previous date that falls on [dayOfWeek], or this date if it falls on the same day.
*/
fun Date.previousOrSame(dayOfWeek: DayOfWeek): Date = if (dayOfWeek == this.dayOfWeek) this else
previous(dayOfWeek)
/**
* The interval defining the ISO week that this date-time falls within.
*
* The ISO week starts on Monday and ends on Sunday.
*/
val DateTime.week: DateTimeInterval
get() = startOfWeek.let { it until it + 7.days }
/**
* The interval defining the week that this date-time falls within. The first day of the week will
* be determined by the provided [settings].
*/
fun DateTime.week(settings: WeekSettings): DateTimeInterval =
startOfWeek(settings).let { it until it + 7.days }
/**
* The interval defining the week that this date-time falls within. The first day of the week will
* be the default associated with the provided [locale].
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*/
fun DateTime.week(locale: Locale): DateTimeInterval =
startOfWeek(locale).let { it until it + 7.days }
/**
* The week of the month, from 0-6, calculated using the ISO week definition.
*/
val DateTime.weekOfMonth: Int
inline get() = date.weekOfMonth
/**
* The week of the month, from 0-6, calculated using the week definition in [settings].
*/
fun DateTime.weekOfMonth(settings: WeekSettings): Int = date.weekOfMonth(settings)
/**
* The week of the month, from 0-6, calculated using the default week definition associated with the
* provided [locale].
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*/
fun DateTime.weekOfMonth(locale: Locale): Int = date.weekOfMonth(locale)
/**
* The week of the year, calculated using the ISO week definition. If the week number is associated
* with the preceding year, `0` will be returned.
*
* To obtain the week number used in the ISO week date system, use [weekOfWeekBasedYear] instead.
*
* @see weekOfWeekBasedYear
*/
val DateTime.weekOfYear: Int
inline get() = date.weekOfYear
/**
* The week of the year, calculated using the week definition in [settings]. If the week number is
* associated with the preceding year, `0` will be returned.
*
* To obtain the week number of the week-based year, use [weekOfWeekBasedYear] instead.
*
* @see weekOfWeekBasedYear
*/
fun DateTime.weekOfYear(settings: WeekSettings): Int = date.weekOfYear(settings)
/**
* The week of the year, calculated using the week definition associated with the provided [locale].
* If the week number is associated with the preceding year, `0` will be returned.
*
* To obtain the week number of the week-based year, use [weekOfWeekBasedYear] instead.
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*
* @see weekOfWeekBasedYear
*/
fun DateTime.weekOfYear(locale: Locale): Int = date.weekOfYear(locale)
/**
* The week-based year used in the ISO
* [week date system](https://en.wikipedia.org/wiki/ISO_week_date). This value differs from the regular
* ISO year when the week number falls in the preceding or following year.
*
* @see weekOfWeekBasedYear
*/
val DateTime.weekBasedYear: Int
inline get() = date.weekBasedYear
/**
* The week-based year, calculated using the week definition in [settings]. This value differs from
* the regular ISO year when the week number falls in the preceding or following year.
*
* @see weekOfWeekBasedYear
*/
fun DateTime.weekBasedYear(settings: WeekSettings): Int = date.weekBasedYear(settings)
/**
* The week-based year, calculated using the week definition associated with the provided [locale].
* This value differs from the regular ISO year when the week number falls in the preceding or
* following year.
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*
* @see weekOfWeekBasedYear
*/
fun DateTime.weekBasedYear(locale: Locale): Int = date.weekBasedYear(locale)
/**
* The week number used in the ISO [week date system](https://en.wikipedia.org/wiki/ISO_week_date).
*
* @see weekBasedYear
*/
val DateTime.weekOfWeekBasedYear: Int
inline get() = date.weekOfWeekBasedYear
/**
* The week number of the week-based year, calculated using the week definition in [settings].
*
* @see weekBasedYear
*/
fun DateTime.weekOfWeekBasedYear(settings: WeekSettings): Int = date.weekOfWeekBasedYear(settings)
/**
* The week number of the week-based year, calculated using the week definition associated with the
* provided [locale].
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*
* @see weekBasedYear
*/
fun DateTime.weekOfWeekBasedYear(locale: Locale): Int = date.weekOfWeekBasedYear(locale)
/**
* Checks if this date-time falls within a leap year.
*/
val DateTime.isInLeapYear: Boolean
inline get() = date.isInLeapYear
/**
* Checks if this date-time falls within February 29.
*/
val DateTime.isInLeapDay: Boolean
inline get() = date.isLeapDay
/**
* The length of this date-time's month in days.
*/
val DateTime.lengthOfMonth: IntDays
inline get() = date.lengthOfMonth
/**
* The length of this date-time's year in days.
*/
val DateTime.lengthOfYear: IntDays
inline get() = date.lengthOfYear
/**
* The length of the ISO week-based year that this date-time falls in, either 52 or 53 weeks.
*/
val DateTime.lengthOfWeekBasedYear: IntWeeks
inline get() = date.lengthOfWeekBasedYear
/**
* The next date-time after this one that falls on [dayOfWeek].
*/
fun DateTime.next(dayOfWeek: DayOfWeek): DateTime = copy(date = date.next(dayOfWeek))
/**
* The next date-time that falls on [dayOfWeek], or this date-time if it falls on the same day.
*/
fun DateTime.nextOrSame(dayOfWeek: DayOfWeek): DateTime = if (dayOfWeek == this.dayOfWeek) this else
next(dayOfWeek)
/**
* The last date-time before this one that falls on [dayOfWeek].
*/
fun DateTime.previous(dayOfWeek: DayOfWeek): DateTime = copy(date = date.previous(dayOfWeek))
/**
* The previous date-time that falls on [dayOfWeek], or this date-time if it falls on the same day.
*/
fun DateTime.previousOrSame(dayOfWeek: DayOfWeek): DateTime = if (dayOfWeek == this.dayOfWeek) this
else previous(dayOfWeek)
/**
* The interval defining the ISO week that this date-time falls within.
*
* The ISO week starts on Monday and ends on Sunday.
*/
val OffsetDateTime.week: OffsetDateTimeInterval
get() = startOfWeek.let { it until it + 7.days }
/**
* The interval defining the week that this date-time falls within. The first day of the week will
* be determined by the provided [settings].
*/
fun OffsetDateTime.week(settings: WeekSettings): OffsetDateTimeInterval =
startOfWeek(settings).let { it until it + 7.days }
/**
* The interval defining the week that this date-time falls within. The first day of the week will
* be the default associated with the provided [locale].
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*/
fun OffsetDateTime.week(locale: Locale): OffsetDateTimeInterval =
startOfWeek(locale).let { it until it + 7.days }
/**
* The week of the month, from 0-6, calculated using the ISO week definition.
*/
val OffsetDateTime.weekOfMonth: Int
inline get() = dateTime.weekOfMonth
/**
* The week of the month, from 0-6, calculated using the week definition in [settings].
*/
fun OffsetDateTime.weekOfMonth(settings: WeekSettings): Int = dateTime.weekOfMonth(settings)
/**
* The week of the month, from 0-6, calculated using the default week definition associated with the
* provided [locale].
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*/
fun OffsetDateTime.weekOfMonth(locale: Locale): Int = dateTime.weekOfMonth(locale)
/**
* The week of the year, calculated using the ISO week definition. If the week number is associated
* with the preceding year, `0` will be returned.
*
* To obtain the week number used in the ISO week date system, use [weekOfWeekBasedYear] instead.
*
* @see weekOfWeekBasedYear
*/
val OffsetDateTime.weekOfYear: Int
inline get() = dateTime.weekOfYear
/**
* The week of the year, calculated using the week definition in [settings]. If the week number is
* associated with the preceding year, `0` will be returned.
*
* To obtain the week number of the week-based year, use [weekOfWeekBasedYear] instead.
*
* @see weekOfWeekBasedYear
*/
fun OffsetDateTime.weekOfYear(settings: WeekSettings): Int = dateTime.weekOfYear(settings)
/**
* The week of the year, calculated using the week definition associated with the provided [locale].
* If the week number is associated with the preceding year, `0` will be returned.
*
* To obtain the week number of the week-based year, use [weekOfWeekBasedYear] instead.
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*
* @see weekOfWeekBasedYear
*/
fun OffsetDateTime.weekOfYear(locale: Locale): Int = dateTime.weekOfYear(locale)
/**
* The week-based year used in the ISO
* [week date system](https://en.wikipedia.org/wiki/ISO_week_date). This value differs from the regular
* ISO year when the week number falls in the preceding or following year.
*
* @see weekOfWeekBasedYear
*/
val OffsetDateTime.weekBasedYear: Int
inline get() = dateTime.weekBasedYear
/**
* The week-based year, calculated using the week definition in [settings]. This value differs from
* the regular ISO year when the week number falls in the preceding or following year.
*
* @see weekOfWeekBasedYear
*/
fun OffsetDateTime.weekBasedYear(settings: WeekSettings): Int = dateTime.weekBasedYear(settings)
/**
* The week-based year, calculated using the week definition associated with the provided [locale].
* This value differs from the regular ISO year when the week number falls in the preceding or
* following year.
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*
* @see weekOfWeekBasedYear
*/
fun OffsetDateTime.weekBasedYear(locale: Locale): Int = dateTime.weekBasedYear(locale)
/**
* The week number used in the ISO [week date system](https://en.wikipedia.org/wiki/ISO_week_date).
*
* @see weekBasedYear
*/
val OffsetDateTime.weekOfWeekBasedYear: Int
inline get() = dateTime.weekOfWeekBasedYear
/**
* The week number of the week-based year, calculated using the week definition in [settings].
*
* @see weekBasedYear
*/
fun OffsetDateTime.weekOfWeekBasedYear(settings: WeekSettings): Int =
dateTime.weekOfWeekBasedYear(settings)
/**
* The week number of the week-based year, calculated using the week definition associated with the
* provided [locale].
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*
* @see weekBasedYear
*/
fun OffsetDateTime.weekOfWeekBasedYear(locale: Locale): Int = dateTime.weekOfWeekBasedYear(locale)
/**
* Checks if this date-time falls within a leap year.
*/
val OffsetDateTime.isInLeapYear: Boolean
inline get() = dateTime.isInLeapYear
/**
* Checks if this date-time falls within February 29.
*/
val OffsetDateTime.isInLeapDay: Boolean
inline get() = date.isLeapDay
/**
* The length of this date-time's month in days.
*/
val OffsetDateTime.lengthOfMonth: IntDays
inline get() = dateTime.lengthOfMonth
/**
* The length of this date-time's year in days.
*/
val OffsetDateTime.lengthOfYear: IntDays
inline get() = dateTime.lengthOfYear
/**
* The length of the ISO week-based year that this date-time falls in, either 52 or 53 weeks.
*/
val OffsetDateTime.lengthOfWeekBasedYear: IntWeeks
inline get() = dateTime.lengthOfWeekBasedYear
/**
* The next date-time after this one that falls on [dayOfWeek].
*/
fun OffsetDateTime.next(dayOfWeek: DayOfWeek): OffsetDateTime = copy(dateTime =
dateTime.next(dayOfWeek))
/**
* The next date-time that falls on [dayOfWeek], or this date-time if it falls on the same day.
*/
fun OffsetDateTime.nextOrSame(dayOfWeek: DayOfWeek): OffsetDateTime = if (dayOfWeek ==
this.dayOfWeek) this else next(dayOfWeek)
/**
* The last date-time before this one that falls on [dayOfWeek].
*/
fun OffsetDateTime.previous(dayOfWeek: DayOfWeek): OffsetDateTime = copy(dateTime =
dateTime.previous(dayOfWeek))
/**
* The previous date-time that falls on [dayOfWeek], or this date-time if it falls on the same day.
*/
fun OffsetDateTime.previousOrSame(dayOfWeek: DayOfWeek): OffsetDateTime = if (dayOfWeek ==
this.dayOfWeek) this else previous(dayOfWeek)
/**
* The interval defining the ISO week that this date-time falls within.
*
* The ISO week starts on Monday and ends on Sunday.
*/
val ZonedDateTime.week: ZonedDateTimeInterval
get() = startOfWeek.let { it until it + 7.days }
/**
* The interval defining the week that this date-time falls within. The first day of the week will
* be determined by the provided [settings].
*/
fun ZonedDateTime.week(settings: WeekSettings): ZonedDateTimeInterval =
startOfWeek(settings).let { it until it + 7.days }
/**
* The interval defining the week that this date-time falls within. The first day of the week will
* be the default associated with the provided [locale].
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*/
fun ZonedDateTime.week(locale: Locale): ZonedDateTimeInterval =
startOfWeek(locale).let { it until it + 7.days }
/**
* The week of the month, from 0-6, calculated using the ISO week definition.
*/
val ZonedDateTime.weekOfMonth: Int
inline get() = dateTime.weekOfMonth
/**
* The week of the month, from 0-6, calculated using the week definition in [settings].
*/
fun ZonedDateTime.weekOfMonth(settings: WeekSettings): Int = dateTime.weekOfMonth(settings)
/**
* The week of the month, from 0-6, calculated using the default week definition associated with the
* provided [locale].
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*/
fun ZonedDateTime.weekOfMonth(locale: Locale): Int = dateTime.weekOfMonth(locale)
/**
* The week of the year, calculated using the ISO week definition. If the week number is associated
* with the preceding year, `0` will be returned.
*
* To obtain the week number used in the ISO week date system, use [weekOfWeekBasedYear] instead.
*
* @see weekOfWeekBasedYear
*/
val ZonedDateTime.weekOfYear: Int
inline get() = dateTime.weekOfYear
/**
* The week of the year, calculated using the week definition in [settings]. If the week number is
* associated with the preceding year, `0` will be returned.
*
* To obtain the week number of the week-based year, use [weekOfWeekBasedYear] instead.
*
* @see weekOfWeekBasedYear
*/
fun ZonedDateTime.weekOfYear(settings: WeekSettings): Int = dateTime.weekOfYear(settings)
/**
* The week of the year, calculated using the week definition associated with the provided [locale].
* If the week number is associated with the preceding year, `0` will be returned.
*
* To obtain the week number of the week-based year, use [weekOfWeekBasedYear] instead.
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*
* @see weekOfWeekBasedYear
*/
fun ZonedDateTime.weekOfYear(locale: Locale): Int = dateTime.weekOfYear(locale)
/**
* The week-based year used in the ISO
* [week date system](https://en.wikipedia.org/wiki/ISO_week_date). This value differs from the regular
* ISO year when the week number falls in the preceding or following year.
*
* @see weekOfWeekBasedYear
*/
val ZonedDateTime.weekBasedYear: Int
inline get() = dateTime.weekBasedYear
/**
* The week-based year, calculated using the week definition in [settings]. This value differs from
* the regular ISO year when the week number falls in the preceding or following year.
*
* @see weekOfWeekBasedYear
*/
fun ZonedDateTime.weekBasedYear(settings: WeekSettings): Int = dateTime.weekBasedYear(settings)
/**
* The week-based year, calculated using the week definition associated with the provided [locale].
* This value differs from the regular ISO year when the week number falls in the preceding or
* following year.
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*
* @see weekOfWeekBasedYear
*/
fun ZonedDateTime.weekBasedYear(locale: Locale): Int = dateTime.weekBasedYear(locale)
/**
* The week number used in the ISO [week date system](https://en.wikipedia.org/wiki/ISO_week_date).
*
* @see weekBasedYear
*/
val ZonedDateTime.weekOfWeekBasedYear: Int
inline get() = dateTime.weekOfWeekBasedYear
/**
* The week number of the week-based year, calculated using the week definition in [settings].
*
* @see weekBasedYear
*/
fun ZonedDateTime.weekOfWeekBasedYear(settings: WeekSettings): Int =
dateTime.weekOfWeekBasedYear(settings)
/**
* The week number of the week-based year, calculated using the week definition associated with the
* provided [locale].
*
* Keep in mind that that the system's calendar settings may differ from that of the default locale
* on some platforms. To respect the system calendar settings, use [WeekSettings.systemDefault]
* instead.
*
* @see weekBasedYear
*/
fun ZonedDateTime.weekOfWeekBasedYear(locale: Locale): Int = dateTime.weekOfWeekBasedYear(locale)
/**
* Checks if this date-time falls within a leap year.
*/
val ZonedDateTime.isInLeapYear: Boolean
inline get() = dateTime.isInLeapYear
/**
* Checks if this date-time falls within February 29.
*/
val ZonedDateTime.isInLeapDay: Boolean
inline get() = date.isLeapDay
/**
* The length of this date-time's month in days.
*/
val ZonedDateTime.lengthOfMonth: IntDays
inline get() = dateTime.lengthOfMonth
/**
* The length of this date-time's year in days.
*/
val ZonedDateTime.lengthOfYear: IntDays
inline get() = dateTime.lengthOfYear
/**
* The length of the ISO week-based year that this date-time falls in, either 52 or 53 weeks.
*/
val ZonedDateTime.lengthOfWeekBasedYear: IntWeeks
inline get() = dateTime.lengthOfWeekBasedYear
/**
* The next date-time after this one that falls on [dayOfWeek].
*/
fun ZonedDateTime.next(dayOfWeek: DayOfWeek): ZonedDateTime = copy(dateTime =
dateTime.next(dayOfWeek))
/**
* The next date-time that falls on [dayOfWeek], or this date-time if it falls on the same day.
*/
fun ZonedDateTime.nextOrSame(dayOfWeek: DayOfWeek): ZonedDateTime = if (dayOfWeek == this.dayOfWeek)
this else next(dayOfWeek)
/**
* The last date-time before this one that falls on [dayOfWeek].
*/
fun ZonedDateTime.previous(dayOfWeek: DayOfWeek): ZonedDateTime = copy(dateTime =
dateTime.previous(dayOfWeek))
/**
* The previous date-time that falls on [dayOfWeek], or this date-time if it falls on the same day.
*/
fun ZonedDateTime.previousOrSame(dayOfWeek: DayOfWeek): ZonedDateTime = if (dayOfWeek ==
this.dayOfWeek) this else previous(dayOfWeek)
© 2015 - 2025 Weber Informatics LLC | Privacy Policy