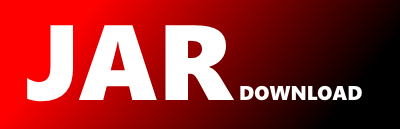
io.joynr.generator.cpp.util.InterfaceUtil Maven / Gradle / Ivy
package io.joynr.generator.cpp.util;
import com.google.common.base.Objects;
import com.google.inject.Inject;
import io.joynr.generator.cpp.util.JoynrCppGeneratorExtensions;
import org.eclipse.emf.common.util.EList;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.StringExtensions;
import org.franca.core.franca.FAttribute;
import org.franca.core.franca.FInterface;
import org.franca.core.franca.FMethod;
@SuppressWarnings("all")
public class InterfaceUtil {
@Inject
@Extension
private JoynrCppGeneratorExtensions _joynrCppGeneratorExtensions;
public CharSequence printFutureParamDefinition() {
StringConcatenation _builder = new StringConcatenation();
_builder.append("* @param future With this object, you will be able to query the joynrInternalStatus and result of");
_builder.newLine();
_builder.append("* the request.");
_builder.newLine();
return _builder;
}
public CharSequence printCallbackParamDefinition() {
StringConcatenation _builder = new StringConcatenation();
_builder.append("* @param callback A callback typed according to the expected return value type.");
_builder.newLine();
_builder.append("* This class will be called, together with a return value when the request succeeds");
_builder.newLine();
_builder.append("* or fails.");
_builder.newLine();
return _builder;
}
public CharSequence produceSyncGetters(final FInterface serviceInterface, final boolean pure) {
StringConcatenation _builder = new StringConcatenation();
{
EList _attributes = this._joynrCppGeneratorExtensions.getAttributes(serviceInterface);
for(final FAttribute attribute : _attributes) {
final String returnType = this._joynrCppGeneratorExtensions.getMappedDatatypeOrList(attribute);
_builder.newLineIfNotEmpty();
final String attributeName = this._joynrCppGeneratorExtensions.joynrName(attribute);
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Synchronous getter for the ");
_builder.append(attributeName, "");
_builder.append(" attribute.");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
_builder.append("* @param joynrInternalStatus The joynrInternalStatus of the request which will be returned to the caller.");
_builder.newLine();
_builder.append("* @param result The result that will be returned to the caller.");
_builder.newLine();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual void get");
String _firstUpper = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("joynr::RequestStatus& joynrInternalStatus,");
_builder.newLine();
_builder.append("\t\t");
_builder.append(returnType, " ");
_builder.append("& result");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.newLine();
}
}
return _builder;
}
public CharSequence produceAsyncGetters(final FInterface serviceInterface, final boolean pure) {
StringConcatenation _builder = new StringConcatenation();
{
EList _attributes = this._joynrCppGeneratorExtensions.getAttributes(serviceInterface);
for(final FAttribute attribute : _attributes) {
final String returnType = this._joynrCppGeneratorExtensions.getMappedDatatypeOrList(attribute);
_builder.newLineIfNotEmpty();
final String attributeName = this._joynrCppGeneratorExtensions.joynrName(attribute);
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Asynchronous getter for the ");
_builder.append(attributeName, "");
_builder.append(" attribute.");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
CharSequence _printCallbackParamDefinition = this.printCallbackParamDefinition();
_builder.append(_printCallbackParamDefinition, "");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual void get");
String _firstUpper = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("QSharedPointer > callback");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Asynchronous getter for the location attribute.");
_builder.newLine();
_builder.append("*");
_builder.newLine();
_builder.append("* @param future With this object, you will be able to query the joynrInternalStatus and result of");
_builder.newLine();
_builder.append("* the request.");
_builder.newLine();
CharSequence _printCallbackParamDefinition_1 = this.printCallbackParamDefinition();
_builder.append(_printCallbackParamDefinition_1, "");
_builder.newLineIfNotEmpty();
CharSequence _printFutureParamDefinition = this.printFutureParamDefinition();
_builder.append(_printFutureParamDefinition, "");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual void get");
String _firstUpper_1 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_1, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("QSharedPointer > future,");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("QSharedPointer > callback");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Asynchronous getter for the location attribute.");
_builder.newLine();
_builder.append("*");
_builder.newLine();
CharSequence _printFutureParamDefinition_1 = this.printFutureParamDefinition();
_builder.append(_printFutureParamDefinition_1, "");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual void get");
String _firstUpper_2 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_2, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("QSharedPointer > future");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
}
}
return _builder;
}
public CharSequence produceSyncSetters(final FInterface serviceInterface, final boolean pure) {
StringConcatenation _builder = new StringConcatenation();
{
EList _attributes = this._joynrCppGeneratorExtensions.getAttributes(serviceInterface);
for(final FAttribute attribute : _attributes) {
final String returnType = this._joynrCppGeneratorExtensions.getMappedDatatypeOrList(attribute);
_builder.newLineIfNotEmpty();
final String attributeName = this._joynrCppGeneratorExtensions.joynrName(attribute);
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Synchronous setter for the ");
_builder.append(attributeName, "");
_builder.append(" attribute.");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
_builder.append("* @param joynrInternalStatus The joynrInternalStatus of the request which will be returned to the caller.");
_builder.newLine();
_builder.append("* @param ");
String _firstLower = StringExtensions.toFirstLower(returnType);
_builder.append(_firstLower, "");
_builder.append(" The value to set.");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual void set");
String _firstUpper = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("joynr::RequestStatus& joynrInternalStatus,");
_builder.newLine();
_builder.append("\t\t");
_builder.append("const ");
_builder.append(returnType, " ");
_builder.append("& ");
String _firstLower_1 = StringExtensions.toFirstLower(attributeName);
_builder.append(_firstLower_1, " ");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
}
}
return _builder;
}
public CharSequence produceAsyncSetters(final FInterface serviceInterface, final boolean pure) {
StringConcatenation _builder = new StringConcatenation();
{
EList _attributes = this._joynrCppGeneratorExtensions.getAttributes(serviceInterface);
for(final FAttribute attribute : _attributes) {
final String returnType = this._joynrCppGeneratorExtensions.getMappedDatatypeOrList(attribute);
_builder.newLineIfNotEmpty();
final String attributeName = this._joynrCppGeneratorExtensions.joynrName(attribute);
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Asynchronous setter for the ");
_builder.append(attributeName, "");
_builder.append(" attribute.");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
CharSequence _printCallbackParamDefinition = this.printCallbackParamDefinition();
_builder.append(_printCallbackParamDefinition, "");
_builder.newLineIfNotEmpty();
_builder.append("* @param ");
String _firstLower = StringExtensions.toFirstLower(returnType);
_builder.append(_firstLower, "");
_builder.append(" The value to set.");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual void set");
String _firstUpper = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("QSharedPointer > callback,");
_builder.newLine();
_builder.append("\t\t");
_builder.append(returnType, " ");
_builder.append(" ");
String _firstLower_1 = StringExtensions.toFirstLower(attributeName);
_builder.append(_firstLower_1, " ");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Asynchronous setter for the ");
_builder.append(attributeName, "");
_builder.append(" attribute.");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
CharSequence _printFutureParamDefinition = this.printFutureParamDefinition();
_builder.append(_printFutureParamDefinition, "");
_builder.newLineIfNotEmpty();
CharSequence _printCallbackParamDefinition_1 = this.printCallbackParamDefinition();
_builder.append(_printCallbackParamDefinition_1, "");
_builder.newLineIfNotEmpty();
_builder.append("* @param ");
String _firstLower_2 = StringExtensions.toFirstLower(returnType);
_builder.append(_firstLower_2, "");
_builder.append(" The value to set.");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual void set");
String _firstUpper_1 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_1, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("QSharedPointer > future,");
_builder.newLine();
_builder.append("\t\t");
_builder.append("QSharedPointer > callback,");
_builder.newLine();
_builder.append("\t\t");
_builder.append(returnType, " ");
_builder.append(" ");
String _firstLower_3 = StringExtensions.toFirstLower(attributeName);
_builder.append(_firstLower_3, " ");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Asynchronous setter for the ");
_builder.append(attributeName, "");
_builder.append(" attribute.");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
CharSequence _printFutureParamDefinition_1 = this.printFutureParamDefinition();
_builder.append(_printFutureParamDefinition_1, "");
_builder.newLineIfNotEmpty();
_builder.append("* @param ");
String _firstLower_4 = StringExtensions.toFirstLower(returnType);
_builder.append(_firstLower_4, "");
_builder.append(" The value to set.");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual void set");
String _firstUpper_2 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_2, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("QSharedPointer > future,");
_builder.newLine();
_builder.append("\t\t");
_builder.append(returnType, " ");
_builder.append(" ");
String _firstLower_5 = StringExtensions.toFirstLower(attributeName);
_builder.append(_firstLower_5, " ");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
}
}
return _builder;
}
public CharSequence produceSyncMethods(final FInterface serviceInterface, final boolean pure) {
StringConcatenation _builder = new StringConcatenation();
{
EList _methods = this._joynrCppGeneratorExtensions.getMethods(serviceInterface);
for(final FMethod method : _methods) {
{
Iterable _mappedOutputParameter = this._joynrCppGeneratorExtensions.getMappedOutputParameter(method);
String _head = IterableExtensions.head(_mappedOutputParameter);
boolean _equals = Objects.equal(_head, "void");
if (_equals) {
_builder.append(" ");
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Synchronous operation ");
String _joynrName = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName, "");
_builder.append(".");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
_builder.append("* @param joynrInternalStatus The joynrInternalStatus of the request which will be returned to the caller.");
_builder.newLine();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual void ");
String _joynrName_1 = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName_1, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("joynr::RequestStatus& joynrInternalStatus ");
String _commaSeperatedTypedParameterList = this._joynrCppGeneratorExtensions.getCommaSeperatedTypedParameterList(method);
String _prependCommaIfNotEmpty = this._joynrCppGeneratorExtensions.prependCommaIfNotEmpty(_commaSeperatedTypedParameterList);
_builder.append(_prependCommaIfNotEmpty, " ");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
} else {
_builder.append(" ");
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Synchronous operation ");
String _joynrName_2 = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName_2, "");
_builder.append(".");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
_builder.append("* @param joynrInternalStatus The joynrInternalStatus of the request which will be returned to the caller.");
_builder.newLine();
_builder.append("* @param result The result that will be returned to the caller.");
_builder.newLine();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual void ");
String _joynrName_3 = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName_3, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("joynr::RequestStatus& joynrInternalStatus ");
String _commaSeperatedTypedOutputParameterList = this._joynrCppGeneratorExtensions.getCommaSeperatedTypedOutputParameterList(method);
String _prependCommaIfNotEmpty_1 = this._joynrCppGeneratorExtensions.prependCommaIfNotEmpty(_commaSeperatedTypedOutputParameterList);
_builder.append(_prependCommaIfNotEmpty_1, " ");
String _commaSeperatedTypedParameterList_1 = this._joynrCppGeneratorExtensions.getCommaSeperatedTypedParameterList(method);
String _prependCommaIfNotEmpty_2 = this._joynrCppGeneratorExtensions.prependCommaIfNotEmpty(_commaSeperatedTypedParameterList_1);
_builder.append(_prependCommaIfNotEmpty_2, " ");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
}
}
}
}
return _builder;
}
public CharSequence produceAsyncMethods(final FInterface serviceInterface, final boolean pure) {
StringConcatenation _builder = new StringConcatenation();
{
EList _methods = this._joynrCppGeneratorExtensions.getMethods(serviceInterface);
for(final FMethod method : _methods) {
Iterable _mappedOutputParameter = this._joynrCppGeneratorExtensions.getMappedOutputParameter(method);
String returnType = IterableExtensions.head(_mappedOutputParameter);
_builder.append(" ");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Asynchronous operation ");
String _joynrName = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName, "");
_builder.append(".");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
CharSequence _printCallbackParamDefinition = this.printCallbackParamDefinition();
_builder.append(_printCallbackParamDefinition, "");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual void ");
String _joynrName_1 = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName_1, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("QSharedPointer > callback ");
String _commaSeperatedTypedParameterList = this._joynrCppGeneratorExtensions.getCommaSeperatedTypedParameterList(method);
String _prependCommaIfNotEmpty = this._joynrCppGeneratorExtensions.prependCommaIfNotEmpty(_commaSeperatedTypedParameterList);
_builder.append(_prependCommaIfNotEmpty, " ");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Asynchronous operation ");
String _joynrName_2 = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName_2, "");
_builder.append(".");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
CharSequence _printFutureParamDefinition = this.printFutureParamDefinition();
_builder.append(_printFutureParamDefinition, "");
_builder.newLineIfNotEmpty();
CharSequence _printCallbackParamDefinition_1 = this.printCallbackParamDefinition();
_builder.append(_printCallbackParamDefinition_1, "");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual void ");
String _joynrName_3 = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName_3, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("QSharedPointer > future,");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("QSharedPointer > callback ");
String _commaSeperatedTypedParameterList_1 = this._joynrCppGeneratorExtensions.getCommaSeperatedTypedParameterList(method);
String _prependCommaIfNotEmpty_1 = this._joynrCppGeneratorExtensions.prependCommaIfNotEmpty(_commaSeperatedTypedParameterList_1);
_builder.append(_prependCommaIfNotEmpty_1, " ");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
_builder.append("/** ");
_builder.newLine();
_builder.append("* @brief Asynchronous operation ");
String _joynrName_4 = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName_4, "");
_builder.append(".");
_builder.newLineIfNotEmpty();
CharSequence _printFutureParamDefinition_1 = this.printFutureParamDefinition();
_builder.append(_printFutureParamDefinition_1, "");
_builder.newLineIfNotEmpty();
CharSequence _printCallbackParamDefinition_2 = this.printCallbackParamDefinition();
_builder.append(_printCallbackParamDefinition_2, "");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.newLine();
_builder.append("virtual void ");
String _joynrName_5 = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName_5, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("QSharedPointer > future ");
String _commaSeperatedTypedParameterList_2 = this._joynrCppGeneratorExtensions.getCommaSeperatedTypedParameterList(method);
String _prependCommaIfNotEmpty_2 = this._joynrCppGeneratorExtensions.prependCommaIfNotEmpty(_commaSeperatedTypedParameterList_2);
_builder.append(_prependCommaIfNotEmpty_2, " ");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
}
}
return _builder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy