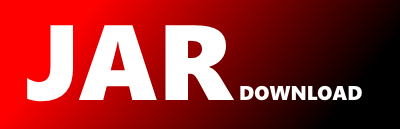
io.jexxa.core.FluentInterceptor Maven / Gradle / Ivy
package io.jexxa.core;
import io.jexxa.adapterapi.invocation.InvocationContext;
import io.jexxa.adapterapi.invocation.InvocationManager;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.function.Consumer;
@SuppressWarnings("unused")
public final class FluentInterceptor
{
private final Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy