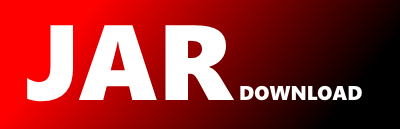
io.joynr.generator.cpp.JoynrCppGenerator Maven / Gradle / Ivy
package io.joynr.generator.cpp;
import com.google.common.base.Objects;
import com.google.common.base.Preconditions;
import com.google.common.collect.Sets;
import io.joynr.generator.IJoynrGenerator;
import io.joynr.generator.cpp.communicationmodel.CommunicationModelGenerator;
import io.joynr.generator.cpp.defaultProvider.DefaultInterfaceProviderGenerator;
import io.joynr.generator.cpp.filter.FilterGenerator;
import io.joynr.generator.cpp.inprocess.InProcessGenerator;
import io.joynr.generator.cpp.joynrmessaging.JoynrMessagingGenerator;
import io.joynr.generator.cpp.provider.ProviderGenerator;
import io.joynr.generator.cpp.proxy.ProxyGenerator;
import io.joynr.generator.util.FileSystemAccessUtil;
import io.joynr.generator.util.InvocationArguments;
import java.io.File;
import java.util.Map;
import java.util.Set;
import javax.inject.Inject;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.common.util.URI;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.emf.ecore.resource.Resource;
import org.eclipse.xtext.generator.IFileSystemAccess;
import org.franca.core.dsl.FrancaPersistenceManager;
import org.franca.core.franca.FModel;
@SuppressWarnings("all")
public class JoynrCppGenerator implements IJoynrGenerator {
@Inject
private FrancaPersistenceManager francaPersistenceManager;
@Inject
private CommunicationModelGenerator communicationModelGenerator;
@Inject
private ProxyGenerator proxyGenerator;
@Inject
private ProviderGenerator providerGenerator;
@Inject
private FilterGenerator filterGenerator;
@Inject
private InProcessGenerator inProcessGenerator;
@Inject
private JoynrMessagingGenerator joynrMessagingGenerator;
@Inject
private DefaultInterfaceProviderGenerator defaultProviderGenerator;
@Inject
private IFileSystemAccess outputHeaderFileSystem;
public final static String OUTPUT_HEADER_PATH = "outputHeaderPath";
private Map parameters;
public void doGenerate(final Resource input, final IFileSystemAccess fsa) {
IFileSystemAccess _headerFileSystemAccess = this.getHeaderFileSystemAccess(fsa);
this.doGenerate(input, fsa, _headerFileSystemAccess);
}
public String getLanguageId() {
return "cpp";
}
/**
* Triggers the generation. In case the parameter "generate" is set to false, the generator is cleaning the generation folder
*/
public void doGenerate(final Resource input, final IFileSystemAccess sourceFileSystem, final IFileSystemAccess headerFileSystem) {
final FModel fModel = this.getModel(input);
String _sourceContainerPath = this.getSourceContainerPath(sourceFileSystem, "filter");
String _headerContainerPath = this.getHeaderContainerPath(sourceFileSystem, headerFileSystem, "filter");
this.filterGenerator.doGenerate(fModel, sourceFileSystem, headerFileSystem, _sourceContainerPath, _headerContainerPath);
String _sourceContainerPath_1 = this.getSourceContainerPath(sourceFileSystem, "proxy");
String _headerContainerPath_1 = this.getHeaderContainerPath(sourceFileSystem, headerFileSystem, "proxy");
this.proxyGenerator.doGenerate(fModel, sourceFileSystem, headerFileSystem, _sourceContainerPath_1, _headerContainerPath_1);
String _sourceContainerPath_2 = this.getSourceContainerPath(sourceFileSystem, "provider");
String _headerContainerPath_2 = this.getHeaderContainerPath(sourceFileSystem, headerFileSystem, "provider");
this.providerGenerator.doGenerate(fModel, sourceFileSystem, headerFileSystem, _sourceContainerPath_2, _headerContainerPath_2);
String _sourceContainerPath_3 = this.getSourceContainerPath(sourceFileSystem, "provider");
String _headerContainerPath_3 = this.getHeaderContainerPath(sourceFileSystem, headerFileSystem, "provider");
this.defaultProviderGenerator.doGenerate(fModel, sourceFileSystem, headerFileSystem, _sourceContainerPath_3, _headerContainerPath_3);
String _sourceContainerPath_4 = this.getSourceContainerPath(sourceFileSystem, "joynr-messaging");
String _headerContainerPath_4 = this.getHeaderContainerPath(sourceFileSystem, headerFileSystem, "joynr-messaging");
this.joynrMessagingGenerator.doGenerate(fModel, sourceFileSystem, headerFileSystem, _sourceContainerPath_4, _headerContainerPath_4);
String _sourceContainerPath_5 = this.getSourceContainerPath(sourceFileSystem, "in-process");
String _headerContainerPath_5 = this.getHeaderContainerPath(sourceFileSystem, headerFileSystem, "in-process");
this.inProcessGenerator.doGenerate(fModel, sourceFileSystem, headerFileSystem, _sourceContainerPath_5, _headerContainerPath_5);
String _sourceContainerPath_6 = this.getSourceContainerPath(sourceFileSystem, "communication-model");
String _headerContainerPath_6 = this.getHeaderContainerPath(sourceFileSystem, headerFileSystem, "communication-model");
this.communicationModelGenerator.doGenerate(fModel, sourceFileSystem, headerFileSystem, _sourceContainerPath_6, _headerContainerPath_6);
}
public String getSourceContainerPath(final IFileSystemAccess sourceFileSystem, final String directory) {
return (((directory + File.separator) + "generated") + File.separator);
}
public String getHeaderContainerPath(final IFileSystemAccess sourceFileSystem, final IFileSystemAccess headerFileSystem, final String directory) {
boolean _equals = Objects.equal(sourceFileSystem, headerFileSystem);
if (_equals) {
return this.getSourceContainerPath(sourceFileSystem, directory);
} else {
return "";
}
}
public void setParameters(final Map parameter) {
this.parameters = parameter;
}
public Set supportedParameters() {
return Sets.newHashSet(JoynrCppGenerator.OUTPUT_HEADER_PATH);
}
public String getOutputHeaderPath() {
String result = null;
boolean _notEquals = (!Objects.equal(this.parameters, null));
if (_notEquals) {
String _get = this.parameters.get(JoynrCppGenerator.OUTPUT_HEADER_PATH);
boolean _notEquals_1 = (!Objects.equal(_get, null));
if (_notEquals_1) {
String _get_1 = this.parameters.get(JoynrCppGenerator.OUTPUT_HEADER_PATH);
result = _get_1;
} else {
String _get_2 = this.parameters.get(InvocationArguments.OUTPUT_PATH);
boolean _notEquals_2 = (!Objects.equal(_get_2, null));
if (_notEquals_2) {
String _get_3 = this.parameters.get(InvocationArguments.OUTPUT_PATH);
String _plus = (_get_3 + File.separator);
String _plus_1 = (_plus + "include");
result = _plus_1;
}
}
}
return result;
}
public IFileSystemAccess getHeaderFileSystemAccess(final IFileSystemAccess fsa) {
IFileSystemAccess _xblockexpression = null;
{
IFileSystemAccess headerFSA = fsa;
String _outputHeaderPath = this.getOutputHeaderPath();
boolean _notEquals = (!Objects.equal(_outputHeaderPath, null));
if (_notEquals) {
String _outputHeaderPath_1 = this.getOutputHeaderPath();
FileSystemAccessUtil.createFileSystemAccess(this.outputHeaderFileSystem, _outputHeaderPath_1);
headerFSA = this.outputHeaderFileSystem;
}
_xblockexpression = headerFSA;
}
return _xblockexpression;
}
public FModel getModel(final Resource input) {
URI _uRI = input.getURI();
String _fileExtension = _uRI.fileExtension();
String _fileExtension_1 = this.francaPersistenceManager.getFileExtension();
final boolean isFrancaIDLResource = _fileExtension.equals(_fileExtension_1);
Preconditions.checkArgument(isFrancaIDLResource, ("Unknown input: " + input));
EList _contents = input.getContents();
EObject _get = _contents.get(0);
return ((FModel) _get);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy