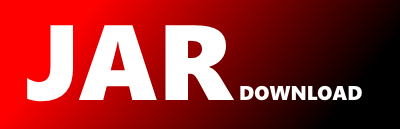
io.joynr.generator.cpp.proxy.IInterfaceConnectorHTemplate Maven / Gradle / Ivy
package io.joynr.generator.cpp.proxy;
import com.google.inject.Inject;
import io.joynr.generator.cpp.util.CppStdTypeUtil;
import io.joynr.generator.cpp.util.DatatypeSystemTransformation;
import io.joynr.generator.cpp.util.InterfaceSubscriptionUtil;
import io.joynr.generator.cpp.util.JoynrCppGeneratorExtensions;
import io.joynr.generator.cpp.util.QtTypeUtil;
import io.joynr.generator.cpp.util.TemplateBase;
import io.joynr.generator.util.InterfaceTemplate;
import java.util.Set;
import org.eclipse.emf.common.util.EList;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.StringExtensions;
import org.franca.core.franca.FArgument;
import org.franca.core.franca.FAttribute;
import org.franca.core.franca.FBroadcast;
import org.franca.core.franca.FInterface;
@SuppressWarnings("all")
public class IInterfaceConnectorHTemplate implements InterfaceTemplate {
@Inject
@Extension
private JoynrCppGeneratorExtensions _joynrCppGeneratorExtensions;
@Inject
@Extension
private TemplateBase _templateBase;
@Inject
private CppStdTypeUtil cppStdTypeUtil;
@Inject
@Extension
private QtTypeUtil qtTypeUtil;
@Inject
@Extension
private InterfaceSubscriptionUtil _interfaceSubscriptionUtil;
public CharSequence generate(final FInterface serviceInterface) {
StringConcatenation _builder = new StringConcatenation();
final String interfaceName = this._joynrCppGeneratorExtensions.joynrName(serviceInterface);
_builder.newLineIfNotEmpty();
String _packagePathWithJoynrPrefix = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(serviceInterface, "_");
String _plus = ("GENERATED_INTERFACE_" + _packagePathWithJoynrPrefix);
String _plus_1 = (_plus +
"_I");
String _plus_2 = (_plus_1 + interfaceName);
String _plus_3 = (_plus_2 + "Connector_h");
final String headerGuard = _plus_3.toUpperCase();
_builder.newLineIfNotEmpty();
CharSequence _warning = this._templateBase.warning();
_builder.append(_warning, "");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("#ifndef ");
_builder.append(headerGuard, "");
_builder.newLineIfNotEmpty();
_builder.append("#define ");
_builder.append(headerGuard, "");
_builder.newLineIfNotEmpty();
_builder.newLine();
String _dllExportIncludeStatement = this._joynrCppGeneratorExtensions.getDllExportIncludeStatement();
_builder.append(_dllExportIncludeStatement, "");
_builder.newLineIfNotEmpty();
{
Set _requiredIncludesFor = this.cppStdTypeUtil.getRequiredIncludesFor(serviceInterface);
for(final String parameterType : _requiredIncludesFor) {
_builder.append("#include ");
_builder.append(parameterType, "");
_builder.newLineIfNotEmpty();
}
}
{
Set _requiredIncludesFor_1 = this.qtTypeUtil.getRequiredIncludesFor(serviceInterface);
for(final String parameterType_1 : _requiredIncludesFor_1) {
_builder.append("#include ");
_builder.append(parameterType_1, "");
_builder.newLineIfNotEmpty();
}
}
_builder.newLine();
_builder.append("#include \"");
String _packagePathWithJoynrPrefix_1 = this._joynrCppGeneratorExtensions.getPackagePathWithJoynrPrefix(serviceInterface, "/");
_builder.append(_packagePathWithJoynrPrefix_1, "");
_builder.append("/I");
_builder.append(interfaceName, "");
_builder.append(".h\"");
_builder.newLineIfNotEmpty();
_builder.append("#include \"joynr/ISubscriptionListener.h\"");
_builder.newLine();
_builder.append("#include \"joynr/SubscriptionCallback.h\"");
_builder.newLine();
_builder.append("#include \"joynr/IConnector.h\"");
_builder.newLine();
_builder.append("#include \"joynr/TypeUtil.h\"");
_builder.newLine();
_builder.append("#include ");
_builder.newLine();
_builder.newLine();
_builder.append("namespace joynr {");
_builder.newLine();
_builder.append("\t");
_builder.append("template class ISubscriptionListener;");
_builder.newLine();
_builder.append("\t");
_builder.append("class ISubscriptionCallback;");
_builder.newLine();
_builder.append("\t");
_builder.append("class SubscriptionQos;");
_builder.newLine();
_builder.append("\t");
_builder.append("class OnChangeSubscriptionQos;");
_builder.newLine();
_builder.append("}");
_builder.newLine();
_builder.newLine();
String _namespaceStarter = this._joynrCppGeneratorExtensions.getNamespaceStarter(serviceInterface);
_builder.append(_namespaceStarter, "");
_builder.newLineIfNotEmpty();
_builder.append("class ");
String _dllExportMacro = this._joynrCppGeneratorExtensions.getDllExportMacro();
_builder.append(_dllExportMacro, "");
_builder.append(" I");
_builder.append(interfaceName, "");
_builder.append("Subscription{");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("/**");
_builder.newLine();
_builder.append("\t ");
_builder.append("* in - subscriptionListener std::shared_ptr to a SubscriptionListener which will receive the updates.");
_builder.newLine();
_builder.append("\t ");
_builder.append("* in - subscriptionQos SubscriptionQos parameters like interval and end date.");
_builder.newLine();
_builder.append("\t ");
_builder.append("* out - assignedSubscriptionId Buffer for the assigned subscriptionId.");
_builder.newLine();
_builder.append("\t ");
_builder.append("*/");
_builder.newLine();
_builder.append("public:");
_builder.newLine();
_builder.append("\t");
_builder.append("virtual ~I");
_builder.append(interfaceName, "\t");
_builder.append("Subscription() {}");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("\t");
CharSequence _produceSubscribeUnsubscribeMethods = this._interfaceSubscriptionUtil.produceSubscribeUnsubscribeMethods(serviceInterface, true);
_builder.append(_produceSubscribeUnsubscribeMethods, "\t");
_builder.newLineIfNotEmpty();
_builder.append("};");
_builder.newLine();
_builder.newLine();
_builder.append("class ");
String _dllExportMacro_1 = this._joynrCppGeneratorExtensions.getDllExportMacro();
_builder.append(_dllExportMacro_1, "");
_builder.append(" I");
_builder.append(interfaceName, "");
_builder.append("Connector: virtual public I");
_builder.append(interfaceName, "");
_builder.append(", public joynr::IConnector, virtual public I");
_builder.append(interfaceName, "");
_builder.append("Subscription{");
_builder.newLineIfNotEmpty();
_builder.newLine();
_builder.append("public:");
_builder.newLine();
_builder.append("\t");
_builder.append("virtual ~I");
_builder.append(interfaceName, "\t");
_builder.append("Connector() {}");
_builder.newLineIfNotEmpty();
_builder.append("protected:");
_builder.newLine();
{
EList _attributes = this._joynrCppGeneratorExtensions.getAttributes(serviceInterface);
final Function1 _function = new Function1() {
public Boolean apply(final FAttribute attribute) {
return Boolean.valueOf(IInterfaceConnectorHTemplate.this._joynrCppGeneratorExtensions.isNotifiable(attribute));
}
};
Iterable _filter = IterableExtensions.filter(_attributes, _function);
for(final FAttribute attribute : _filter) {
_builder.append("\t");
final String returnType = this.cppStdTypeUtil.getTypeName(attribute);
_builder.newLineIfNotEmpty();
_builder.append("\t");
final String returnTypeQT = this.qtTypeUtil.getTypeName(attribute);
_builder.newLineIfNotEmpty();
{
boolean _needsDatatypeConversion = this.qtTypeUtil.needsDatatypeConversion(attribute);
if (_needsDatatypeConversion) {
_builder.append("\t");
_builder.append("class ");
String _joynrName = this._joynrCppGeneratorExtensions.joynrName(attribute);
String _firstUpper = StringExtensions.toFirstUpper(_joynrName);
_builder.append(_firstUpper, "\t");
_builder.append("AttributeSubscriptionListenerWrapper : public ISubscriptionListener<");
_builder.append(returnTypeQT, "\t");
_builder.append("> {");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t");
_builder.append("public:");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
String _joynrName_1 = this._joynrCppGeneratorExtensions.joynrName(attribute);
String _firstUpper_1 = StringExtensions.toFirstUpper(_joynrName_1);
_builder.append(_firstUpper_1, "\t\t\t");
_builder.append("AttributeSubscriptionListenerWrapper(");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t\t\t");
_builder.append("std::shared_ptr> wrappedListener");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t");
_builder.append(") :");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t\t");
_builder.append("wrappedListener(wrappedListener)");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("{");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("void onReceive(const ");
_builder.append(returnTypeQT, "\t\t\t");
_builder.append("& receivedValue) {");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t\t");
_builder.append("wrappedListener->onReceive(");
String _fromQTTypeToStdType = this.qtTypeUtil.fromQTTypeToStdType(attribute, "receivedValue");
_builder.append(_fromQTTypeToStdType, "\t\t\t\t");
_builder.append(");");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("void onError() {");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t");
_builder.append("wrappedListener->onError();");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("}");
_builder.newLine();
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("private:");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("std::shared_ptr> wrappedListener;");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("};");
_builder.newLine();
_builder.newLine();
_builder.append("\t");
_builder.append("class ");
String _joynrName_2 = this._joynrCppGeneratorExtensions.joynrName(attribute);
String _firstUpper_2 = StringExtensions.toFirstUpper(_joynrName_2);
_builder.append(_firstUpper_2, "\t");
_builder.append("AttributeSubscriptionCallbackWrapper : public SubscriptionCallback<");
_builder.append(returnTypeQT, "\t");
_builder.append("> {");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("public:");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
String _joynrName_3 = this._joynrCppGeneratorExtensions.joynrName(attribute);
String _firstUpper_3 = StringExtensions.toFirstUpper(_joynrName_3);
_builder.append(_firstUpper_3, "\t\t");
_builder.append("AttributeSubscriptionCallbackWrapper(");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t\t");
_builder.append("std::shared_ptr> wrappedListener");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t");
_builder.append(") :");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t");
_builder.append("SubscriptionCallback(");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t\t\t");
_builder.append("std::shared_ptr>(");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t\t\t\t\t\t");
_builder.append("new ");
String _joynrName_4 = this._joynrCppGeneratorExtensions.joynrName(attribute);
String _firstUpper_4 = StringExtensions.toFirstUpper(_joynrName_4);
_builder.append(_firstUpper_4, "\t\t\t\t\t\t\t\t");
_builder.append("AttributeSubscriptionListenerWrapper(wrappedListener))");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t\t\t\t");
_builder.append(")");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("{");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("virtual void onSuccess(const ");
_builder.append(returnTypeQT, "\t\t");
_builder.append("& receivedValue) {");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("std::shared_ptr<");
String _joynrName_5 = this._joynrCppGeneratorExtensions.joynrName(attribute);
String _firstUpper_5 = StringExtensions.toFirstUpper(_joynrName_5);
_builder.append(_firstUpper_5, "\t\t\t");
_builder.append("AttributeSubscriptionListenerWrapper> wrapper =");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t\t");
_builder.append("std::dynamic_pointer_cast<");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t\t\t");
String _joynrName_6 = this._joynrCppGeneratorExtensions.joynrName(attribute);
String _firstUpper_6 = StringExtensions.toFirstUpper(_joynrName_6);
_builder.append(_firstUpper_6, "\t\t\t\t\t\t");
_builder.append("AttributeSubscriptionListenerWrapper>(listener);");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("wrapper->onReceive(receivedValue);");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("virtual void onError() {");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("std::shared_ptr<");
String _joynrName_7 = this._joynrCppGeneratorExtensions.joynrName(attribute);
String _firstUpper_7 = StringExtensions.toFirstUpper(_joynrName_7);
_builder.append(_firstUpper_7, "\t\t\t");
_builder.append("AttributeSubscriptionListenerWrapper> wrapper =");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t\t");
_builder.append("std::dynamic_pointer_cast<");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t\t\t");
String _joynrName_8 = this._joynrCppGeneratorExtensions.joynrName(attribute);
String _firstUpper_8 = StringExtensions.toFirstUpper(_joynrName_8);
_builder.append(_firstUpper_8, "\t\t\t\t\t\t");
_builder.append("AttributeSubscriptionListenerWrapper>(listener);");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("wrapper->onError();");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t");
_builder.append("};");
_builder.newLine();
}
}
}
}
{
EList _broadcasts = serviceInterface.getBroadcasts();
for(final FBroadcast broadcast : _broadcasts) {
{
boolean _needsDatatypeConversion_1 = this.qtTypeUtil.needsDatatypeConversion(broadcast);
if (_needsDatatypeConversion_1) {
_builder.append("\t");
final String returnTypesQT = this.qtTypeUtil.getCommaSeparatedOutputParameterTypes(broadcast);
_builder.newLineIfNotEmpty();
_builder.append("\t");
final String returnTypesStd = this.cppStdTypeUtil.getCommaSeparatedOutputParameterTypes(broadcast);
_builder.newLineIfNotEmpty();
_builder.append("\t");
final String outputParametersSignature = this.qtTypeUtil.getCommaSeperatedTypedConstOutputParameterList(broadcast);
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("class ");
String _joynrName_9 = this._joynrCppGeneratorExtensions.joynrName(broadcast);
String _firstUpper_9 = StringExtensions.toFirstUpper(_joynrName_9);
_builder.append(_firstUpper_9, "\t");
_builder.append("BroadcastSubscriptionListenerWrapper : public ISubscriptionListener<");
_builder.append(returnTypesQT, "\t");
_builder.append("> {");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t");
_builder.append("public:");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
String _joynrName_10 = this._joynrCppGeneratorExtensions.joynrName(broadcast);
String _firstUpper_10 = StringExtensions.toFirstUpper(_joynrName_10);
_builder.append(_firstUpper_10, "\t\t\t");
_builder.append("BroadcastSubscriptionListenerWrapper(");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t\t\t");
_builder.append("std::shared_ptr> wrappedListener");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t");
_builder.append(") :");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t\t");
_builder.append("wrappedListener(wrappedListener)");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("{");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("void onReceive(");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t\t");
_builder.append(outputParametersSignature, "\t\t\t\t\t");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t");
_builder.append(") {");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t");
_builder.append("wrappedListener->onReceive(");
String _commaSeperatedUntypedOutputParameterList = this.qtTypeUtil.getCommaSeperatedUntypedOutputParameterList(broadcast, DatatypeSystemTransformation.FROM_QT_TO_STANDARD);
_builder.append(_commaSeperatedUntypedOutputParameterList, "\t\t\t\t");
_builder.append(");");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("void onError() {");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t");
_builder.append("wrappedListener->onError();");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("}");
_builder.newLine();
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("private:");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("std::shared_ptr> wrappedListener;");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("};");
_builder.newLine();
_builder.newLine();
_builder.append("\t");
_builder.append("class ");
String _joynrName_11 = this._joynrCppGeneratorExtensions.joynrName(broadcast);
String _firstUpper_11 = StringExtensions.toFirstUpper(_joynrName_11);
_builder.append(_firstUpper_11, "\t");
_builder.append("BroadcastSubscriptionCallbackWrapper");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t");
_builder.append(": public SubscriptionCallback<");
_builder.append(returnTypesQT, "\t\t\t");
_builder.append(">");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("{");
_builder.newLine();
_builder.append("\t");
_builder.append("public:");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
String _joynrName_12 = this._joynrCppGeneratorExtensions.joynrName(broadcast);
String _firstUpper_12 = StringExtensions.toFirstUpper(_joynrName_12);
_builder.append(_firstUpper_12, "\t\t");
_builder.append("BroadcastSubscriptionCallbackWrapper(");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t\t");
_builder.append("std::shared_ptr> wrappedListener");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append(") :");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t");
_builder.append("SubscriptionCallback(");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t\t\t");
_builder.append("std::shared_ptr>(new ");
String _joynrName_13 = this._joynrCppGeneratorExtensions.joynrName(broadcast);
String _firstUpper_13 = StringExtensions.toFirstUpper(_joynrName_13);
_builder.append(_firstUpper_13, "\t\t\t\t\t\t");
_builder.append("BroadcastSubscriptionListenerWrapper(wrappedListener)))");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t");
_builder.append("{");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("virtual void onSuccess(");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t");
String _substring = outputParametersSignature.substring(1);
_builder.append(_substring, "\t\t\t\t");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t");
_builder.append(") {");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("std::shared_ptr<");
String _joynrName_14 = this._joynrCppGeneratorExtensions.joynrName(broadcast);
String _firstUpper_14 = StringExtensions.toFirstUpper(_joynrName_14);
_builder.append(_firstUpper_14, "\t\t\t");
_builder.append("BroadcastSubscriptionListenerWrapper> wrapper =");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t\t");
_builder.append("std::dynamic_pointer_cast<");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t\t\t\t");
String _joynrName_15 = this._joynrCppGeneratorExtensions.joynrName(broadcast);
String _firstUpper_15 = StringExtensions.toFirstUpper(_joynrName_15);
_builder.append(_firstUpper_15, "\t\t\t\t\t\t\t");
_builder.append("BroadcastSubscriptionListenerWrapper>(listener);");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("wrapper->onReceive(");
Iterable _outputParameters = this._joynrCppGeneratorExtensions.getOutputParameters(broadcast);
String _commaSeperatedUntypedParameterList = this.cppStdTypeUtil.getCommaSeperatedUntypedParameterList(_outputParameters);
_builder.append(_commaSeperatedUntypedParameterList, "\t\t\t");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t");
_builder.append(");");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("virtual void onError()");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("{");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("std::shared_ptr<");
String _joynrName_16 = this._joynrCppGeneratorExtensions.joynrName(broadcast);
String _firstUpper_16 = StringExtensions.toFirstUpper(_joynrName_16);
_builder.append(_firstUpper_16, "\t\t\t");
_builder.append("BroadcastSubscriptionListenerWrapper> wrapper =");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t\t");
_builder.append("std::dynamic_pointer_cast<");
_builder.newLine();
_builder.append("\t");
_builder.append("\t\t\t\t\t\t");
String _joynrName_17 = this._joynrCppGeneratorExtensions.joynrName(broadcast);
String _firstUpper_17 = StringExtensions.toFirstUpper(_joynrName_17);
_builder.append(_firstUpper_17, "\t\t\t\t\t\t\t");
_builder.append("BroadcastSubscriptionListenerWrapper>(listener);");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("\t\t");
_builder.append("wrapper->onError();");
_builder.newLine();
_builder.append("\t");
_builder.append("\t");
_builder.append("}");
_builder.newLine();
_builder.append("\t");
_builder.append("};");
_builder.newLine();
_builder.newLine();
}
}
}
}
_builder.append("};");
_builder.newLine();
_builder.newLine();
String _namespaceEnder = this._joynrCppGeneratorExtensions.getNamespaceEnder(serviceInterface);
_builder.append(_namespaceEnder, "");
_builder.newLineIfNotEmpty();
_builder.append("#endif // ");
_builder.append(headerGuard, "");
_builder.newLineIfNotEmpty();
return _builder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy