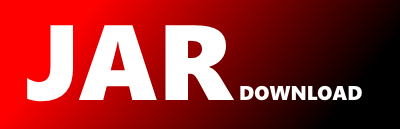
joynr.infrastructure.GlobalDomainAccessControllerProvider Maven / Gradle / Ivy
/*
*
* Copyright (C) 2011 - 2015 BMW Car IT GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// #####################################################
//#######################################################
//### ###
//## WARNING: This file is generated. DO NOT EDIT ##
//## All changes will be lost! ##
//### ###
//#######################################################
// #####################################################
package joynr.infrastructure;
import io.joynr.provider.Promise;
import io.joynr.provider.AbstractDeferred;
import io.joynr.provider.JoynrInterface;
import io.joynr.JoynrVersion;
import joynr.infrastructure.DacTypes.ChangeType;
import joynr.infrastructure.DacTypes.DomainRoleEntry;
import joynr.infrastructure.DacTypes.MasterAccessControlEntry;
import joynr.infrastructure.DacTypes.MasterRegistrationControlEntry;
import joynr.infrastructure.DacTypes.OwnerAccessControlEntry;
import joynr.infrastructure.DacTypes.OwnerRegistrationControlEntry;
import joynr.infrastructure.DacTypes.Role;
import io.joynr.provider.SubscriptionPublisherInjection;
interface GlobalDomainAccessControllerSubscriptionPublisherInjection extends SubscriptionPublisherInjection {}
@JoynrInterface(provides=GlobalDomainAccessControllerProvider.class, name="infrastructure/GlobalDomainAccessController")
@JoynrVersion(major=0, minor=1)
public interface GlobalDomainAccessControllerProvider extends GlobalDomainAccessControllerSubscriptionPublisherInjection {
/**
* getDomainRoles
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getDomainRoles(
String uid
);
/**
* updateDomainRole
* @param updatedEntry the parameter updatedEntry
* @return promise for asynchronous handling
*/
public Promise updateDomainRole(
DomainRoleEntry updatedEntry
);
/**
* removeDomainRole
* @param uid the parameter uid
* @param role the parameter role
* @return promise for asynchronous handling
*/
public Promise removeDomainRole(
String uid,
Role role
);
/**
* getMasterAccessControlEntries
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getMasterAccessControlEntries(
String uid
);
/**
* getEditableMasterAccessControlEntries
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getEditableMasterAccessControlEntries(
String uid
);
/**
* getMasterAccessControlEntries
* @param domain the parameter domain
* @param interfaceName the parameter interfaceName
* @return promise for asynchronous handling
*/
public Promise getMasterAccessControlEntries(
String domain,
String interfaceName
);
/**
* updateMasterAccessControlEntry
* @param updatedMasterAce the parameter updatedMasterAce
* @return promise for asynchronous handling
*/
public Promise updateMasterAccessControlEntry(
MasterAccessControlEntry updatedMasterAce
);
/**
* removeMasterAccessControlEntry
* @param uid the parameter uid
* @param domain the parameter domain
* @param interfaceName the parameter interfaceName
* @param operation the parameter operation
* @return promise for asynchronous handling
*/
public Promise removeMasterAccessControlEntry(
String uid,
String domain,
String interfaceName,
String operation
);
/**
* getMediatorAccessControlEntries
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getMediatorAccessControlEntries(
String uid
);
/**
* getEditableMediatorAccessControlEntries
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getEditableMediatorAccessControlEntries(
String uid
);
/**
* getMediatorAccessControlEntries
* @param domain the parameter domain
* @param interfaceName the parameter interfaceName
* @return promise for asynchronous handling
*/
public Promise getMediatorAccessControlEntries(
String domain,
String interfaceName
);
/**
* updateMediatorAccessControlEntry
* @param updatedMediatorAce the parameter updatedMediatorAce
* @return promise for asynchronous handling
*/
public Promise updateMediatorAccessControlEntry(
MasterAccessControlEntry updatedMediatorAce
);
/**
* removeMediatorAccessControlEntry
* @param uid the parameter uid
* @param domain the parameter domain
* @param interfaceName the parameter interfaceName
* @param operation the parameter operation
* @return promise for asynchronous handling
*/
public Promise removeMediatorAccessControlEntry(
String uid,
String domain,
String interfaceName,
String operation
);
/**
* getOwnerAccessControlEntries
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getOwnerAccessControlEntries(
String uid
);
/**
* getOwnerAccessControlEntries
* @param domain the parameter domain
* @param interfaceName the parameter interfaceName
* @return promise for asynchronous handling
*/
public Promise getOwnerAccessControlEntries(
String domain,
String interfaceName
);
/**
* getEditableOwnerAccessControlEntries
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getEditableOwnerAccessControlEntries(
String uid
);
/**
* updateOwnerAccessControlEntry
* @param updatedOwnerAce the parameter updatedOwnerAce
* @return promise for asynchronous handling
*/
public Promise updateOwnerAccessControlEntry(
OwnerAccessControlEntry updatedOwnerAce
);
/**
* removeOwnerAccessControlEntry
* @param uid the parameter uid
* @param domain the parameter domain
* @param interfaceName the parameter interfaceName
* @param operation the parameter operation
* @return promise for asynchronous handling
*/
public Promise removeOwnerAccessControlEntry(
String uid,
String domain,
String interfaceName,
String operation
);
/**
* getMasterRegistrationControlEntries
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getMasterRegistrationControlEntries(
String uid
);
/**
* getEditableMasterRegistrationControlEntries
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getEditableMasterRegistrationControlEntries(
String uid
);
/**
* updateMasterRegistrationControlEntry
* @param updatedMasterRce the parameter updatedMasterRce
* @return promise for asynchronous handling
*/
public Promise updateMasterRegistrationControlEntry(
MasterRegistrationControlEntry updatedMasterRce
);
/**
* removeMasterRegistrationControlEntry
* @param uid the parameter uid
* @param domain the parameter domain
* @param interfaceName the parameter interfaceName
* @return promise for asynchronous handling
*/
public Promise removeMasterRegistrationControlEntry(
String uid,
String domain,
String interfaceName
);
/**
* getMediatorRegistrationControlEntries
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getMediatorRegistrationControlEntries(
String uid
);
/**
* getEditableMediatorRegistrationControlEntries
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getEditableMediatorRegistrationControlEntries(
String uid
);
/**
* updateMediatorRegistrationControlEntry
* @param updatedMediatorRce the parameter updatedMediatorRce
* @return promise for asynchronous handling
*/
public Promise updateMediatorRegistrationControlEntry(
MasterRegistrationControlEntry updatedMediatorRce
);
/**
* removeMediatorRegistrationControlEntry
* @param uid the parameter uid
* @param domain the parameter domain
* @param interfaceName the parameter interfaceName
* @return promise for asynchronous handling
*/
public Promise removeMediatorRegistrationControlEntry(
String uid,
String domain,
String interfaceName
);
/**
* getOwnerRegistrationControlEntries
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getOwnerRegistrationControlEntries(
String uid
);
/**
* getEditableOwnerRegistrationControlEntries
* @param uid the parameter uid
* @return promise for asynchronous handling
*/
public Promise getEditableOwnerRegistrationControlEntries(
String uid
);
/**
* updateOwnerRegistrationControlEntry
* @param updatedOwnerRce the parameter updatedOwnerRce
* @return promise for asynchronous handling
*/
public Promise updateOwnerRegistrationControlEntry(
OwnerRegistrationControlEntry updatedOwnerRce
);
/**
* removeOwnerRegistrationControlEntry
* @param uid the parameter uid
* @param domain the parameter domain
* @param interfaceName the parameter interfaceName
* @return promise for asynchronous handling
*/
public Promise removeOwnerRegistrationControlEntry(
String uid,
String domain,
String interfaceName
);
public class GetDomainRolesDeferred extends AbstractDeferred {
public synchronized boolean resolve(DomainRoleEntry[] domainRoleEntries) {
return super.resolve((Object)domainRoleEntries);
}
}
public class UpdateDomainRoleDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class RemoveDomainRoleDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class GetMasterAccessControlEntries1Deferred extends AbstractDeferred {
public synchronized boolean resolve(MasterAccessControlEntry[] masterAces) {
return super.resolve((Object)masterAces);
}
}
public class GetEditableMasterAccessControlEntriesDeferred extends AbstractDeferred {
public synchronized boolean resolve(MasterAccessControlEntry[] masterAces) {
return super.resolve((Object)masterAces);
}
}
public class UpdateMasterAccessControlEntryDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class RemoveMasterAccessControlEntryDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class GetMediatorAccessControlEntries1Deferred extends AbstractDeferred {
public synchronized boolean resolve(MasterAccessControlEntry[] mediatorAces) {
return super.resolve((Object)mediatorAces);
}
}
public class GetEditableMediatorAccessControlEntriesDeferred extends AbstractDeferred {
public synchronized boolean resolve(MasterAccessControlEntry[] mediatorAces) {
return super.resolve((Object)mediatorAces);
}
}
public class UpdateMediatorAccessControlEntryDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class RemoveMediatorAccessControlEntryDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class GetOwnerAccessControlEntries1Deferred extends AbstractDeferred {
public synchronized boolean resolve(OwnerAccessControlEntry[] ownerAces) {
return super.resolve((Object)ownerAces);
}
}
public class GetEditableOwnerAccessControlEntriesDeferred extends AbstractDeferred {
public synchronized boolean resolve(OwnerAccessControlEntry[] ownerAces) {
return super.resolve((Object)ownerAces);
}
}
public class UpdateOwnerAccessControlEntryDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class RemoveOwnerAccessControlEntryDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class GetMasterRegistrationControlEntriesDeferred extends AbstractDeferred {
public synchronized boolean resolve(MasterRegistrationControlEntry[] masterRces) {
return super.resolve((Object)masterRces);
}
}
public class GetEditableMasterRegistrationControlEntriesDeferred extends AbstractDeferred {
public synchronized boolean resolve(MasterRegistrationControlEntry[] masterRces) {
return super.resolve((Object)masterRces);
}
}
public class UpdateMasterRegistrationControlEntryDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class RemoveMasterRegistrationControlEntryDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class GetMediatorRegistrationControlEntriesDeferred extends AbstractDeferred {
public synchronized boolean resolve(MasterRegistrationControlEntry[] mediatorRces) {
return super.resolve((Object)mediatorRces);
}
}
public class GetEditableMediatorRegistrationControlEntriesDeferred extends AbstractDeferred {
public synchronized boolean resolve(MasterRegistrationControlEntry[] mediatorRces) {
return super.resolve((Object)mediatorRces);
}
}
public class UpdateMediatorRegistrationControlEntryDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class RemoveMediatorRegistrationControlEntryDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class GetOwnerRegistrationControlEntriesDeferred extends AbstractDeferred {
public synchronized boolean resolve(OwnerRegistrationControlEntry[] ownerRces) {
return super.resolve((Object)ownerRces);
}
}
public class GetEditableOwnerRegistrationControlEntriesDeferred extends AbstractDeferred {
public synchronized boolean resolve(OwnerRegistrationControlEntry[] ownerRces) {
return super.resolve((Object)ownerRces);
}
}
public class UpdateOwnerRegistrationControlEntryDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
public class RemoveOwnerRegistrationControlEntryDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean success) {
return super.resolve(success);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy