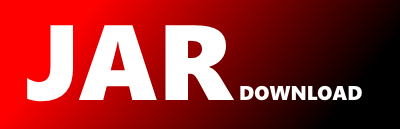
joynr.types.ProviderQos Maven / Gradle / Ivy
/*
*
* Copyright (C) 2011 - 2018 BMW Car IT GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// #####################################################
//#######################################################
//### ###
//## WARNING: This file is generated. DO NOT EDIT ##
//## All changes will be lost! ##
//### ###
//#######################################################
// #####################################################
package joynr.types;
import java.io.Serializable;
import io.joynr.subtypes.JoynrType;
import joynr.types.CustomParameter;
import joynr.types.ProviderScope;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonIgnore;
// NOTE: serialVersionUID is not defined since we don't support Franca versions right now.
// The compiler will generate a serialVersionUID based on the class and its members
// (cf. http://docs.oracle.com/javase/6/docs/platform/serialization/spec/class.html#4100),
// which is probably more restrictive than what we want.
/**
* the provider quality of service settings
*/
@SuppressWarnings("serial")
public class ProviderQos implements Serializable, JoynrType {
public static final int MAJOR_VERSION = 0;
public static final int MINOR_VERSION = 0;
@JsonProperty("customParameters")
private CustomParameter[] customParameters = { };
@JsonProperty("priority")
private Long priority;
@JsonProperty("scope")
private ProviderScope scope;
@JsonProperty("supportsOnChangeSubscriptions")
private Boolean supportsOnChangeSubscriptions;
/**
* Default Constructor
*/
public ProviderQos() {
this.priority = 0L;
this.scope = ProviderScope.GLOBAL;
this.supportsOnChangeSubscriptions = false;
}
/**
* Copy constructor
*
* @param providerQosObj reference to the object to be copied
*/
public ProviderQos(ProviderQos providerQosObj) {
this.customParameters = providerQosObj.customParameters;
this.priority = providerQosObj.priority;
this.scope = providerQosObj.scope;
this.supportsOnChangeSubscriptions = providerQosObj.supportsOnChangeSubscriptions;
}
/**
* Parameterized constructor
*
* @param customParameters the list of Provider-Specific and InterfaceSpecific QoS Parameters
* @param priority the priority value of the provider used for discovery
* @param scope the scope of the provider (e.g. if it is to be used only with the local cluster controller)
* @param supportsOnChangeSubscriptions true, if the provider supports onChangeSubscriptions, false otherwise
*/
public ProviderQos(
CustomParameter[] customParameters,
Long priority,
ProviderScope scope,
Boolean supportsOnChangeSubscriptions
) {
if (customParameters != null) {
this.customParameters = customParameters.clone();
}
this.priority = priority;
this.scope = scope;
this.supportsOnChangeSubscriptions = supportsOnChangeSubscriptions;
}
/**
* Gets CustomParameters
*
* @return the list of Provider-Specific and InterfaceSpecific QoS Parameters
*/
@JsonIgnore
public CustomParameter[] getCustomParameters() {
if (customParameters != null) {
return customParameters.clone();
} else {
return null;
}
}
/**
* Sets CustomParameters
*
* @param customParameters the list of Provider-Specific and InterfaceSpecific QoS Parameters
*/
@JsonIgnore
public void setCustomParameters(CustomParameter[] customParameters) {
if (customParameters == null) {
throw new IllegalArgumentException("setting customParameters to null is not allowed");
}
this.customParameters = customParameters.clone();
}
/**
* Gets Priority
*
* @return the priority value of the provider used for discovery
*/
@JsonIgnore
public Long getPriority() {
return priority;
}
/**
* Sets Priority
*
* @param priority the priority value of the provider used for discovery
*/
@JsonIgnore
public void setPriority(Long priority) {
if (priority == null) {
throw new IllegalArgumentException("setting priority to null is not allowed");
}
this.priority = priority;
}
/**
* Gets Scope
*
* @return the scope of the provider (e.g. if it is to be used only with the local cluster controller)
*/
@JsonIgnore
public ProviderScope getScope() {
return scope;
}
/**
* Sets Scope
*
* @param scope the scope of the provider (e.g. if it is to be used only with the local cluster controller)
*/
@JsonIgnore
public void setScope(ProviderScope scope) {
if (scope == null) {
throw new IllegalArgumentException("setting scope to null is not allowed");
}
this.scope = scope;
}
/**
* Gets SupportsOnChangeSubscriptions
*
* @return true, if the provider supports onChangeSubscriptions, false otherwise
*/
@JsonIgnore
public Boolean getSupportsOnChangeSubscriptions() {
return supportsOnChangeSubscriptions;
}
/**
* Sets SupportsOnChangeSubscriptions
*
* @param supportsOnChangeSubscriptions true, if the provider supports onChangeSubscriptions, false otherwise
*/
@JsonIgnore
public void setSupportsOnChangeSubscriptions(Boolean supportsOnChangeSubscriptions) {
if (supportsOnChangeSubscriptions == null) {
throw new IllegalArgumentException("setting supportsOnChangeSubscriptions to null is not allowed");
}
this.supportsOnChangeSubscriptions = supportsOnChangeSubscriptions;
}
/**
* Stringifies the class
*
* @return stringified class content
*/
@Override
public String toString() {
return "ProviderQos ["
+ "customParameters=" + java.util.Arrays.toString(this.customParameters) + ", "
+ "priority=" + this.priority + ", "
+ "scope=" + this.scope + ", "
+ "supportsOnChangeSubscriptions=" + this.supportsOnChangeSubscriptions
+ "]";
}
/**
* Check for equality
*
* @param obj Reference to the object to compare to
* @return true, if objects are equal, false otherwise
*/
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
ProviderQos other = (ProviderQos) obj;
if (this.customParameters == null) {
if (other.customParameters != null) {
return false;
}
} else if (!java.util.Arrays.deepEquals(this.customParameters, other.customParameters)) {
return false;
}
if (this.priority == null) {
if (other.priority != null) {
return false;
}
} else if (!this.priority.equals(other.priority)) {
return false;
}
if (this.scope == null) {
if (other.scope != null) {
return false;
}
} else if (!this.scope.equals(other.scope)) {
return false;
}
if (this.supportsOnChangeSubscriptions == null) {
if (other.supportsOnChangeSubscriptions != null) {
return false;
}
} else if (!this.supportsOnChangeSubscriptions.equals(other.supportsOnChangeSubscriptions)) {
return false;
}
return true;
}
/**
* Calculate code for hashing based on member contents
*
* @return The calculated hash code
*/
@Override
public int hashCode() {
int result = 1;
final int prime = 31;
result = prime * result + ((this.customParameters == null) ? 0 : java.util.Arrays.hashCode(this.customParameters));
result = prime * result + ((this.priority == null) ? 0 : this.priority.hashCode());
result = prime * result + ((this.scope == null) ? 0 : this.scope.hashCode());
result = prime * result + ((this.supportsOnChangeSubscriptions == null) ? 0 : this.supportsOnChangeSubscriptions.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy