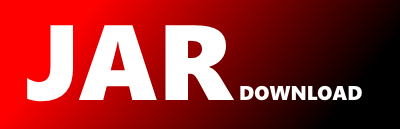
io.joynr.generator.cpp.joynrmessaging.InterfaceJoynrMessagingConnectorHTemplate Maven / Gradle / Ivy
package io.joynr.generator.cpp.joynrmessaging;
import com.google.common.base.Objects;
import com.google.inject.Inject;
import io.joynr.generator.cpp.util.JoynrCppGeneratorExtensions;
import io.joynr.generator.cpp.util.TemplateBase;
import org.eclipse.emf.common.util.EList;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.StringExtensions;
import org.franca.core.franca.FAttribute;
import org.franca.core.franca.FInterface;
import org.franca.core.franca.FMethod;
@SuppressWarnings("all")
public class InterfaceJoynrMessagingConnectorHTemplate {
@Inject
@Extension
private TemplateBase _templateBase;
@Inject
@Extension
private JoynrCppGeneratorExtensions _joynrCppGeneratorExtensions;
public CharSequence generate(final FInterface serviceInterface) {
CharSequence _xblockexpression = null;
{
final String interfaceName = this._joynrCppGeneratorExtensions.joynrName(serviceInterface);
String _packagePathWithJoynrPrefix = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(serviceInterface, "_");
String _plus = ("GENERATED_INTERFACE_" + _packagePathWithJoynrPrefix);
String _plus_1 = (_plus + "_");
String _plus_2 = (_plus_1 + interfaceName);
String _plus_3 = (_plus_2 + "JoynrMessagingConnector_h");
final String headerGuard = _plus_3.toUpperCase();
StringConcatenation _builder = new StringConcatenation();
CharSequence _warning = this._templateBase.warning();
_builder.append(_warning, "");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("#ifndef ");
_builder.append(headerGuard, "");
_builder.newLineIfNotEmpty();
_builder.append("#define ");
_builder.append(headerGuard, "");
_builder.newLineIfNotEmpty();
_builder.newLine();
String _dllExportIncludeStatement = this._joynrCppGeneratorExtensions.getDllExportIncludeStatement();
_builder.append(_dllExportIncludeStatement, "");
_builder.newLineIfNotEmpty();
_builder.append("#include \"");
String _packagePathWithJoynrPrefix_1 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(serviceInterface, "/");
_builder.append(_packagePathWithJoynrPrefix_1, "");
_builder.append("/I");
_builder.append(interfaceName, "");
_builder.append("Connector.h\"");
_builder.newLineIfNotEmpty();
_builder.append("#include \"joynr/AbstractJoynrMessagingConnector.h\"");
_builder.newLine();
_builder.append("#include \"joynr/JoynrMessagingConnectorFactory.h\"");
_builder.newLine();
_builder.newLine();
_builder.append("namespace joynr {");
_builder.newLine();
_builder.append("\t");
_builder.append("class MessagingQos;");
_builder.newLine();
_builder.append("\t");
_builder.append("class IJoynrMessageSender;");
_builder.newLine();
_builder.append("\t");
_builder.append("class SubscriptionManager;");
_builder.newLine();
_builder.append("}");
_builder.newLine();
_builder.newLine();
String _namespaceStarter = this._joynrCppGeneratorExtensions.getNamespaceStarter(serviceInterface);
_builder.append(_namespaceStarter, "");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.newLine();
_builder.append("class ");
String _dllExportMacro = this._joynrCppGeneratorExtensions.getDllExportMacro();
_builder.append(_dllExportMacro, "");
_builder.append(" ");
_builder.append(interfaceName, "");
_builder.append("JoynrMessagingConnector : public I");
_builder.append(interfaceName, "");
_builder.append("Connector, virtual public joynr::AbstractJoynrMessagingConnector {");
_builder.newLineIfNotEmpty();
_builder.append("public:");
_builder.newLine();
_builder.append(" ");
_builder.append(interfaceName, " ");
_builder.append("JoynrMessagingConnector(");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.append("joynr::IJoynrMessageSender* messageSender,");
_builder.newLine();
_builder.append(" ");
_builder.append("joynr::SubscriptionManager* subscriptionManager,");
_builder.newLine();
_builder.append(" ");
_builder.append("const QString &domain,");
_builder.newLine();
_builder.append(" ");
_builder.append("const QString proxyParticipantId,");
_builder.newLine();
_builder.append(" ");
_builder.append("const QString& providerParticipantId,");
_builder.newLine();
_builder.append(" ");
_builder.append("const joynr::MessagingQos &qosSettings,");
_builder.newLine();
_builder.append(" ");
_builder.append("joynr::IClientCache *cache,");
_builder.newLine();
_builder.append(" ");
_builder.append("bool cached,");
_builder.newLine();
_builder.append(" ");
_builder.append("qint64 reqCacheDataFreshness_ms);");
_builder.newLine();
_builder.newLine();
_builder.newLine();
_builder.append("\t");
_builder.append("virtual bool usesClusterController() const;");
_builder.newLine();
_builder.append("\t");
_builder.newLine();
_builder.append("\t");
_builder.append("virtual ~");
_builder.append(interfaceName, " ");
_builder.append("JoynrMessagingConnector(){}");
_builder.newLineIfNotEmpty();
_builder.newLine();
{
EList _attributes = this._joynrCppGeneratorExtensions.getAttributes(serviceInterface);
for(final FAttribute attribute : _attributes) {
_builder.append("\t");
final String returnType = this._joynrCppGeneratorExtensions.getMappedDatatypeOrList(attribute);
_builder.newLineIfNotEmpty();
_builder.append("\t");
final String attributeName = this._joynrCppGeneratorExtensions.joynrName(attribute);
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("virtual void get");
String _firstUpper = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper, " ");
_builder.append("(joynr::RequestStatus& status, ");
String _mappedDatatypeOrList = this._joynrCppGeneratorExtensions.getMappedDatatypeOrList(attribute);
_builder.append(_mappedDatatypeOrList, " ");
_builder.append("& ");
_builder.append(attributeName, " ");
_builder.append(");");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("virtual void get");
String _firstUpper_1 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_1, " ");
_builder.append("(QSharedPointer > future, QSharedPointer< joynr::ICallback<");
String _mappedDatatypeOrList_2 = this._joynrCppGeneratorExtensions.getMappedDatatypeOrList(attribute);
_builder.append(_mappedDatatypeOrList_2, " ");
_builder.append("> > callBack);");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("virtual void get");
String _firstUpper_2 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_2, " ");
_builder.append("(QSharedPointer > future);");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("virtual void get");
String _firstUpper_3 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_3, " ");
_builder.append("(QSharedPointer > callBack);");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("\t");
_builder.append("virtual void set");
String _firstUpper_4 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_4, " ");
_builder.append("(QSharedPointer > callBack, ");
String _mappedDatatypeOrList_5 = this._joynrCppGeneratorExtensions.getMappedDatatypeOrList(attribute);
_builder.append(_mappedDatatypeOrList_5, " ");
_builder.append(" ");
_builder.append(attributeName, " ");
_builder.append(");");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("virtual void set");
String _firstUpper_5 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_5, " ");
_builder.append("(joynr::RequestStatus &status, const ");
String _mappedDatatypeOrList_6 = this._joynrCppGeneratorExtensions.getMappedDatatypeOrList(attribute);
_builder.append(_mappedDatatypeOrList_6, " ");
_builder.append("& ");
_builder.append(attributeName, " ");
_builder.append(");");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("virtual void set");
String _firstUpper_6 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_6, " ");
_builder.append("(QSharedPointer > future, QSharedPointer< joynr::ICallback > callBack, ");
String _mappedDatatypeOrList_7 = this._joynrCppGeneratorExtensions.getMappedDatatypeOrList(attribute);
_builder.append(_mappedDatatypeOrList_7, " ");
_builder.append(" ");
_builder.append(attributeName, " ");
_builder.append(");");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("virtual void set");
String _firstUpper_7 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_7, " ");
_builder.append("(QSharedPointer > future, ");
String _mappedDatatypeOrList_8 = this._joynrCppGeneratorExtensions.getMappedDatatypeOrList(attribute);
_builder.append(_mappedDatatypeOrList_8, " ");
_builder.append(" ");
_builder.append(attributeName, " ");
_builder.append(");");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("\t");
_builder.append("virtual QString subscribeTo");
String _firstUpper_8 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_8, " ");
_builder.append("(QSharedPointer > subscriptionListener, QSharedPointer subscriptionQos);");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("virtual void unsubscribeFrom");
String _firstUpper_9 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_9, " ");
_builder.append("(QString& subscriptionId);");
_builder.newLineIfNotEmpty();
}
}
_builder.append(" ");
_builder.newLine();
{
EList _methods = this._joynrCppGeneratorExtensions.getMethods(serviceInterface);
for(final FMethod method : _methods) {
_builder.append("\t");
final String methodName = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.newLineIfNotEmpty();
{
Iterable _mappedOutputParameter = this._joynrCppGeneratorExtensions.getMappedOutputParameter(method);
String _head = IterableExtensions.head(_mappedOutputParameter);
boolean _equals = Objects.equal(_head, "void");
if (_equals) {
_builder.append("\t");
_builder.append("virtual void ");
_builder.append(methodName, " ");
_builder.append(" (joynr::RequestStatus &status ");
String _commaSeperatedTypedParameterList = this._joynrCppGeneratorExtensions.getCommaSeperatedTypedParameterList(method);
String _prependCommaIfNotEmpty = this._joynrCppGeneratorExtensions.prependCommaIfNotEmpty(_commaSeperatedTypedParameterList);
_builder.append(_prependCommaIfNotEmpty, " ");
_builder.append(");");
_builder.newLineIfNotEmpty();
} else {
_builder.append("\t");
_builder.append("virtual void ");
_builder.append(methodName, " ");
_builder.append(" (joynr::RequestStatus &status");
String _commaSeperatedTypedOutputParameterList = this._joynrCppGeneratorExtensions.getCommaSeperatedTypedOutputParameterList(method);
String _prependCommaIfNotEmpty_1 = this._joynrCppGeneratorExtensions.prependCommaIfNotEmpty(_commaSeperatedTypedOutputParameterList);
_builder.append(_prependCommaIfNotEmpty_1, " ");
String _commaSeperatedTypedParameterList_1 = this._joynrCppGeneratorExtensions.getCommaSeperatedTypedParameterList(method);
String _prependCommaIfNotEmpty_2 = this._joynrCppGeneratorExtensions.prependCommaIfNotEmpty(_commaSeperatedTypedParameterList_1);
_builder.append(_prependCommaIfNotEmpty_2, " ");
_builder.append(");");
_builder.newLineIfNotEmpty();
}
}
_builder.append("\t");
_builder.append("virtual void ");
_builder.append(methodName, " ");
_builder.append(" (QSharedPointer _mappedOutputParameter_1 = this._joynrCppGeneratorExtensions.getMappedOutputParameter(method);
String _head_1 = IterableExtensions.head(_mappedOutputParameter_1);
_builder.append(_head_1, " ");
_builder.append("> > future, QSharedPointer< joynr::ICallback<");
Iterable _mappedOutputParameter_2 = this._joynrCppGeneratorExtensions.getMappedOutputParameter(method);
String _head_2 = IterableExtensions.head(_mappedOutputParameter_2);
_builder.append(_head_2, " ");
_builder.append(" > > callBack ");
String _commaSeperatedTypedParameterList_2 = this._joynrCppGeneratorExtensions.getCommaSeperatedTypedParameterList(method);
String _prependCommaIfNotEmpty_3 = this._joynrCppGeneratorExtensions.prependCommaIfNotEmpty(_commaSeperatedTypedParameterList_2);
_builder.append(_prependCommaIfNotEmpty_3, " ");
_builder.append(");");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("virtual void ");
_builder.append(methodName, " ");
_builder.append(" (QSharedPointer _mappedOutputParameter_3 = this._joynrCppGeneratorExtensions.getMappedOutputParameter(method);
String _head_3 = IterableExtensions.head(_mappedOutputParameter_3);
_builder.append(_head_3, " ");
_builder.append("> > future ");
String _commaSeperatedTypedParameterList_3 = this._joynrCppGeneratorExtensions.getCommaSeperatedTypedParameterList(method);
String _prependCommaIfNotEmpty_4 = this._joynrCppGeneratorExtensions.prependCommaIfNotEmpty(_commaSeperatedTypedParameterList_3);
_builder.append(_prependCommaIfNotEmpty_4, " ");
_builder.append(");");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("virtual void ");
_builder.append(methodName, " ");
_builder.append(" (QSharedPointer _mappedOutputParameter_4 = this._joynrCppGeneratorExtensions.getMappedOutputParameter(method);
String _head_4 = IterableExtensions.head(_mappedOutputParameter_4);
_builder.append(_head_4, " ");
_builder.append("> > callBack ");
String _commaSeperatedTypedParameterList_4 = this._joynrCppGeneratorExtensions.getCommaSeperatedTypedParameterList(method);
String _prependCommaIfNotEmpty_5 = this._joynrCppGeneratorExtensions.prependCommaIfNotEmpty(_commaSeperatedTypedParameterList_4);
_builder.append(_prependCommaIfNotEmpty_5, " ");
_builder.append(");");
_builder.newLineIfNotEmpty();
}
}
_builder.append("};");
_builder.newLine();
String _namespaceEnder = this._joynrCppGeneratorExtensions.getNamespaceEnder(serviceInterface);
_builder.append(_namespaceEnder, "");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("namespace joynr {");
_builder.newLine();
_builder.append("\t");
_builder.newLine();
_builder.append("// Helper class for use by the JoynrMessagingConnectorFactory");
_builder.newLine();
_builder.append("// This class creates instances of ");
_builder.append(interfaceName, "");
_builder.append("JoynrMessagingConnector");
_builder.newLineIfNotEmpty();
_builder.append("template <>");
_builder.newLine();
_builder.append("class JoynrMessagingConnectorFactoryHelper <");
String _packagePathWithJoynrPrefix_2 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(serviceInterface, "::");
_builder.append(_packagePathWithJoynrPrefix_2, "");
_builder.append("::I");
_builder.append(interfaceName, "");
_builder.append("Connector> {");
_builder.newLineIfNotEmpty();
_builder.append("public:");
_builder.newLine();
_builder.append(" ");
String _packagePathWithJoynrPrefix_3 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(serviceInterface, "::");
_builder.append(_packagePathWithJoynrPrefix_3, " ");
_builder.append("::");
_builder.append(interfaceName, " ");
_builder.append("JoynrMessagingConnector* create(");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.append("joynr::IJoynrMessageSender* messageSender,");
_builder.newLine();
_builder.append(" ");
_builder.append("joynr::SubscriptionManager* subscriptionManager,");
_builder.newLine();
_builder.append(" ");
_builder.append("const QString &domain,");
_builder.newLine();
_builder.append(" ");
_builder.append("const QString proxyParticipantId,");
_builder.newLine();
_builder.append(" ");
_builder.append("const QString& providerParticipantId,");
_builder.newLine();
_builder.append(" ");
_builder.append("const joynr::MessagingQos &qosSettings,");
_builder.newLine();
_builder.append(" ");
_builder.append("joynr::IClientCache *cache,");
_builder.newLine();
_builder.append(" ");
_builder.append("bool cached,");
_builder.newLine();
_builder.append(" ");
_builder.append("qint64 reqCacheDataFreshness_ms");
_builder.newLine();
_builder.append(" ");
_builder.append(") {");
_builder.newLine();
_builder.append(" ");
_builder.append("return new ");
String _packagePathWithJoynrPrefix_4 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(serviceInterface, "::");
_builder.append(_packagePathWithJoynrPrefix_4, " ");
_builder.append("::");
_builder.append(interfaceName, " ");
_builder.append("JoynrMessagingConnector(");
_builder.newLineIfNotEmpty();
_builder.append(" \t\t");
_builder.append("messageSender,");
_builder.newLine();
_builder.append(" \t\t");
_builder.append("subscriptionManager,");
_builder.newLine();
_builder.append(" \t\t");
_builder.append("domain,");
_builder.newLine();
_builder.append(" \t\t");
_builder.append("proxyParticipantId,");
_builder.newLine();
_builder.append(" \t\t");
_builder.append("providerParticipantId,");
_builder.newLine();
_builder.append(" \t\t");
_builder.append("qosSettings,");
_builder.newLine();
_builder.append(" \t\t");
_builder.append("cache,");
_builder.newLine();
_builder.append(" \t\t");
_builder.append("cached,");
_builder.newLine();
_builder.append(" \t\t");
_builder.append("reqCacheDataFreshness_ms");
_builder.newLine();
_builder.append(" ");
_builder.append(");");
_builder.newLine();
_builder.append(" ");
_builder.append("}");
_builder.newLine();
_builder.append("};");
_builder.newLine();
_builder.newLine();
_builder.append("} // namespace joynr");
_builder.newLine();
_builder.append("#endif // ");
_builder.append(headerGuard, "");
_builder.newLineIfNotEmpty();
_xblockexpression = (_builder);
}
return _xblockexpression;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy