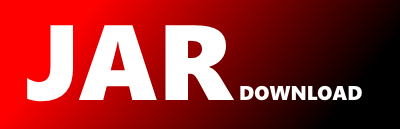
org.jenetics.BoltzmannSelector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jenetics Show documentation
Show all versions of jenetics Show documentation
Jenetics - Java Genetic Algorithm Library
/*
* Java Genetic Algorithm Library (jenetics-3.8.0).
* Copyright (c) 2007-2017 Franz Wilhelmstötter
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Author:
* Franz Wilhelmstötter ([email protected])
*/
package org.jenetics;
import static java.lang.Math.exp;
import static java.lang.String.format;
import static org.jenetics.internal.math.arithmetic.normalize;
import java.util.Arrays;
import org.jenetics.internal.util.Hash;
/**
*
* In this {@code Selector}, the probability for selection is defined as.
*
*
*
* where b controls the selection intensity, and
*
.
*
*
* fj denotes the fitness value of the
* jth individual.
*
* Positive values of b increases the selection probability of the phenotype
* with high fitness values. Negative values of b increases the selection
* probability of phenotypes with low fitness values. If b is zero the
* selection probability of all phenotypes is set to 1/N.
*
* @param the gene type.
* @param the BoltzmannSelector requires a number type.
*
* @author Franz Wilhelmstötter
* @since 1.0
* @version 3.2
*/
public final class BoltzmannSelector<
G extends Gene, G>,
N extends Number & Comparable super N>
>
extends ProbabilitySelector
{
private final double _b;
/**
* Create a new BoltzmannSelector with the given b value. High
* absolute values of b can create numerical overflows while
* calculating the selection probabilities.
*
* @param b the b value of this BoltzmannSelector
*/
public BoltzmannSelector(final double b) {
_b = b;
}
/**
* Create a new BoltzmannSelector with a default beta of 4.0.
*/
public BoltzmannSelector() {
this(4.0);
}
@Override
protected double[] probabilities(
final Population population,
final int count
) {
assert population != null : "Population must not be null. ";
assert !population.isEmpty() : "Population is empty.";
assert count > 0 : "Population to select must be greater than zero. ";
// Copy the fitness values to probabilities arrays.
final double[] fitness = new double[population.size()];
fitness[0] = population.get(0).getFitness().doubleValue();
double min = fitness[0];
double max = fitness[0];
for (int i = 1; i < fitness.length; ++i) {
fitness[i] = population.get(i).getFitness().doubleValue();
if (fitness[i] < min) min = fitness[i];
else if (fitness[i] > max) max = fitness[i];
}
final double diff = max - min;
if (eq(diff, 0.0)) {
// Set equal probabilities if diff (almost) zero.
Arrays.fill(fitness, 1.0/fitness.length);
} else {
// Scale fitness values to avoid overflow.
for (int i = fitness.length; --i >= 0;) {
fitness[i] = (fitness[i] - min)/diff;
}
// Apply the "Boltzmann" function.
for (int i = fitness.length; --i >= 0;) {
fitness[i] = exp(_b*fitness[i]);
}
}
return normalize(fitness);
}
@Override
public int hashCode() {
return Hash.of(getClass()).and(_b).value();
}
@Override
public boolean equals(final Object obj) {
return obj instanceof BoltzmannSelector &&
Double.compare(((BoltzmannSelector)obj)._b, _b) == 0;
}
@Override
public String toString() {
return format("BoltzmannSelector[b=%f]", _b);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy