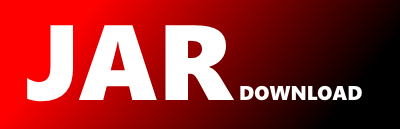
commonMain.io.islandtime.measures._Years.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core-metadata Show documentation
Show all versions of core-metadata Show documentation
A multiplatform library for working with dates and times
The newest version!
//
// This file is auto-generated by 'tools:code-generator'
//
@file:JvmMultifileClass
@file:JvmName("YearsKt")
package io.islandtime.measures
import dev.erikchristensen.javamath2kmp.minusExact
import dev.erikchristensen.javamath2kmp.negateExact
import dev.erikchristensen.javamath2kmp.plusExact
import dev.erikchristensen.javamath2kmp.timesExact
import dev.erikchristensen.javamath2kmp.toIntExact
import io.islandtime.internal.MONTHS_PER_YEAR
import io.islandtime.internal.YEARS_PER_CENTURY
import io.islandtime.internal.YEARS_PER_DECADE
import kotlin.Boolean
import kotlin.Comparable
import kotlin.Int
import kotlin.Long
import kotlin.PublishedApi
import kotlin.String
import kotlin.jvm.JvmMultifileClass
import kotlin.jvm.JvmName
import kotlin.math.absoluteValue
/**
* A number of years.
*/
inline class IntYears(
/**
* The underlying value.
*/
val value: Int
) : Comparable {
/**
* The absolute value of this duration.
* @throws ArithmeticException if overflow occurs
*/
val absoluteValue: IntYears
get() = if (value < 0) -this else this
/**
* Converts this duration to months.
* @throws ArithmeticException if overflow occurs
*/
val inMonths: IntMonths
get() = (value timesExact MONTHS_PER_YEAR).months
/**
* Converts this duration to months without checking for overflow.
*/
internal val inMonthsUnchecked: IntMonths
get() = (value * MONTHS_PER_YEAR).months
/**
* Converts this duration to the number of whole decades.
*/
val inDecades: IntDecades
get() = (value / YEARS_PER_DECADE).decades
/**
* Converts this duration to the number of whole centuries.
*/
val inCenturies: IntCenturies
get() = (value / YEARS_PER_CENTURY).centuries
/**
* Checks if this duration is zero.
*/
fun isZero(): Boolean = value == 0
/**
* Checks if this duration is negative.
*/
fun isNegative(): Boolean = value < 0
/**
* Checks if this duration is positive.
*/
fun isPositive(): Boolean = value > 0
override fun compareTo(other: IntYears): Int = value.compareTo(other.value)
/**
* Converts this duration to an ISO-8601 time interval representation.
*/
override fun toString(): String {
return when (value) {
0 -> "P0Y"
Int.MIN_VALUE -> "-P2147483648Y"
else -> buildString {
if (value < 0) { append('-') }
append("P")
append(value.absoluteValue)
append('Y')
}
}
}
/**
* Negates this duration.
* @throws ArithmeticException if overflow occurs
*/
operator fun unaryMinus() = IntYears(value.negateExact())
/**
* Negates this duration without checking for overflow.
*/
internal fun negateUnchecked() = IntYears(-value)
/**
* Multiplies this duration by a scalar value.
* @throws ArithmeticException if overflow occurs
*/
operator fun times(scalar: Int) = IntYears(value timesExact scalar)
/**
* Multiplies this duration by a scalar value.
* @throws ArithmeticException if overflow occurs
*/
operator fun times(scalar: Long) = this.toLongYears() * scalar
/**
* Divides this duration by a scalar value.
* @throws ArithmeticException if overflow occurs or the scalar is zero
*/
operator fun div(scalar: Int): IntYears {
return if (scalar == -1) {
-this
} else {
IntYears(value / scalar)
}
}
/**
* Divides this duration by a scalar value.
* @throws ArithmeticException if the scalar is zero
*/
operator fun div(scalar: Long): LongYears = this.toLongYears() / scalar
operator fun rem(scalar: Int) = IntYears(value % scalar)
operator fun rem(scalar: Long) = this.toLongYears() % scalar
operator fun plus(months: IntMonths) = this.inMonths + months
operator fun minus(months: IntMonths) = this.inMonths - months
operator fun plus(months: LongMonths) = this.toLongYears().inMonths + months
operator fun minus(months: LongMonths) = this.toLongYears().inMonths - months
operator fun plus(years: IntYears) = IntYears(value plusExact years.value)
operator fun minus(years: IntYears) = IntYears(value minusExact years.value)
operator fun plus(years: LongYears) = LongYears(value.toLong() plusExact years.value)
operator fun minus(years: LongYears) = LongYears(value.toLong() minusExact years.value)
operator fun plus(decades: IntDecades) = this + decades.inYears
operator fun minus(decades: IntDecades) = this - decades.inYears
operator fun plus(decades: LongDecades) = this.toLongYears() + decades.inYears
operator fun minus(decades: LongDecades) = this.toLongYears() - decades.inYears
operator fun plus(centuries: IntCenturies) = this + centuries.inYears
operator fun minus(centuries: IntCenturies) = this - centuries.inYears
operator fun plus(centuries: LongCenturies) = this.toLongYears() + centuries.inYears
operator fun minus(centuries: LongCenturies) = this.toLongYears() - centuries.inYears
inline fun toComponents(action: (decades: IntDecades, years: IntYears) -> T): T {
val decades = (value / YEARS_PER_DECADE).decades
val years = (value % YEARS_PER_DECADE).years
return action(decades, years)
}
inline fun toComponents(action: (
centuries: IntCenturies,
decades: IntDecades,
years: IntYears
) -> T): T {
val centuries = (value / YEARS_PER_CENTURY).centuries
val decades = ((value % YEARS_PER_CENTURY) / YEARS_PER_DECADE).decades
val years = (value % YEARS_PER_DECADE).years
return action(centuries, decades, years)
}
/**
* Converts this duration to [LongYears].
*/
fun toLongYears() = LongYears(value.toLong())
/**
* Converts this duration to a `Long` value.
*/
fun toLong() = value.toLong()
companion object {
/**
* The smallest supported value.
*/
val MIN: IntYears = IntYears(Int.MIN_VALUE)
/**
* The largest supported value.
*/
val MAX: IntYears = IntYears(Int.MAX_VALUE)
}
}
/**
* Converts this value to a duration of years.
*/
val Int.years: IntYears
get() = IntYears(this)
/**
* Multiplies this value by a duration of years.
* @throws ArithmeticException if overflow occurs
*/
operator fun Int.times(years: IntYears) = years * this
/**
* Multiplies this value by a duration of years.
* @throws ArithmeticException if overflow occurs
*/
operator fun Long.times(years: IntYears) = years * this
/**
* A number of years.
*/
inline class LongYears(
/**
* The underlying value.
*/
val value: Long
) : Comparable {
/**
* The absolute value of this duration.
* @throws ArithmeticException if overflow occurs
*/
val absoluteValue: LongYears
get() = if (value < 0) -this else this
/**
* Converts this duration to months.
* @throws ArithmeticException if overflow occurs
*/
val inMonths: LongMonths
get() = (value timesExact MONTHS_PER_YEAR).months
/**
* Converts this duration to months without checking for overflow.
*/
internal val inMonthsUnchecked: LongMonths
get() = (value * MONTHS_PER_YEAR).months
/**
* Converts this duration to the number of whole decades.
*/
val inDecades: LongDecades
get() = (value / YEARS_PER_DECADE).decades
/**
* Converts this duration to the number of whole centuries.
*/
val inCenturies: LongCenturies
get() = (value / YEARS_PER_CENTURY).centuries
/**
* Checks if this duration is zero.
*/
fun isZero(): Boolean = value == 0L
/**
* Checks if this duration is negative.
*/
fun isNegative(): Boolean = value < 0L
/**
* Checks if this duration is positive.
*/
fun isPositive(): Boolean = value > 0L
override fun compareTo(other: LongYears): Int = value.compareTo(other.value)
/**
* Converts this duration to an ISO-8601 time interval representation.
*/
override fun toString(): String {
return when (value) {
0L -> "P0Y"
Long.MIN_VALUE -> "-P9223372036854775808Y"
else -> buildString {
if (value < 0) { append('-') }
append("P")
append(value.absoluteValue)
append('Y')
}
}
}
/**
* Negates this duration.
* @throws ArithmeticException if overflow occurs
*/
operator fun unaryMinus() = LongYears(value.negateExact())
/**
* Negates this duration without checking for overflow.
*/
internal fun negateUnchecked() = LongYears(-value)
/**
* Multiplies this duration by a scalar value.
* @throws ArithmeticException if overflow occurs
*/
operator fun times(scalar: Int) = LongYears(value timesExact scalar)
/**
* Multiplies this duration by a scalar value.
* @throws ArithmeticException if overflow occurs
*/
operator fun times(scalar: Long) = LongYears(value timesExact scalar)
/**
* Divides this duration by a scalar value.
* @throws ArithmeticException if overflow occurs or the scalar is zero
*/
operator fun div(scalar: Int): LongYears {
return if (scalar == -1) {
-this
} else {
LongYears(value / scalar)
}
}
/**
* Divides this duration by a scalar value.
* @throws ArithmeticException if overflow occurs or the scalar is zero
*/
operator fun div(scalar: Long): LongYears {
return if (scalar == -1L) {
-this
} else {
LongYears(value / scalar)
}
}
operator fun rem(scalar: Int) = LongYears(value % scalar)
operator fun rem(scalar: Long) = LongYears(value % scalar)
operator fun plus(months: IntMonths) = this.inMonths + months
operator fun minus(months: IntMonths) = this.inMonths - months
operator fun plus(months: LongMonths) = this.inMonths + months
operator fun minus(months: LongMonths) = this.inMonths - months
operator fun plus(years: IntYears) = LongYears(value plusExact years.value)
operator fun minus(years: IntYears) = LongYears(value minusExact years.value)
operator fun plus(years: LongYears) = LongYears(value plusExact years.value)
operator fun minus(years: LongYears) = LongYears(value minusExact years.value)
operator fun plus(decades: IntDecades) = this + decades.inYears
operator fun minus(decades: IntDecades) = this - decades.inYears
operator fun plus(decades: LongDecades) = this + decades.inYears
operator fun minus(decades: LongDecades) = this - decades.inYears
operator fun plus(centuries: IntCenturies) = this + centuries.inYears
operator fun minus(centuries: IntCenturies) = this - centuries.inYears
operator fun plus(centuries: LongCenturies) = this + centuries.inYears
operator fun minus(centuries: LongCenturies) = this - centuries.inYears
inline fun toComponents(action: (decades: LongDecades, years: IntYears) -> T): T {
val decades = (value / YEARS_PER_DECADE).decades
val years = (value % YEARS_PER_DECADE).toInt().years
return action(decades, years)
}
inline fun toComponents(action: (
centuries: LongCenturies,
decades: IntDecades,
years: IntYears
) -> T): T {
val centuries = (value / YEARS_PER_CENTURY).centuries
val decades = ((value % YEARS_PER_CENTURY) / YEARS_PER_DECADE).toInt().decades
val years = (value % YEARS_PER_DECADE).toInt().years
return action(centuries, decades, years)
}
/**
* Converts this duration to [IntYears].
* @throws ArithmeticException if overflow occurs
*/
fun toIntYears() = IntYears(value.toIntExact())
/**
* Converts this duration to [IntYears] without checking for overflow.
*/
@PublishedApi
internal fun toIntYearsUnchecked() = IntYears(value.toInt())
/**
* Converts this duration to an `Int` value.
* @throws ArithmeticException if overflow occurs
*/
fun toInt() = value.toIntExact()
/**
* Converts this duration to an `Int` value without checking for overflow.
*/
internal fun toIntUnchecked() = value.toInt()
companion object {
/**
* The smallest supported value.
*/
val MIN: LongYears = LongYears(Long.MIN_VALUE)
/**
* The largest supported value.
*/
val MAX: LongYears = LongYears(Long.MAX_VALUE)
}
}
/**
* Converts this value to a duration of years.
*/
val Long.years: LongYears
get() = LongYears(this)
/**
* Multiplies this value by a duration of years.
* @throws ArithmeticException if overflow occurs
*/
operator fun Int.times(years: LongYears) = years * this
/**
* Multiplies this value by a duration of years.
* @throws ArithmeticException if overflow occurs
*/
operator fun Long.times(years: LongYears) = years * this
© 2015 - 2025 Weber Informatics LLC | Privacy Policy