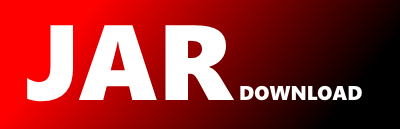
io.javalin.community.routing.dsl.DslFactory.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of routing-dsl Show documentation
Show all versions of routing-dsl Show documentation
Module provides set of DSLs for building routing in Javalin application
package io.javalin.community.routing.dsl
import io.javalin.community.routing.Route
import io.javalin.community.routing.Route.AFTER
import io.javalin.community.routing.Route.AFTER_MATCHED
import io.javalin.community.routing.Route.BEFORE
import io.javalin.community.routing.Route.BEFORE_MATCHED
import io.javalin.community.routing.Route.DELETE
import io.javalin.community.routing.Route.GET
import io.javalin.community.routing.Route.HEAD
import io.javalin.community.routing.Route.OPTIONS
import io.javalin.community.routing.Route.PATCH
import io.javalin.community.routing.Route.POST
import io.javalin.community.routing.Route.PUT
import io.javalin.community.routing.Routes
import io.javalin.http.ExceptionHandler
import io.javalin.http.Handler
import kotlin.reflect.KClass
/* Dsl setup */
interface RoutingDslFactory<
CONFIG : RoutingDslConfiguration,
ROUTE : DslRoute,
CONTEXT,
RESPONSE : Any
> {
fun createConfiguration(): CONFIG
fun createHandler(route: ROUTE): Handler
fun createExceptionHandler(handler: DslExceptionHandler): ExceptionHandler
}
open class RoutingDslConfiguration, CONTEXT, RESPONSE : Any> {
internal val routes = mutableSetOf()
internal val exceptionHandlers = mutableMapOf, DslExceptionHandler>()
fun get(path: String, handler: CONTEXT.() -> RESPONSE) = route(GET, path, handler)
fun post(path: String, handler: CONTEXT.() -> RESPONSE) = route(POST, path, handler)
fun put(path: String, handler: CONTEXT.() -> RESPONSE) = route(PUT, path, handler)
fun delete(path: String, handler: CONTEXT.() -> RESPONSE) = route(DELETE, path, handler)
fun patch(path: String, handler: CONTEXT.() -> RESPONSE) = route(PATCH, path, handler)
fun head(path: String, handler: CONTEXT.() -> RESPONSE) = route(HEAD, path, handler)
fun options(path: String, handler: CONTEXT.() -> RESPONSE) = route(OPTIONS, path, handler)
fun before(path: String = "", handler: CONTEXT.() -> RESPONSE) = route(BEFORE, path, handler)
fun beforeMatched(path: String = "", handler: CONTEXT.() -> RESPONSE) = route(BEFORE_MATCHED, path, handler)
fun after(path: String = "", handler: CONTEXT.() -> RESPONSE) = route(AFTER, path, handler)
fun afterMatched(path: String = "", handler: CONTEXT.() -> RESPONSE) = route(AFTER_MATCHED, path, handler)
fun routes(container: DslContainer) {
routes(container.routes())
container.exceptionHandlers().forEach { exception(it.type, it.handler) }
}
fun routes(routesToAdd: Collection>) = routesToAdd.forEach {
@Suppress("UNCHECKED_CAST")
routes.add(it as ROUTE)
}
fun route(method: Route, path: String, handler: CONTEXT.() -> RESPONSE) {
routes(
listOf(
DefaultDslRoute(
method = method,
path = path,
handler = handler
)
)
)
}
@Suppress("UNCHECKED_CAST")
fun exception(type: KClass, handler: DslExceptionHandler) {
if (exceptionHandlers.containsKey(type)) {
throw IllegalArgumentException("Exception handler for type ${type.simpleName} is already registered")
}
exceptionHandlers[type] = handler as DslExceptionHandler
}
}
interface DslContainer, CONTEXT, RESPONSE : Any> : Routes {
fun exceptionHandlers(): Collection> = emptySet()
fun route(method: Route, path: String, handler: CONTEXT.() -> RESPONSE): DslRoute =
DefaultDslRoute(
method = method,
path = path,
handler = handler
)
@Suppress("UNCHECKED_CAST")
fun exceptionHandler(type: KClass, handler: DslExceptionHandler): DslException =
DefaultDslException(
type = type as KClass,
handler = handler as DslExceptionHandler
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy