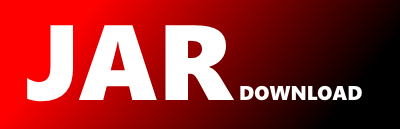
node_modules.smrf-native.src.util.h Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libjoynr-js Show documentation
Show all versions of libjoynr-js Show documentation
JOYnr JavaScript libjoynr-js
/*
* #%L
* %%
* Copyright (C) 2017 BMW Car IT GmbH
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
#ifndef UTIL_H
#define UTIL_H
#include
#include
#include
#include "ExternalString.h"
namespace util
{
void noOpFreeCallback(char* data, void* hint)
{
std::ignore = data;
std::ignore = hint;
}
char* castToCharPointer(const smrf::Byte* buffer)
{
return const_cast(reinterpret_cast(buffer));
}
decltype(auto) bufferWitNoOpFreeCallback(const smrf::ByteArrayView& view)
{
return Nan::NewBuffer(castToCharPointer(view.data()), view.size(), noOpFreeCallback, nullptr).ToLocalChecked();
}
template
static void bufferFreeCallback(char* data, void* hint)
{
std::ignore = data;
delete reinterpret_cast(hint);
}
auto bufferToByteArrayView(v8::Local buffer)
{
if (!node::Buffer::HasInstance(buffer)) {
throw std::invalid_argument("'buffer' must be a buffer");
}
const smrf::Byte* bufferPointer = reinterpret_cast(node::Buffer::Data(buffer));
const std::size_t bufferSize = node::Buffer::Length(buffer);
return smrf::ByteArrayView(bufferPointer, bufferSize);
}
auto bufferToByteVector(v8::Local buffer)
{
smrf::ByteArrayView view = bufferToByteArrayView(buffer->ToObject());
return smrf::ByteVector(view.data(), view.data() + view.size());
}
template
auto string(const char (&str)[N])
{
return Nan::New(&str[0], N - 1).ToLocalChecked();
}
auto viewToBuffer(const smrf::ByteArrayView& view)
{
return Nan::CopyBuffer(reinterpret_cast(view.data()), view.size()).ToLocalChecked();
}
auto viewToString(const smrf::ByteArrayView& view)
{
return Nan::New(reinterpret_cast(view.data()), view.size()).ToLocalChecked();
}
auto viewToExternalString(const smrf::ByteArrayView& view)
{
return Nan::New(new ExternalString(reinterpret_cast(view.data()), view.size())).ToLocalChecked();
}
auto byteVectorToBuffer(smrf::ByteVector&& vec)
{
// store vec in a heap allocated vector
// node buffer wraps around that vector's data
// this vector will be deleted when the node Buffer goes out of scope
auto* byteVector = new smrf::ByteVector(std::move(vec));
return Nan::NewBuffer(
castToCharPointer(byteVector->data()), byteVector->size(), bufferFreeCallback, byteVector)
.ToLocalChecked();
}
} // namespace util
#endif // UTIL_H
© 2015 - 2025 Weber Informatics LLC | Privacy Policy