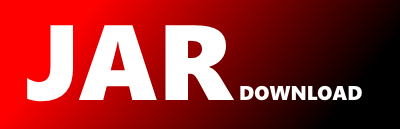
org.hyperic.sigar.DirUsage Maven / Gradle / Ivy
/*****************************************************
* WARNING: this file was generated by -e
* on Mon May 24 16:26:06 2010.
* Any changes made here will be LOST.
*****************************************************/
package org.hyperic.sigar;
import java.util.HashMap;
import java.util.Map;
/**
* DirUsage sigar class.
*/
public class DirUsage implements java.io.Serializable {
private static final long serialVersionUID = 9250L;
public DirUsage() { }
public native void gather(Sigar sigar, String name) throws SigarException;
/**
* This method is not intended to be called directly.
* use Sigar.getDirUsage() instead.
* @exception SigarException on failure.
* @see org.hyperic.sigar.Sigar#getDirUsage
*/
static DirUsage fetch(Sigar sigar, String name) throws SigarException {
DirUsage dirUsage = new DirUsage();
dirUsage.gather(sigar, name);
return dirUsage;
}
long total = 0;
/**
* Get the total.
* Supported Platforms: Undocumented.
*
* @return total
*/
public long getTotal() { return total; }
long files = 0;
/**
* Get the files.
* Supported Platforms: Undocumented.
*
* @return files
*/
public long getFiles() { return files; }
long subdirs = 0;
/**
* Get the subdirs.
* Supported Platforms: Undocumented.
*
* @return subdirs
*/
public long getSubdirs() { return subdirs; }
long symlinks = 0;
/**
* Get the symlinks.
* Supported Platforms: Undocumented.
*
* @return symlinks
*/
public long getSymlinks() { return symlinks; }
long chrdevs = 0;
/**
* Get the chrdevs.
* Supported Platforms: Undocumented.
*
* @return chrdevs
*/
public long getChrdevs() { return chrdevs; }
long blkdevs = 0;
/**
* Get the blkdevs.
* Supported Platforms: Undocumented.
*
* @return blkdevs
*/
public long getBlkdevs() { return blkdevs; }
long sockets = 0;
/**
* Get the sockets.
* Supported Platforms: Undocumented.
*
* @return sockets
*/
public long getSockets() { return sockets; }
long diskUsage = 0;
/**
* Get the disk_usage.
* Supported Platforms: Undocumented.
*
* @return disk_usage
*/
public long getDiskUsage() { return diskUsage; }
void copyTo(DirUsage copy) {
copy.total = this.total;
copy.files = this.files;
copy.subdirs = this.subdirs;
copy.symlinks = this.symlinks;
copy.chrdevs = this.chrdevs;
copy.blkdevs = this.blkdevs;
copy.sockets = this.sockets;
copy.diskUsage = this.diskUsage;
}
public Map toMap() {
Map map = new HashMap();
String strtotal =
String.valueOf(this.total);
if (!"-1".equals(strtotal))
map.put("Total", strtotal);
String strfiles =
String.valueOf(this.files);
if (!"-1".equals(strfiles))
map.put("Files", strfiles);
String strsubdirs =
String.valueOf(this.subdirs);
if (!"-1".equals(strsubdirs))
map.put("Subdirs", strsubdirs);
String strsymlinks =
String.valueOf(this.symlinks);
if (!"-1".equals(strsymlinks))
map.put("Symlinks", strsymlinks);
String strchrdevs =
String.valueOf(this.chrdevs);
if (!"-1".equals(strchrdevs))
map.put("Chrdevs", strchrdevs);
String strblkdevs =
String.valueOf(this.blkdevs);
if (!"-1".equals(strblkdevs))
map.put("Blkdevs", strblkdevs);
String strsockets =
String.valueOf(this.sockets);
if (!"-1".equals(strsockets))
map.put("Sockets", strsockets);
String strdiskUsage =
String.valueOf(this.diskUsage);
if (!"-1".equals(strdiskUsage))
map.put("DiskUsage", strdiskUsage);
return map;
}
public String toString() {
return toMap().toString();
}
}