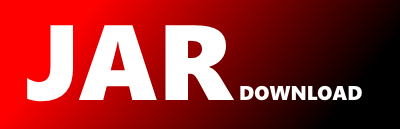
io.janusproject.kernel.bic.InternalEventBusSkill Maven / Gradle / Ivy
/*
* $Id: io/janusproject/kernel/bic/InternalEventBusSkill.java v2.0.3.1 2016-01-24 00:05:13$
*
* Janus platform is an open-source multiagent platform.
* More details on http://www.janusproject.io
*
* Copyright (C) 2014-2015 the original authors or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.janusproject.kernel.bic;
import java.util.ArrayList;
import java.util.List;
import java.util.Queue;
import java.util.UUID;
import java.util.concurrent.atomic.AtomicReference;
import com.google.common.collect.Queues;
import com.google.common.eventbus.AsyncSyncEventBus;
import com.google.inject.Inject;
import io.janusproject.services.logging.LogService;
import io.janusproject.services.spawn.SpawnService;
import io.janusproject.services.spawn.SpawnService.AgentKillException;
import io.sarl.core.AgentSpawned;
import io.sarl.core.Destroy;
import io.sarl.core.Initialize;
import io.sarl.lang.core.Address;
import io.sarl.lang.core.Agent;
import io.sarl.lang.core.Event;
import io.sarl.lang.core.EventListener;
import io.sarl.lang.core.Skill;
/** Janus implementation of an internal skill that provides
* an event bus to notify the different components of an agent.
*
* @author Sebastian Rodriguez
* @author Nicolas Gaud
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
class InternalEventBusSkill extends Skill implements InternalEventBusCapacity {
/** State of the owner.
*/
private final AtomicReference state = new AtomicReference<>(OwnerState.NEW);
/** Implementation of an EventListener linked to the owner of this skill.
*/
private final AgentEventListener agentAsEventListener;
/** Reference to the event bus.
* The event bus is the mean of routing of the events inside
* the context of the agents. The agent itself and the behaviors
* are connected to the event bus.
*/
@Inject
private AsyncSyncEventBus eventBus;
@Inject
private LogService logger;
@Inject
private SpawnService spawnService;
/** Address of the agent in the inner space.
*/
private final Address agentAddressInInnerDefaultSpace;
/** Collection of objects that are listening the event bus,
* except the owner of this skill.
*/
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy