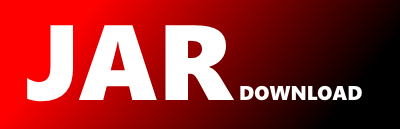
joynr.interlanguagetest.TestInterfaceProvider Maven / Gradle / Ivy
/*
*
* Copyright (C) 2011 - 2018 BMW Car IT GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// #####################################################
//#######################################################
//### ###
//## WARNING: This file is generated. DO NOT EDIT ##
//## All changes will be lost! ##
//### ###
//#######################################################
// #####################################################
package joynr.interlanguagetest;
import io.joynr.provider.Promise;
import io.joynr.provider.Deferred;
import io.joynr.provider.AbstractDeferred;
import io.joynr.provider.DeferredVoid;
import joynr.exceptions.ApplicationException;
import io.joynr.provider.JoynrInterface;
import io.joynr.JoynrVersion;
import joynr.interlanguagetest.Enumeration;
import joynr.interlanguagetest.namedTypeCollection1.StructWithStringArray;
import joynr.interlanguagetest.namedTypeCollection2.BaseStruct;
import joynr.interlanguagetest.namedTypeCollection2.BaseStructWithoutElements;
import joynr.interlanguagetest.namedTypeCollection2.ExtendedBaseStruct;
import joynr.interlanguagetest.namedTypeCollection2.ExtendedEnumerationWithPartlyDefinedValues;
import joynr.interlanguagetest.namedTypeCollection2.ExtendedExtendedBaseStruct;
import joynr.interlanguagetest.namedTypeCollection2.ExtendedExtendedEnumeration;
import joynr.interlanguagetest.namedTypeCollection2.ExtendedInterfaceEnumerationInTypeCollection;
import joynr.interlanguagetest.namedTypeCollection2.ExtendedStructOfPrimitives;
import joynr.interlanguagetest.namedTypeCollection2.ExtendedTypeCollectionEnumerationInTypeCollection;
import joynr.interlanguagetest.namedTypeCollection2.MapStringString;
import io.joynr.provider.SubscriptionPublisherInjection;
interface TestInterfaceSubscriptionPublisherInjection extends SubscriptionPublisherInjection {}
@JoynrInterface(provides = TestInterface.class, provider = TestInterfaceProvider.class, name = "interlanguagetest/TestInterface")
@JoynrVersion(major = 0, minor = 1)
public interface TestInterfaceProvider extends TestInterfaceSubscriptionPublisherInjection {
Promise> getAttributeUInt8();
Promise setAttributeUInt8(Byte attributeUInt8);
Promise> getAttributeDouble();
Promise setAttributeDouble(Double attributeDouble);
Promise> getAttributeBooleanReadonly();
Promise> getAttributeStringNoSubscriptions();
Promise setAttributeStringNoSubscriptions(String attributeStringNoSubscriptions);
Promise> getAttributeInt8readonlyNoSubscriptions();
Promise> getAttributeArrayOfStringImplicit();
Promise setAttributeArrayOfStringImplicit(String[] attributeArrayOfStringImplicit);
Promise> getAttributeEnumeration();
Promise setAttributeEnumeration(Enumeration attributeEnumeration);
Promise> getAttributeExtendedEnumerationReadonly();
Promise> getAttributeBaseStruct();
Promise setAttributeBaseStruct(BaseStruct attributeBaseStruct);
Promise> getAttributeExtendedExtendedBaseStruct();
Promise setAttributeExtendedExtendedBaseStruct(ExtendedExtendedBaseStruct attributeExtendedExtendedBaseStruct);
Promise> getAttributeMapStringString();
Promise setAttributeMapStringString(MapStringString attributeMapStringString);
Promise> getAttributeWithExceptionFromGetter();
Promise> getAttributeWithExceptionFromSetter();
Promise setAttributeWithExceptionFromSetter(Boolean attributeWithExceptionFromSetter);
Promise> getAttributeFireAndForget();
Promise setAttributeFireAndForget(Integer attributeFireAndForget);
/**
* methodWithoutParameters
* @return promise for asynchronous handling
*/
public Promise methodWithoutParameters(
);
/**
* methodWithoutInputParameter
* @return promise for asynchronous handling
*/
public Promise methodWithoutInputParameter(
);
/**
* methodWithoutOutputParameter
* @param booleanArg the parameter booleanArg
* @return promise for asynchronous handling
*/
public Promise methodWithoutOutputParameter(
Boolean booleanArg
);
/**
* methodWithSinglePrimitiveParameters
* @param uInt16Arg the parameter uInt16Arg
* @return promise for asynchronous handling
*/
public Promise methodWithSinglePrimitiveParameters(
Short uInt16Arg
);
/**
* methodWithMultiplePrimitiveParameters
* @param int32Arg the parameter int32Arg
* @param floatArg the parameter floatArg
* @param booleanArg the parameter booleanArg
* @return promise for asynchronous handling
*/
public Promise methodWithMultiplePrimitiveParameters(
Integer int32Arg,
Float floatArg,
Boolean booleanArg
);
/**
* methodWithSingleArrayParameters
* @param doubleArrayArg the parameter doubleArrayArg
* @return promise for asynchronous handling
*/
public Promise methodWithSingleArrayParameters(
Double[] doubleArrayArg
);
/**
* methodWithMultipleArrayParameters
* @param stringArrayArg the parameter stringArrayArg
* @param int8ArrayArg the parameter int8ArrayArg
* @param enumArrayArg the parameter enumArrayArg
* @param structWithStringArrayArrayArg the parameter structWithStringArrayArrayArg
* @return promise for asynchronous handling
*/
public Promise methodWithMultipleArrayParameters(
String[] stringArrayArg,
Byte[] int8ArrayArg,
ExtendedInterfaceEnumerationInTypeCollection[] enumArrayArg,
StructWithStringArray[] structWithStringArrayArrayArg
);
/**
* methodWithSingleEnumParameters
* @param enumerationArg the parameter enumerationArg
* @return promise for asynchronous handling
*/
public Promise methodWithSingleEnumParameters(
ExtendedEnumerationWithPartlyDefinedValues enumerationArg
);
/**
* methodWithMultipleEnumParameters
* @param enumerationArg the parameter enumerationArg
* @param extendedEnumerationArg the parameter extendedEnumerationArg
* @return promise for asynchronous handling
*/
public Promise methodWithMultipleEnumParameters(
Enumeration enumerationArg,
ExtendedTypeCollectionEnumerationInTypeCollection extendedEnumerationArg
);
/**
* methodWithSingleMapParameters
* @param mapArg the parameter mapArg
* @return promise for asynchronous handling
*/
public Promise methodWithSingleMapParameters(
MapStringString mapArg
);
/**
* methodWithSingleStructParameters
* @param extendedBaseStructArg the parameter extendedBaseStructArg
* @return promise for asynchronous handling
*/
public Promise methodWithSingleStructParameters(
ExtendedBaseStruct extendedBaseStructArg
);
/**
* methodWithMultipleStructParameters
* @param extendedStructOfPrimitivesArg the parameter extendedStructOfPrimitivesArg
* @param baseStructArg the parameter baseStructArg
* @return promise for asynchronous handling
*/
public Promise methodWithMultipleStructParameters(
ExtendedStructOfPrimitives extendedStructOfPrimitivesArg,
BaseStruct baseStructArg
);
/**
* methodWithStringsAndSpecifiedStringOutLength
* @param stringArg the parameter stringArg
* @param int32StringLengthArg the parameter int32StringLengthArg
* @return promise for asynchronous handling
*/
public Promise methodWithStringsAndSpecifiedStringOutLength(
String stringArg,
Integer int32StringLengthArg
);
/**
* methodFireAndForgetWithoutParameter
* @return promise for asynchronous handling
*/
public void methodFireAndForgetWithoutParameter(
);
/**
* methodFireAndForgetWithInputParameter
* @param int32Arg the parameter int32Arg
* @return promise for asynchronous handling
*/
public void methodFireAndForgetWithInputParameter(
Integer int32Arg
);
/**
* overloadedMethod
* @return promise for asynchronous handling
*/
public Promise overloadedMethod(
);
/**
* overloadedMethod
* @param booleanArg the parameter booleanArg
* @return promise for asynchronous handling
*/
public Promise overloadedMethod(
Boolean booleanArg
);
/**
* overloadedMethod
* @param enumArrayArg the parameter enumArrayArg
* @param int64Arg the parameter int64Arg
* @param baseStructArg the parameter baseStructArg
* @param booleanArg the parameter booleanArg
* @return promise for asynchronous handling
*/
public Promise overloadedMethod(
ExtendedExtendedEnumeration[] enumArrayArg,
Long int64Arg,
BaseStruct baseStructArg,
Boolean booleanArg
);
/**
* overloadedMethodWithSelector
* @return promise for asynchronous handling
*/
public Promise overloadedMethodWithSelector(
);
/**
* overloadedMethodWithSelector
* @param booleanArg the parameter booleanArg
* @return promise for asynchronous handling
*/
public Promise overloadedMethodWithSelector(
Boolean booleanArg
);
/**
* overloadedMethodWithSelector
* @param enumArrayArg the parameter enumArrayArg
* @param int64Arg the parameter int64Arg
* @param baseStructArg the parameter baseStructArg
* @param booleanArg the parameter booleanArg
* @return promise for asynchronous handling
*/
public Promise overloadedMethodWithSelector(
ExtendedExtendedEnumeration[] enumArrayArg,
Long int64Arg,
BaseStruct baseStructArg,
Boolean booleanArg
);
/**
* methodWithoutErrorEnum
* @param wantedExceptionArg the parameter wantedExceptionArg
* @return promise for asynchronous handling
*/
public Promise methodWithoutErrorEnum(
String wantedExceptionArg
);
/**
* methodWithAnonymousErrorEnum
* @param wantedExceptionArg the parameter wantedExceptionArg
* @return promise for asynchronous handling
*/
public Promise methodWithAnonymousErrorEnum(
String wantedExceptionArg
);
/**
* methodWithExistingErrorEnum
* @param wantedExceptionArg the parameter wantedExceptionArg
* @return promise for asynchronous handling
*/
public Promise methodWithExistingErrorEnum(
String wantedExceptionArg
);
/**
* methodWithExtendedErrorEnum
* @param wantedExceptionArg the parameter wantedExceptionArg
* @return promise for asynchronous handling
*/
public Promise methodWithExtendedErrorEnum(
String wantedExceptionArg
);
/**
* methodToFireBroadcastWithSinglePrimitiveParameter
* @return promise for asynchronous handling
*/
public Promise methodToFireBroadcastWithSinglePrimitiveParameter(
);
/**
* methodToFireBroadcastWithMultiplePrimitiveParameters
* @return promise for asynchronous handling
*/
public Promise methodToFireBroadcastWithMultiplePrimitiveParameters(
);
/**
* methodToFireBroadcastWithSingleArrayParameter
* @return promise for asynchronous handling
*/
public Promise methodToFireBroadcastWithSingleArrayParameter(
);
/**
* methodToFireBroadcastWithMultipleArrayParameters
* @return promise for asynchronous handling
*/
public Promise methodToFireBroadcastWithMultipleArrayParameters(
);
/**
* methodToFireBroadcastWithSingleEnumerationParameter
* @return promise for asynchronous handling
*/
public Promise methodToFireBroadcastWithSingleEnumerationParameter(
);
/**
* methodToFireBroadcastWithMultipleEnumerationParameters
* @return promise for asynchronous handling
*/
public Promise methodToFireBroadcastWithMultipleEnumerationParameters(
);
/**
* methodToFireBroadcastWithSingleStructParameter
* @return promise for asynchronous handling
*/
public Promise methodToFireBroadcastWithSingleStructParameter(
);
/**
* methodToFireBroadcastWithMultipleStructParameters
* @return promise for asynchronous handling
*/
public Promise methodToFireBroadcastWithMultipleStructParameters(
);
/**
* methodToFireBroadcastWithFiltering
* @param stringArg the parameter stringArg
* @return promise for asynchronous handling
*/
public Promise methodToFireBroadcastWithFiltering(
String stringArg
);
public class MethodWithoutInputParameterDeferred extends AbstractDeferred {
public synchronized boolean resolve(Boolean booleanOut) {
return super.resolve(booleanOut);
}
}
public class MethodWithSinglePrimitiveParametersDeferred extends AbstractDeferred {
public synchronized boolean resolve(String stringOut) {
return super.resolve(stringOut);
}
}
public class MethodWithMultiplePrimitiveParametersDeferred extends AbstractDeferred {
public synchronized boolean resolve(Double doubleOut, String stringOut) {
return super.resolve(doubleOut, stringOut);
}
}
public class MethodWithSingleArrayParametersDeferred extends AbstractDeferred {
public synchronized boolean resolve(String[] stringArrayOut) {
return super.resolve((Object)stringArrayOut);
}
}
public class MethodWithMultipleArrayParametersDeferred extends AbstractDeferred {
public synchronized boolean resolve(Long[] uInt64ArrayOut, StructWithStringArray[] structWithStringArrayArrayOut) {
return super.resolve(uInt64ArrayOut, structWithStringArrayArrayOut);
}
}
public class MethodWithSingleEnumParametersDeferred extends AbstractDeferred {
public synchronized boolean resolve(ExtendedTypeCollectionEnumerationInTypeCollection enumerationOut) {
return super.resolve(enumerationOut);
}
}
public class MethodWithMultipleEnumParametersDeferred extends AbstractDeferred {
public synchronized boolean resolve(ExtendedEnumerationWithPartlyDefinedValues extendedEnumerationOut, Enumeration enumerationOut) {
return super.resolve(extendedEnumerationOut, enumerationOut);
}
}
public class MethodWithSingleMapParametersDeferred extends AbstractDeferred {
public synchronized boolean resolve(MapStringString mapOut) {
return super.resolve(mapOut);
}
}
public class MethodWithSingleStructParametersDeferred extends AbstractDeferred {
public synchronized boolean resolve(ExtendedStructOfPrimitives extendedStructOfPrimitivesOut) {
return super.resolve(extendedStructOfPrimitivesOut);
}
}
public class MethodWithMultipleStructParametersDeferred extends AbstractDeferred {
public synchronized boolean resolve(BaseStructWithoutElements baseStructWithoutElementsOut, ExtendedExtendedBaseStruct extendedExtendedBaseStructOut) {
return super.resolve(baseStructWithoutElementsOut, extendedExtendedBaseStructOut);
}
}
public class MethodWithStringsAndSpecifiedStringOutLengthDeferred extends AbstractDeferred {
public synchronized boolean resolve(String stringOut) {
return super.resolve(stringOut);
}
}
public class OverloadedMethod1Deferred extends AbstractDeferred {
public synchronized boolean resolve(String stringOut) {
return super.resolve(stringOut);
}
}
public class OverloadedMethod2Deferred extends AbstractDeferred {
public synchronized boolean resolve(Double doubleOut, String[] stringArrayOut, ExtendedBaseStruct extendedBaseStructOut) {
return super.resolve(doubleOut, stringArrayOut, extendedBaseStructOut);
}
}
public class OverloadedMethodWithSelector1Deferred extends AbstractDeferred {
public synchronized boolean resolve(String stringOut) {
return super.resolve(stringOut);
}
}
public class OverloadedMethodWithSelector2Deferred extends AbstractDeferred {
public synchronized boolean resolve(Double doubleOut, String[] stringArrayOut, ExtendedBaseStruct extendedBaseStructOut) {
return super.resolve(doubleOut, stringArrayOut, extendedBaseStructOut);
}
}
public class MethodWithAnonymousErrorEnumDeferred extends AbstractDeferred {
public synchronized boolean resolve() {
Object[] values = new Object[] {};
return super.resolve(values);
}
public synchronized boolean reject(joynr.interlanguagetest.TestInterface.MethodWithAnonymousErrorEnumErrorEnum error) {
return super.reject(new ApplicationException(error));
}
}
public class MethodWithExistingErrorEnumDeferred extends AbstractDeferred {
public synchronized boolean resolve() {
Object[] values = new Object[] {};
return super.resolve(values);
}
public synchronized boolean reject(joynr.interlanguagetest.namedTypeCollection2.ExtendedErrorEnumTc error) {
return super.reject(new ApplicationException(error));
}
}
public class MethodWithExtendedErrorEnumDeferred extends AbstractDeferred {
public synchronized boolean resolve() {
Object[] values = new Object[] {};
return super.resolve(values);
}
public synchronized boolean reject(joynr.interlanguagetest.TestInterface.MethodWithExtendedErrorEnumErrorEnum error) {
return super.reject(new ApplicationException(error));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy