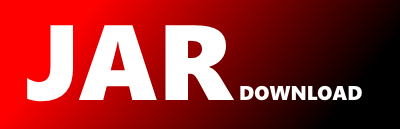
io.joshworks.stream.client.sse.SseConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stream-client Show documentation
Show all versions of stream-client Show documentation
Resilient client for Server sent events and WebSockets
package io.joshworks.stream.client.sse;
import io.joshworks.stream.client.ClientConfiguration;
import io.joshworks.stream.client.ConnectionMonitor;
import org.xnio.XnioWorker;
import java.util.concurrent.ScheduledExecutorService;
import java.util.function.Consumer;
/**
* Created by Josh Gontijo on 6/8/17.
*/
public class SseConfiguration extends ClientConfiguration {
private SseClientCallback clientCallback;
private String lastEventId;
private Runnable onOpen = () -> {};
private Consumer onEvent = (eventData) -> {};
private Consumer onClose = (lastEventId) -> {};
private Consumer onError = (e) -> {};
public SseConfiguration(String url, XnioWorker worker, ScheduledExecutorService scheduler, ConnectionMonitor register) {
super(url, worker, scheduler, register);
}
public SseConfiguration(String url, XnioWorker worker, ScheduledExecutorService scheduler,
ConnectionMonitor register, SseClientCallback clientCallback) {
super(url, worker, scheduler, register);
this.clientCallback = clientCallback;
}
public SseConfiguration onOpen(Runnable onOpen) {
this.onOpen = onOpen;
return this;
}
public SseConfiguration onEvent(Consumer onEvent) {
this.onEvent = onEvent;
return this;
}
public SseConfiguration onClose(Consumer onClose) {
this.onClose = onClose;
return this;
}
public SseConfiguration onError(Consumer onError) {
this.onError = onError;
return this;
}
public SseConfiguration lastEventId(String lastEventId) {
this.lastEventId = lastEventId;
return this;
}
public SseConfiguration retryInterval(int retryInterval) {
this.retryInterval = retryInterval;
return this;
}
public SseConfiguration maxRetries(int maxRetries) {
this.maxRetries = maxRetries;
return this;
}
public SseConfiguration autoReconnect(boolean autoReconnect) {
this.autoReconnect = autoReconnect;
return this;
}
public SSEConnection connect() {
clientCallback = clientCallback == null ? createClientCallback() : clientCallback;
SSEConnection connection = new SSEConnection(url, worker, scheduler, monitor, retryInterval, maxRetries, autoReconnect, clientCallback);
connection.connect(lastEventId);
return connection;
}
private SseClientCallback createClientCallback() {
return new SseClientCallback() {
@Override
public void onEvent(EventData event) {
onEvent.accept(event);
}
@Override
public void onOpen() {
onOpen.run();
}
@Override
public void onClose(String lastEventId) {
onClose.accept(lastEventId);
}
@Override
public void onError(Exception e) {
onError.accept(e);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy