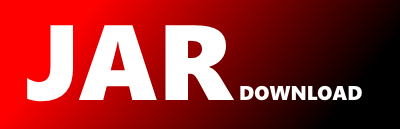
io.janusproject.util.AbstractDMultiMapView Maven / Gradle / Ivy
/*
* $Id: io/janusproject/util/AbstractDMultiMapView.java v2.0.3.1 2016-01-24 00:05:13$
*
* Janus platform is an open-source multiagent platform.
* More details on http://www.janusproject.io
*
* Copyright (C) 2014-2015 the original authors or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.janusproject.util;
import java.io.Serializable;
import java.util.AbstractCollection;
import java.util.AbstractList;
import java.util.AbstractMap;
import java.util.AbstractSet;
import java.util.Collection;
import java.util.Iterator;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import java.util.TreeSet;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.google.common.collect.Multimap;
import com.google.common.collect.Multiset;
import com.google.common.collect.Sets;
import io.janusproject.services.distributeddata.DMultiMap;
import io.janusproject.util.DataViewDelegate.Delegator;
/** A view on a Map that provides the API for the DMultiMap.
*
* @param - type of the keys.
* @param - type of the values.
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
public abstract class AbstractDMultiMapView extends AbstractMapView
implements DMultiMap, Serializable, Delegator> {
private static final long serialVersionUID = -6970650402150118406L;
private final String name;
private transient Map> map;
/**
* @param name - the name of the map.
*/
public AbstractDMultiMapView(String name) {
this.name = name;
}
@Override
public boolean isBackedCollection() {
return true;
}
/** Wrap the given values into a dedicated view.
*
* The replies view may be a {@link SingleKeyValueListView} or
* a {@link SingleKeyValueSetView} according to the type of the
* given values' collection.
*
* @param key - the key of the values.
* @param values - the values.
* @return the wrapper.
*/
Collection wrapValues(K key, Collection values) {
Object backEnd = DataViewDelegate.undelegate(values);
if (backEnd instanceof List>) {
return new SingleKeyValueListView(key, (List) values);
}
if (backEnd instanceof Set>) {
return new SingleKeyValueSetView(key, (Set) values);
}
throw new IllegalStateException("Unsupported type of the backend multimap: " + values.getClass()); //$NON-NLS-1$
}
/** Copy the given values.
*
* The replies collection may be a {@link List} or
* a {@link Set} according to the type of the
* given values' collection.
*
* @param values - the values.
* @return the copy.
*/
@SuppressWarnings({"unchecked", "checkstyle:illegaltype"})
Collection copyValues(Collection values) {
Object backEnd = DataViewDelegate.undelegate(values);
if (backEnd instanceof List>) {
return Lists.newArrayList(values);
}
if (backEnd instanceof TreeSet>) {
TreeSet c = (TreeSet) backEnd;
c = Sets.newTreeSet(c.comparator());
c.addAll(values);
return c;
}
if (backEnd instanceof LinkedHashSet>) {
return Sets.newLinkedHashSet(values);
}
if (backEnd instanceof Set>) {
return Sets.newHashSet(values);
}
throw new IllegalStateException("Unsupported type of the backend multimap: " + values.getClass()); //$NON-NLS-1$
}
@Override
public String getName() {
return this.name;
}
@Override
public boolean equals(Object obj) {
return getDelegatedObject().equals(DataViewDelegate.undelegate(obj));
}
@Override
public int hashCode() {
return getDelegatedObject().hashCode();
}
@Override
public String toString() {
return getDelegatedObject().toString();
}
@Override
public boolean containsKey(Object key) {
return getDelegatedObject().containsKey(key);
}
@Override
public boolean containsValue(Object value) {
return getDelegatedObject().containsValue(value);
}
@Override
public boolean containsEntry(Object key, Object value) {
return getDelegatedObject().containsEntry(key, value);
}
@Override
public int size() {
return getDelegatedObject().size();
}
@Override
public int valueCount(K key) {
Collection values = getDelegatedObject().get(key);
if (values != null && !values.isEmpty()) {
return values.size();
}
return 0;
}
@Override
public boolean isEmpty() {
return getDelegatedObject().isEmpty();
}
@Override
public boolean put(K key, V value) {
if (getDelegatedObject().put(key, value)) {
fireEntryAdded(key, value);
return true;
}
return false;
}
@SuppressWarnings("unchecked")
@Override
public boolean remove(Object key, Object value) {
if (getDelegatedObject().remove(key, value)) {
fireEntryRemoved((K) key, (V) value);
return true;
}
return false;
}
@SuppressWarnings("unchecked")
@Override
public Collection removeAll(Object key) {
Collection values = getDelegatedObject().removeAll(key);
if (values != null && !values.isEmpty()) {
K kkey = (K) key;
for (V value : values) {
fireEntryRemoved(kkey, value);
}
}
return values;
}
@Override
public boolean putAll(K key, Iterable extends V> values) {
boolean changed = false;
for (V value : values) {
if (getDelegatedObject().put(key, value)) {
changed = true;
fireEntryAdded(key, value);
}
}
return changed;
}
@Override
public boolean putAll(Multimap extends K, ? extends V> multimap) {
boolean changed = false;
for (Entry extends K, ? extends V> entry : multimap.entries()) {
if (getDelegatedObject().put(entry.getKey(), entry.getValue())) {
changed = true;
fireEntryAdded(entry.getKey(), entry.getValue());
}
}
return changed;
}
@Override
public Collection replaceValues(K key, Iterable extends V> values) {
return getDelegatedObject().replaceValues(key, values);
}
@Override
public void clear() {
if (!getDelegatedObject().isEmpty()) {
getDelegatedObject().clear();
fireCleared(false);
}
}
@Override
public Collection get(K key) {
return wrapValues(key, getDelegatedObject().get(key));
}
@Override
public Set keySet() {
return new KeySetView(getDelegatedObject().keySet());
}
@Override
public Collection> entries() {
return new EntryCollectionView(getDelegatedObject().entries());
}
@Override
public Collection values() {
return new ValueCollectionView(getDelegatedObject().entries());
}
@Override
public Multiset keys() {
return new MultisetView<>(this);
}
@Override
public Map> asMap() {
if (this.map == null) {
this.map = new MapView(getDelegatedObject().asMap());
}
return this.map;
}
/** A view on the values of specific key in a {@link AbstractDMultiMapView}.
*
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
protected class SingleKeyValueListView extends AbstractList implements Serializable, Delegator> {
private static final long serialVersionUID = 4290615787745160981L;
private final K key;
private final List values;
/**
* @param key - the key of the values.
* @param values - the values.
*/
public SingleKeyValueListView(K key, List values) {
this.key = key;
this.values = values;
}
@Override
public List getDelegatedObject() {
return this.values;
}
@Override
public boolean equals(Object obj) {
return getDelegatedObject().equals(DataViewDelegate.undelegate(obj));
}
@Override
public int hashCode() {
return getDelegatedObject().hashCode();
}
@Override
public String toString() {
return getDelegatedObject().toString();
}
@Override
public int size() {
return this.values.size();
}
@Override
public V get(int index) {
return this.values.get(index);
}
@Override
public Iterator iterator() {
return new ValueIterator(this.values.iterator());
}
@Override
public boolean add(V element) {
if (this.values.add(element)) {
fireEntryAdded(this.key, element);
return true;
}
return false;
}
@Override
public void clear() {
AbstractDMultiMapView.this.removeAll(this.key);
}
/** Iterator on the DMulitmap values.
*
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
private class ValueIterator implements Iterator {
private final Iterator iterator;
private V value;
ValueIterator(Iterator iterator) {
this.iterator = iterator;
}
@Override
public boolean hasNext() {
return this.iterator.hasNext();
}
@Override
public V next() {
this.value = this.iterator.next();
return this.value;
}
@SuppressWarnings("synthetic-access")
@Override
public void remove() {
this.iterator.remove();
fireEntryRemoved(SingleKeyValueListView.this.key, this.value);
}
}
}
/** A view on the values of specific key in a {@link AbstractDMultiMapView}.
*
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
protected class SingleKeyValueSetView extends AbstractSet implements Serializable, Delegator> {
private static final long serialVersionUID = 4290615787745160981L;
private final K key;
private final Set values;
/**
* @param key - the key of the values.
* @param values - the values.
*/
public SingleKeyValueSetView(K key, Set values) {
this.key = key;
this.values = values;
}
@Override
public Set getDelegatedObject() {
return this.values;
}
@Override
public boolean equals(Object obj) {
return getDelegatedObject().equals(DataViewDelegate.undelegate(obj));
}
@Override
public int hashCode() {
return getDelegatedObject().hashCode();
}
@Override
public String toString() {
return getDelegatedObject().toString();
}
@Override
public int size() {
return this.values.size();
}
@Override
public Iterator iterator() {
return new ValueIterator(this.values.iterator());
}
@Override
public boolean add(V element) {
if (this.values.add(element)) {
fireEntryAdded(this.key, element);
return true;
}
return false;
}
@Override
public void clear() {
AbstractDMultiMapView.this.removeAll(this.key);
}
/** Iterator on the DMultimap values.
*
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
private class ValueIterator implements Iterator {
private final Iterator iterator;
private V value;
ValueIterator(Iterator iterator) {
this.iterator = iterator;
}
@Override
public boolean hasNext() {
return this.iterator.hasNext();
}
@Override
public V next() {
this.value = this.iterator.next();
return this.value;
}
@SuppressWarnings("synthetic-access")
@Override
public void remove() {
this.iterator.remove();
fireEntryRemoved(SingleKeyValueSetView.this.key, this.value);
}
}
}
/** A view on the keys in a {@link AbstractDMultiMapView}.
*
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
protected class KeySetView extends AbstractSet implements Serializable, Delegator> {
private static final long serialVersionUID = -5008029594376258921L;
private final Set keys;
/**
* @param keys - the keys in the multimap.
*/
public KeySetView(Set keys) {
this.keys = keys;
}
@Override
public Set getDelegatedObject() {
return this.keys;
}
@Override
public boolean equals(Object obj) {
return getDelegatedObject().equals(DataViewDelegate.undelegate(obj));
}
@Override
public int hashCode() {
return getDelegatedObject().hashCode();
}
@Override
public String toString() {
return getDelegatedObject().toString();
}
@Override
public int size() {
return this.keys.size();
}
@Override
public Iterator iterator() {
return new KeyIterator(this.keys.iterator());
}
/** Iterator on the DMultiMap keys.
*
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
private class KeyIterator implements Iterator {
private final Iterator iterator;
private K key;
KeyIterator(Iterator iterator) {
this.iterator = iterator;
}
@Override
public boolean hasNext() {
return this.iterator.hasNext();
}
@Override
public K next() {
this.key = this.iterator.next();
return this.key;
}
@SuppressWarnings("unchecked")
@Override
public void remove() {
Collection oldValues = get(this.key);
V[] tab = (V[]) new Object[oldValues.size()];
oldValues.toArray(tab);
this.iterator.remove();
for (V value : tab) {
fireEntryRemoved(this.key, value);
}
}
}
}
/** A view on the entries in a {@link AbstractDMultiMapView}.
*
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
protected class EntryCollectionView extends AbstractCollection>
implements Serializable, Delegator>> {
private static final long serialVersionUID = 3746778947439539504L;
private final Collection> entries;
/**
* @param entries - the entries in the multimap.
*/
public EntryCollectionView(Collection> entries) {
this.entries = entries;
}
@Override
public Collection> getDelegatedObject() {
return AbstractDMultiMapView.this.getDelegatedObject().entries();
}
@Override
public boolean equals(Object obj) {
return getDelegatedObject().equals(DataViewDelegate.undelegate(obj));
}
@Override
public int hashCode() {
return getDelegatedObject().hashCode();
}
@Override
public String toString() {
return getDelegatedObject().toString();
}
@Override
public int size() {
return this.entries.size();
}
@Override
public Iterator> iterator() {
return new EntryIterator(this.entries.iterator());
}
@Override
public boolean add(Entry entry) {
if (this.entries.add(entry)) {
fireEntryAdded(entry.getKey(), entry.getValue());
return true;
}
return false;
}
/** Iterator on DMultiMap entries.
*
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
private class EntryIterator implements Iterator> {
private final Iterator> iterator;
private Entry entry;
EntryIterator(Iterator> iterator) {
this.iterator = iterator;
}
@Override
public boolean hasNext() {
return this.iterator.hasNext();
}
@Override
public Entry next() {
this.entry = this.iterator.next();
return this.entry;
}
@Override
public void remove() {
this.iterator.remove();
fireEntryRemoved(this.entry.getKey(), this.entry.getValue());
}
}
}
/** A view on all the values in a {@link AbstractDMultiMapView}.
*
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
protected class ValueCollectionView extends AbstractCollection implements Serializable, Delegator> {
private static final long serialVersionUID = -1288962742301549934L;
private final Collection> entries;
/**
* @param entries - the entries in the multimap.
*/
public ValueCollectionView(Collection> entries) {
this.entries = entries;
}
@Override
public Collection getDelegatedObject() {
return AbstractDMultiMapView.this.getDelegatedObject().values();
}
@Override
public boolean equals(Object obj) {
return getDelegatedObject().equals(DataViewDelegate.undelegate(obj));
}
@Override
public int hashCode() {
return getDelegatedObject().hashCode();
}
@Override
public String toString() {
return getDelegatedObject().toString();
}
@Override
public void clear() {
AbstractDMultiMapView.this.clear();
}
@Override
public int size() {
return this.entries.size();
}
@Override
public Iterator iterator() {
return new ValueIterator(this.entries.iterator());
}
/** Iterator on the DMultiMap values.
*
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
private class ValueIterator implements Iterator {
private final Iterator> iterator;
private Entry entry;
ValueIterator(Iterator> iterator) {
this.iterator = iterator;
}
@Override
public boolean hasNext() {
return this.iterator.hasNext();
}
@Override
public V next() {
this.entry = this.iterator.next();
return this.entry.getValue();
}
@Override
public void remove() {
this.iterator.remove();
fireEntryRemoved(this.entry.getKey(), this.entry.getValue());
}
}
}
/** Map view on a {@link AbstractDMultiMapView}.
*
* @author Stéphane Galland
* @version 2.0.3.1 2016-01-24 00:05:13
* @mavengroupid io.janusproject
* @mavenartifactid io.janusproject.kernel
*/
protected class MapView extends AbstractMap> implements Delegator