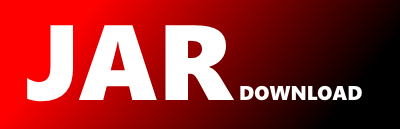
io.joynr.generator.cpp.communicationmodel.EnumHTemplate Maven / Gradle / Ivy
package io.joynr.generator.cpp.communicationmodel;
import com.google.inject.Inject;
import io.joynr.generator.cpp.util.CppStdTypeUtil;
import io.joynr.generator.cpp.util.JoynrCppGeneratorExtensions;
import io.joynr.generator.cpp.util.TemplateBase;
import io.joynr.generator.util.EnumTemplate;
import org.eclipse.emf.common.util.EList;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.StringExtensions;
import org.franca.core.franca.FEnumerationType;
import org.franca.core.franca.FEnumerator;
@SuppressWarnings("all")
public class EnumHTemplate implements EnumTemplate {
@Inject
@Extension
private TemplateBase _templateBase;
@Inject
@Extension
private JoynrCppGeneratorExtensions _joynrCppGeneratorExtensions;
@Inject
@Extension
private CppStdTypeUtil _cppStdTypeUtil;
public CharSequence generate(final FEnumerationType type) {
StringConcatenation _builder = new StringConcatenation();
final String typeName = this._joynrCppGeneratorExtensions.joynrNameQt(type);
_builder.newLineIfNotEmpty();
String _packagePathWithJoynrPrefix = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "_");
String _plus = ("GENERATED_ENUM_" + _packagePathWithJoynrPrefix);
String _plus_1 = (_plus + "_");
String _plus_2 = (_plus_1 + typeName);
String _plus_3 = (_plus_2 + "_h");
final String headerGuard = _plus_3.toUpperCase();
_builder.newLineIfNotEmpty();
CharSequence _warning = this._templateBase.warning();
_builder.append(_warning, "");
_builder.newLineIfNotEmpty();
_builder.append("#ifndef ");
_builder.append(headerGuard, "");
_builder.newLineIfNotEmpty();
_builder.append("#define ");
_builder.append(headerGuard, "");
_builder.newLineIfNotEmpty();
_builder.newLine();
String _dllExportIncludeStatement = this._joynrCppGeneratorExtensions.getDllExportIncludeStatement();
_builder.append(_dllExportIncludeStatement, "");
_builder.newLineIfNotEmpty();
_builder.append("#include ");
_builder.newLine();
_builder.append("#include ");
_builder.newLine();
_builder.append("#include \"joynr/Util.h\"");
_builder.newLine();
_builder.append("#include \"");
String _includeOfStd = this._cppStdTypeUtil.getIncludeOfStd(type);
_builder.append(_includeOfStd, "");
_builder.append("\"");
_builder.newLineIfNotEmpty();
_builder.newLine();
String _namespaceStarter = this._joynrCppGeneratorExtensions.getNamespaceStarter(type);
_builder.append(_namespaceStarter, "");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("/** @brief Enumeration wrapper class ");
_builder.append(typeName, "");
_builder.append(" */");
_builder.newLineIfNotEmpty();
_builder.append("class ");
String _dllExportMacro = this._joynrCppGeneratorExtensions.getDllExportMacro();
_builder.append(_dllExportMacro, "");
_builder.append(" ");
_builder.append(typeName, "");
_builder.append(" : public QObject {");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("Q_OBJECT");
_builder.newLine();
_builder.append("\t");
_builder.append("Q_ENUMS(");
String _nestedEnumName = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName, "\t");
_builder.append(")");
_builder.newLineIfNotEmpty();
_builder.append("public:");
_builder.newLine();
_builder.append("\t");
_builder.append("/**");
_builder.newLine();
_builder.append("\t");
CharSequence _appendDoxygenSummaryAndWriteSeeAndDescription = this._joynrCppGeneratorExtensions.appendDoxygenSummaryAndWriteSeeAndDescription(type, " *");
_builder.append(_appendDoxygenSummaryAndWriteSeeAndDescription, "\t");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.append("*/");
_builder.newLine();
_builder.append("\t");
_builder.append("enum ");
String _nestedEnumName_1 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_1, "\t");
_builder.append(" {");
_builder.newLineIfNotEmpty();
{
EList _enumElementsAndBaseEnumElements = this._joynrCppGeneratorExtensions.getEnumElementsAndBaseEnumElements(type);
boolean _hasElements = false;
for(final FEnumerator enumtype : _enumElementsAndBaseEnumElements) {
if (!_hasElements) {
_hasElements = true;
} else {
_builder.appendImmediate(",", "\t\t");
}
_builder.append("\t\t");
_builder.append("/**");
_builder.newLine();
_builder.append("\t\t");
_builder.append(" ");
_builder.append("* @brief ");
CharSequence _appendDoxygenComment = this._joynrCppGeneratorExtensions.appendDoxygenComment(enumtype, "* ");
_builder.append(_appendDoxygenComment, "\t\t ");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append(" ");
_builder.append("*/");
_builder.newLine();
_builder.append("\t\t");
String _joynrName = this._joynrCppGeneratorExtensions.joynrName(enumtype);
_builder.append(_joynrName, "\t\t");
_builder.newLineIfNotEmpty();
}
}
_builder.append("\t");
_builder.append("};");
_builder.newLine();
_builder.append("\t");
_builder.append("// Constructors required by QT metatype system");
_builder.newLine();
_builder.append("\t");
_builder.append("/** @brief Constructor */");
_builder.newLine();
_builder.append("\t");
_builder.append(typeName, "\t");
_builder.append("() : QObject() {}");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("/**");
_builder.newLine();
_builder.append("\t ");
_builder.append("* @brief Copy constructor");
_builder.newLine();
_builder.append("\t ");
_builder.append("* @param o the object to copy from");
_builder.newLine();
_builder.append("\t ");
_builder.append("*/");
_builder.newLine();
_builder.append("\t");
_builder.append(typeName, "\t");
_builder.append("(const ");
_builder.append(typeName, "\t");
_builder.append("& o) : QObject() { Q_UNUSED(o); }");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("\t");
_builder.append("/**");
_builder.newLine();
_builder.append("\t ");
_builder.append("* @brief convert standard C++ enum value to QT specific enum value");
_builder.newLine();
_builder.append("\t ");
_builder.append("* @param ");
String _joynrNameStd = this._joynrCppGeneratorExtensions.joynrNameStd(type);
String _firstLower = StringExtensions.toFirstLower(_joynrNameStd);
_builder.append(_firstLower, "\t ");
_builder.append(" the standard enum value to be converted");
_builder.newLineIfNotEmpty();
_builder.append("\t ");
_builder.append("* @return the converted QT specific enum value");
_builder.newLine();
_builder.append("\t ");
_builder.append("*/");
_builder.newLine();
_builder.append("\t");
_builder.append("static ");
_builder.append(typeName, "\t");
_builder.append("::");
String _nestedEnumName_2 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_2, "\t");
_builder.append(" createQt(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t\t");
_builder.append("const ");
{
boolean _isPartOfTypeCollection = this._joynrCppGeneratorExtensions.isPartOfTypeCollection(type);
if (_isPartOfTypeCollection) {
String _typeCollectionName = this._joynrCppGeneratorExtensions.getTypeCollectionName(type);
_builder.append(_typeCollectionName, "\t\t\t");
_builder.append("::");
}
}
String _joynrNameStd_1 = this._joynrCppGeneratorExtensions.joynrNameStd(type);
_builder.append(_joynrNameStd_1, "\t\t\t");
_builder.append("::");
String _nestedEnumName_3 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_3, "\t\t\t");
_builder.append("& ");
String _joynrNameStd_2 = this._joynrCppGeneratorExtensions.joynrNameStd(type);
String _firstLower_1 = StringExtensions.toFirstLower(_joynrNameStd_2);
_builder.append(_firstLower_1, "\t\t\t");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append(") {");
_builder.newLine();
_builder.append("\t\t");
_builder.append(typeName, "\t\t");
_builder.append("::");
String _nestedEnumName_4 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_4, "\t\t");
_builder.append(" qt");
_builder.append(typeName, "\t\t");
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("switch (");
String _joynrNameStd_3 = this._joynrCppGeneratorExtensions.joynrNameStd(type);
String _firstLower_2 = StringExtensions.toFirstLower(_joynrNameStd_3);
_builder.append(_firstLower_2, "\t\t");
_builder.append(") {");
_builder.newLineIfNotEmpty();
{
EList _enumElementsAndBaseEnumElements_1 = this._joynrCppGeneratorExtensions.getEnumElementsAndBaseEnumElements(type);
for(final FEnumerator enumtype_1 : _enumElementsAndBaseEnumElements_1) {
_builder.append("\t\t");
_builder.append("case ");
{
boolean _isPartOfTypeCollection_1 = this._joynrCppGeneratorExtensions.isPartOfTypeCollection(type);
if (_isPartOfTypeCollection_1) {
String _typeCollectionName_1 = this._joynrCppGeneratorExtensions.getTypeCollectionName(type);
_builder.append(_typeCollectionName_1, "\t\t");
_builder.append("::");
}
}
String _joynrNameStd_4 = this._joynrCppGeneratorExtensions.joynrNameStd(type);
_builder.append(_joynrNameStd_4, "\t\t");
_builder.append("::");
String _joynrName_1 = this._joynrCppGeneratorExtensions.joynrName(enumtype_1);
_builder.append(_joynrName_1, "\t\t");
_builder.append(":");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("\t");
_builder.append("qt");
_builder.append(typeName, "\t\t\t");
_builder.append(" = ");
String _joynrName_2 = this._joynrCppGeneratorExtensions.joynrName(enumtype_1);
_builder.append(_joynrName_2, "\t\t\t");
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("\t");
_builder.append("break;");
_builder.newLine();
}
}
_builder.append("\t\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t\t");
_builder.append("return qt");
_builder.append(typeName, "\t\t");
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("}");
_builder.newLine();
_builder.newLine();
_builder.append("\t");
_builder.append("/**");
_builder.newLine();
_builder.append("\t ");
_builder.append("* @brief convert QT specific enum value to standard C++ enum value");
_builder.newLine();
_builder.append("\t ");
_builder.append("* @param qt");
_builder.append(typeName, "\t ");
_builder.append(" the QT specific enum value to be converted");
_builder.newLineIfNotEmpty();
_builder.append("\t ");
_builder.append("* @return the converted standard enum value");
_builder.newLine();
_builder.append("\t ");
_builder.append("*/");
_builder.newLine();
_builder.append("\t");
_builder.append("static ");
{
boolean _isPartOfTypeCollection_2 = this._joynrCppGeneratorExtensions.isPartOfTypeCollection(type);
if (_isPartOfTypeCollection_2) {
String _typeCollectionName_2 = this._joynrCppGeneratorExtensions.getTypeCollectionName(type);
_builder.append(_typeCollectionName_2, "\t");
_builder.append("::");
}
}
String _joynrNameStd_5 = this._joynrCppGeneratorExtensions.joynrNameStd(type);
_builder.append(_joynrNameStd_5, "\t");
_builder.append("::");
String _nestedEnumName_5 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_5, "\t");
_builder.append(" createStd(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t\t");
_builder.append("const ");
_builder.append(typeName, "\t\t\t");
_builder.append("::");
String _nestedEnumName_6 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_6, "\t\t\t");
_builder.append("& qt");
_builder.append(typeName, "\t\t\t");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append(") {");
_builder.newLine();
_builder.append("\t\t");
{
boolean _isPartOfTypeCollection_3 = this._joynrCppGeneratorExtensions.isPartOfTypeCollection(type);
if (_isPartOfTypeCollection_3) {
String _typeCollectionName_3 = this._joynrCppGeneratorExtensions.getTypeCollectionName(type);
_builder.append(_typeCollectionName_3, "\t\t");
_builder.append("::");
}
}
String _joynrNameStd_6 = this._joynrCppGeneratorExtensions.joynrNameStd(type);
_builder.append(_joynrNameStd_6, "\t\t");
_builder.append("::");
String _nestedEnumName_7 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_7, "\t\t");
_builder.append(" ");
String _joynrNameStd_7 = this._joynrCppGeneratorExtensions.joynrNameStd(type);
String _firstLower_3 = StringExtensions.toFirstLower(_joynrNameStd_7);
_builder.append(_firstLower_3, "\t\t");
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("switch (qt");
_builder.append(typeName, "\t\t");
_builder.append(") {");
_builder.newLineIfNotEmpty();
{
EList _enumElementsAndBaseEnumElements_2 = this._joynrCppGeneratorExtensions.getEnumElementsAndBaseEnumElements(type);
for(final FEnumerator enumtype_2 : _enumElementsAndBaseEnumElements_2) {
_builder.append("\t\t");
_builder.append("case ");
String _joynrName_3 = this._joynrCppGeneratorExtensions.joynrName(enumtype_2);
_builder.append(_joynrName_3, "\t\t");
_builder.append(":");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("\t");
String _joynrNameStd_8 = this._joynrCppGeneratorExtensions.joynrNameStd(type);
String _firstLower_4 = StringExtensions.toFirstLower(_joynrNameStd_8);
_builder.append(_firstLower_4, "\t\t\t");
_builder.append(" = ");
{
boolean _isPartOfTypeCollection_4 = this._joynrCppGeneratorExtensions.isPartOfTypeCollection(type);
if (_isPartOfTypeCollection_4) {
String _typeCollectionName_4 = this._joynrCppGeneratorExtensions.getTypeCollectionName(type);
_builder.append(_typeCollectionName_4, "\t\t\t");
_builder.append("::");
}
}
String _joynrNameStd_9 = this._joynrCppGeneratorExtensions.joynrNameStd(type);
_builder.append(_joynrNameStd_9, "\t\t\t");
_builder.append("::");
String _joynrName_4 = this._joynrCppGeneratorExtensions.joynrName(enumtype_2);
_builder.append(_joynrName_4, "\t\t\t");
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("\t");
_builder.append("break;");
_builder.newLine();
}
}
_builder.append("\t\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t\t");
_builder.append("return ");
String _joynrNameStd_10 = this._joynrCppGeneratorExtensions.joynrNameStd(type);
String _firstLower_5 = StringExtensions.toFirstLower(_joynrNameStd_10);
_builder.append(_firstLower_5, "\t\t");
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("}");
_builder.newLine();
_builder.append("};");
_builder.newLine();
_builder.newLine();
String _namespaceEnder = this._joynrCppGeneratorExtensions.getNamespaceEnder(type);
_builder.append(_namespaceEnder, "");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("namespace joynr {");
_builder.newLine();
_builder.append("template <>");
_builder.newLine();
_builder.append("inline ");
String _packagePathWithJoynrPrefix_1 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "::");
_builder.append(_packagePathWithJoynrPrefix_1, "");
_builder.append("::");
_builder.append(typeName, "");
_builder.append("::");
String _nestedEnumName_8 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_8, "");
_builder.append(" joynr::Util::valueOf<");
String _packagePathWithJoynrPrefix_2 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "::");
_builder.append(_packagePathWithJoynrPrefix_2, "");
_builder.append("::");
_builder.append(typeName, "");
_builder.append("::");
String _nestedEnumName_9 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_9, "");
_builder.append(">(const QVariant& variant)");
_builder.newLineIfNotEmpty();
_builder.append("{");
_builder.newLine();
_builder.append(" ");
_builder.append("return ");
String _joynrGenerationPrefix = this._joynrCppGeneratorExtensions.getJoynrGenerationPrefix();
_builder.append(_joynrGenerationPrefix, " ");
_builder.append("::Util::convertVariantToEnum<");
String _packagePathWithJoynrPrefix_3 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "::");
_builder.append(_packagePathWithJoynrPrefix_3, " ");
_builder.append("::");
_builder.append(typeName, " ");
_builder.append(">(variant);");
_builder.newLineIfNotEmpty();
_builder.append("}");
_builder.newLine();
_builder.newLine();
_builder.append("template <>");
_builder.newLine();
_builder.append("inline QList<");
String _packagePathWithJoynrPrefix_4 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "::");
_builder.append(_packagePathWithJoynrPrefix_4, "");
_builder.append("::");
_builder.append(typeName, "");
_builder.append("::Enum> joynr::Util::valueOf>(const QVariant& variant){");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.append("return ");
String _joynrGenerationPrefix_1 = this._joynrCppGeneratorExtensions.getJoynrGenerationPrefix();
_builder.append(_joynrGenerationPrefix_1, " ");
_builder.append("::Util::convertVariantListToEnumList<");
String _packagePathWithJoynrPrefix_6 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "::");
_builder.append(_packagePathWithJoynrPrefix_6, " ");
_builder.append("::");
_builder.append(typeName, " ");
_builder.append(">(variant.value());");
_builder.newLineIfNotEmpty();
_builder.append("}");
_builder.newLine();
_builder.append("}");
_builder.newLine();
_builder.append("// Metatype for the wrapper class");
_builder.newLine();
_builder.append("typedef ");
String _packagePathWithJoynrPrefix_7 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "::");
_builder.append(_packagePathWithJoynrPrefix_7, "");
_builder.append("::");
_builder.append(typeName, "");
_builder.append(" ");
String _packagePathWithJoynrPrefix_8 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "__");
_builder.append(_packagePathWithJoynrPrefix_8, "");
_builder.append("__");
_builder.append(typeName, "");
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append("Q_DECLARE_METATYPE(");
String _packagePathWithJoynrPrefix_9 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "__");
_builder.append(_packagePathWithJoynrPrefix_9, "");
_builder.append("__");
_builder.append(typeName, "");
_builder.append(")");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("// Metatypes for the ");
String _nestedEnumName_10 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_10, "");
_builder.newLineIfNotEmpty();
_builder.append("typedef ");
String _packagePathWithJoynrPrefix_10 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "::");
_builder.append(_packagePathWithJoynrPrefix_10, "");
_builder.append("::");
_builder.append(typeName, "");
_builder.append("::");
String _nestedEnumName_11 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_11, "");
_builder.append(" ");
String _packagePathWithJoynrPrefix_11 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "__");
_builder.append(_packagePathWithJoynrPrefix_11, "");
_builder.append("__");
_builder.append(typeName, "");
_builder.append("__");
String _nestedEnumName_12 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_12, "");
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.append("Q_DECLARE_METATYPE(");
String _packagePathWithJoynrPrefix_12 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "__");
_builder.append(_packagePathWithJoynrPrefix_12, "");
_builder.append("__");
_builder.append(typeName, "");
_builder.append("__");
String _nestedEnumName_13 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_13, "");
_builder.append(")");
_builder.newLineIfNotEmpty();
_builder.append("Q_DECLARE_METATYPE(QList<");
String _packagePathWithJoynrPrefix_13 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "__");
_builder.append(_packagePathWithJoynrPrefix_13, "");
_builder.append("__");
_builder.append(typeName, "");
_builder.append("__");
String _nestedEnumName_14 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_14, "");
_builder.append(">)");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("inline uint qHash(");
String _packagePathWithJoynrPrefix_14 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(type, "::");
_builder.append(_packagePathWithJoynrPrefix_14, "");
_builder.append("::");
_builder.append(typeName, "");
_builder.append("::");
String _nestedEnumName_15 = this._joynrCppGeneratorExtensions.getNestedEnumName();
_builder.append(_nestedEnumName_15, "");
_builder.append(" key, uint seed = 0) {");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("return uint(key) ^ seed;");
_builder.newLine();
_builder.append("}");
_builder.newLine();
_builder.newLine();
_builder.append("#endif // ");
_builder.append(headerGuard, "");
_builder.newLineIfNotEmpty();
return _builder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy