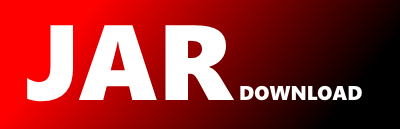
io.joynr.generator.cpp.util.InterfaceUtil Maven / Gradle / Ivy
package io.joynr.generator.cpp.util;
import com.google.inject.Inject;
import io.joynr.generator.cpp.util.CppStdTypeUtil;
import io.joynr.generator.cpp.util.JoynrCppGeneratorExtensions;
import org.eclipse.emf.common.util.EList;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.StringExtensions;
import org.franca.core.franca.FArgument;
import org.franca.core.franca.FAttribute;
import org.franca.core.franca.FInterface;
import org.franca.core.franca.FMethod;
@SuppressWarnings("all")
public class InterfaceUtil {
@Inject
@Extension
private JoynrCppGeneratorExtensions _joynrCppGeneratorExtensions;
@Inject
@Extension
private CppStdTypeUtil _cppStdTypeUtil;
public CharSequence printFutureReturnDefinition() {
StringConcatenation _builder = new StringConcatenation();
_builder.append("* @returns A future representing the result of the asynchronous method call. It provides methods");
_builder.newLine();
_builder.append("* to wait for completion, to get the result or the request status object.");
_builder.newLine();
return _builder;
}
public CharSequence printOnSuccessFctParamDefinition() {
StringConcatenation _builder = new StringConcatenation();
_builder.append("* @param onSuccess A callback function to be called once the asynchronous computation has");
_builder.newLine();
_builder.append("* finished successfully. It must expect the method out parameters.");
_builder.newLine();
return _builder;
}
public CharSequence printOnErrorFctParamDefinition() {
StringConcatenation _builder = new StringConcatenation();
_builder.append("* @param onError A callback function to be called once the asynchronous computation has");
_builder.newLine();
_builder.append("* failed. It must expect the request status object.");
_builder.newLine();
return _builder;
}
public CharSequence produceSyncGetters(final FInterface serviceInterface, final boolean pure) {
StringConcatenation _builder = new StringConcatenation();
{
EList _attributes = this._joynrCppGeneratorExtensions.getAttributes(serviceInterface);
final Function1 _function = new Function1() {
public Boolean apply(final FAttribute attribute) {
return Boolean.valueOf(InterfaceUtil.this._joynrCppGeneratorExtensions.isReadable(attribute));
}
};
Iterable _filter = IterableExtensions.filter(_attributes, _function);
for(final FAttribute attribute : _filter) {
final String returnType = this._cppStdTypeUtil.getTypeName(attribute);
_builder.newLineIfNotEmpty();
final String attributeName = this._joynrCppGeneratorExtensions.joynrName(attribute);
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Synchronous getter for the ");
_builder.append(attributeName, "");
_builder.append(" attribute.");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
_builder.append("* @param result The result that will be returned to the caller.");
_builder.newLine();
_builder.append("* @returns the RequestStatus of the get");
String _firstUpper = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper, "");
_builder.append(" call");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual joynr::RequestStatus get");
String _firstUpper_1 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_1, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append(returnType, "\t\t");
_builder.append("& result");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
_builder.newLine();
}
}
return _builder;
}
public CharSequence produceAsyncGetters(final FInterface serviceInterface, final boolean pure) {
StringConcatenation _builder = new StringConcatenation();
{
EList _attributes = this._joynrCppGeneratorExtensions.getAttributes(serviceInterface);
final Function1 _function = new Function1() {
public Boolean apply(final FAttribute attribute) {
return Boolean.valueOf(InterfaceUtil.this._joynrCppGeneratorExtensions.isReadable(attribute));
}
};
Iterable _filter = IterableExtensions.filter(_attributes, _function);
for(final FAttribute attribute : _filter) {
final String returnType = this._cppStdTypeUtil.getTypeName(attribute);
_builder.newLineIfNotEmpty();
final String attributeName = this._joynrCppGeneratorExtensions.joynrName(attribute);
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Asynchronous getter for the ");
_builder.append(attributeName, "");
_builder.append(" attribute.");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
CharSequence _printOnSuccessFctParamDefinition = this.printOnSuccessFctParamDefinition();
_builder.append(_printOnSuccessFctParamDefinition, "");
_builder.newLineIfNotEmpty();
CharSequence _printOnErrorFctParamDefinition = this.printOnErrorFctParamDefinition();
_builder.append(_printOnErrorFctParamDefinition, "");
_builder.newLineIfNotEmpty();
CharSequence _printFutureReturnDefinition = this.printFutureReturnDefinition();
_builder.append(_printFutureReturnDefinition, "");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual std::shared_ptr > get");
String _firstUpper = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper, "");
_builder.append("Async(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("std::function onSuccess = nullptr,");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("std::function onError = nullptr");
_builder.newLine();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
}
}
return _builder;
}
public CharSequence produceSyncSetters(final FInterface serviceInterface, final boolean pure) {
StringConcatenation _builder = new StringConcatenation();
{
EList _attributes = this._joynrCppGeneratorExtensions.getAttributes(serviceInterface);
final Function1 _function = new Function1() {
public Boolean apply(final FAttribute attribute) {
return Boolean.valueOf(InterfaceUtil.this._joynrCppGeneratorExtensions.isWritable(attribute));
}
};
Iterable _filter = IterableExtensions.filter(_attributes, _function);
for(final FAttribute attribute : _filter) {
final String returnType = this._cppStdTypeUtil.getTypeName(attribute);
_builder.newLineIfNotEmpty();
final String attributeName = this._joynrCppGeneratorExtensions.joynrName(attribute);
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Synchronous setter for the ");
_builder.append(attributeName, "");
_builder.append(" attribute.");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
_builder.append("* @param ");
String _firstLower = StringExtensions.toFirstLower(attributeName);
_builder.append(_firstLower, "");
_builder.append(" The value to set.");
_builder.newLineIfNotEmpty();
_builder.append("* @return the RequestStatus of the set");
String _firstUpper = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper, "");
_builder.append(" call");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual joynr::RequestStatus set");
String _firstUpper_1 = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper_1, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("const ");
_builder.append(returnType, "\t\t");
_builder.append("& ");
String _firstLower_1 = StringExtensions.toFirstLower(attributeName);
_builder.append(_firstLower_1, "\t\t");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
}
}
return _builder;
}
public CharSequence produceAsyncSetters(final FInterface serviceInterface, final boolean pure) {
StringConcatenation _builder = new StringConcatenation();
{
EList _attributes = this._joynrCppGeneratorExtensions.getAttributes(serviceInterface);
final Function1 _function = new Function1() {
public Boolean apply(final FAttribute attribute) {
return Boolean.valueOf(InterfaceUtil.this._joynrCppGeneratorExtensions.isWritable(attribute));
}
};
Iterable _filter = IterableExtensions.filter(_attributes, _function);
for(final FAttribute attribute : _filter) {
final String returnType = this._cppStdTypeUtil.getTypeName(attribute);
_builder.newLineIfNotEmpty();
final String attributeName = this._joynrCppGeneratorExtensions.joynrName(attribute);
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Asynchronous setter for the ");
_builder.append(attributeName, "");
_builder.append(" attribute.");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
_builder.append("* @param ");
String _firstLower = StringExtensions.toFirstLower(attributeName);
_builder.append(_firstLower, "");
_builder.append(" The value to set.");
_builder.newLineIfNotEmpty();
CharSequence _printOnSuccessFctParamDefinition = this.printOnSuccessFctParamDefinition();
_builder.append(_printOnSuccessFctParamDefinition, "");
_builder.newLineIfNotEmpty();
CharSequence _printOnErrorFctParamDefinition = this.printOnErrorFctParamDefinition();
_builder.append(_printOnErrorFctParamDefinition, "");
_builder.newLineIfNotEmpty();
CharSequence _printFutureReturnDefinition = this.printFutureReturnDefinition();
_builder.append(_printFutureReturnDefinition, "");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual std::shared_ptr > set");
String _firstUpper = StringExtensions.toFirstUpper(attributeName);
_builder.append(_firstUpper, "");
_builder.append("Async(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append(returnType, "\t\t");
_builder.append(" ");
String _firstLower_1 = StringExtensions.toFirstLower(attributeName);
_builder.append(_firstLower_1, "\t\t");
_builder.append(",");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("std::function onSuccess = nullptr,");
_builder.newLine();
_builder.append("\t\t");
_builder.append("std::function onError = nullptr");
_builder.newLine();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
}
}
return _builder;
}
public CharSequence produceSyncMethods(final FInterface serviceInterface, final boolean pure) {
StringConcatenation _builder = new StringConcatenation();
{
EList _methods = this._joynrCppGeneratorExtensions.getMethods(serviceInterface);
for(final FMethod method : _methods) {
final String outputTypedParamList = this._cppStdTypeUtil.getCommaSeperatedTypedOutputParameterList(method);
_builder.newLineIfNotEmpty();
final String inputTypedParamList = this._cppStdTypeUtil.getCommaSeperatedTypedConstInputParameterList(method);
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Synchronous operation ");
String _joynrName = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName, "");
_builder.append(".");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
{
Iterable _outputParameters = this._joynrCppGeneratorExtensions.getOutputParameters(method);
for(final FArgument outputParam : _outputParameters) {
_builder.append("* @param ");
String _typeName = this._cppStdTypeUtil.getTypeName(outputParam);
_builder.append(_typeName, "");
_builder.append(" ");
String _joynrName_1 = this._joynrCppGeneratorExtensions.joynrName(outputParam);
_builder.append(_joynrName_1, "");
_builder.append(" this is an output parameter");
_builder.newLineIfNotEmpty();
_builder.append("* and will be set within function ");
String _joynrName_2 = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName_2, "");
_builder.newLineIfNotEmpty();
}
}
{
Iterable _inputParameters = this._joynrCppGeneratorExtensions.getInputParameters(method);
for(final FArgument inputParam : _inputParameters) {
_builder.append("* @param ");
String _typeName_1 = this._cppStdTypeUtil.getTypeName(inputParam);
_builder.append(_typeName_1, "");
_builder.append(" ");
String _joynrName_3 = this._joynrCppGeneratorExtensions.joynrName(inputParam);
_builder.append(_joynrName_3, "");
_builder.newLineIfNotEmpty();
}
}
_builder.append("* @return the internal status of the request which will be returned to the caller.");
_builder.newLine();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual joynr::RequestStatus ");
String _joynrName_4 = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName_4, "");
_builder.append("(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append(outputTypedParamList, "\t\t");
{
boolean _and = false;
Iterable _outputParameters_1 = this._joynrCppGeneratorExtensions.getOutputParameters(method);
int _size = IterableExtensions.size(_outputParameters_1);
boolean _greaterThan = (_size > 0);
if (!_greaterThan) {
_and = false;
} else {
Iterable _inputParameters_1 = this._joynrCppGeneratorExtensions.getInputParameters(method);
int _size_1 = IterableExtensions.size(_inputParameters_1);
boolean _greaterThan_1 = (_size_1 > 0);
_and = _greaterThan_1;
}
if (_and) {
_builder.append(", ");
}
}
_builder.append(inputTypedParamList, "\t\t");
_builder.newLineIfNotEmpty();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
}
}
return _builder;
}
public CharSequence produceAsyncMethods(final FInterface serviceInterface, final boolean pure) {
StringConcatenation _builder = new StringConcatenation();
{
EList _methods = this._joynrCppGeneratorExtensions.getMethods(serviceInterface);
for(final FMethod method : _methods) {
String outputParameters = this._cppStdTypeUtil.getCommaSeparatedOutputParameterTypes(method);
_builder.newLineIfNotEmpty();
final String outputTypedParamList = this._cppStdTypeUtil.getCommaSeperatedTypedConstOutputParameterList(method);
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("/**");
_builder.newLine();
_builder.append("* @brief Asynchronous operation ");
String _joynrName = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName, "");
_builder.append(".");
_builder.newLineIfNotEmpty();
_builder.append("*");
_builder.newLine();
CharSequence _printOnSuccessFctParamDefinition = this.printOnSuccessFctParamDefinition();
_builder.append(_printOnSuccessFctParamDefinition, "");
_builder.newLineIfNotEmpty();
CharSequence _printOnErrorFctParamDefinition = this.printOnErrorFctParamDefinition();
_builder.append(_printOnErrorFctParamDefinition, "");
_builder.newLineIfNotEmpty();
CharSequence _printFutureReturnDefinition = this.printFutureReturnDefinition();
_builder.append(_printFutureReturnDefinition, "");
_builder.newLineIfNotEmpty();
_builder.append("*/");
_builder.newLine();
_builder.append("virtual std::shared_ptr > ");
String _joynrName_1 = this._joynrCppGeneratorExtensions.joynrName(method);
_builder.append(_joynrName_1, "");
_builder.append("Async(");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
String _commaSeperatedTypedConstInputParameterList = this._cppStdTypeUtil.getCommaSeperatedTypedConstInputParameterList(method);
_builder.append(_commaSeperatedTypedConstInputParameterList, "\t\t");
{
Iterable _inputParameters = this._joynrCppGeneratorExtensions.getInputParameters(method);
boolean _isEmpty = IterableExtensions.isEmpty(_inputParameters);
boolean _not = (!_isEmpty);
if (_not) {
_builder.append(",");
}
}
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("std::function onSuccess = nullptr,");
_builder.newLineIfNotEmpty();
_builder.append("\t\t");
_builder.append("std::function onError = nullptr");
_builder.newLine();
_builder.append(")");
{
if (pure) {
_builder.append("=0");
}
}
_builder.append(";");
_builder.newLineIfNotEmpty();
}
}
return _builder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy