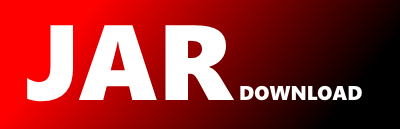
org.hyperic.sigar.DiskUsage Maven / Gradle / Ivy
/*****************************************************
* WARNING: this file was generated by -e
* on Mon May 24 16:26:06 2010.
* Any changes made here will be LOST.
*****************************************************/
package org.hyperic.sigar;
import java.util.HashMap;
import java.util.Map;
/**
* DiskUsage sigar class.
*/
public class DiskUsage implements java.io.Serializable {
private static final long serialVersionUID = 8090L;
public DiskUsage() { }
public native void gather(Sigar sigar, String name) throws SigarException;
/**
* This method is not intended to be called directly.
* use Sigar.getDiskUsage() instead.
* @exception SigarException on failure.
* @see org.hyperic.sigar.Sigar#getDiskUsage
*/
static DiskUsage fetch(Sigar sigar, String name) throws SigarException {
DiskUsage diskUsage = new DiskUsage();
diskUsage.gather(sigar, name);
return diskUsage;
}
long reads = 0;
/**
* Get the Number of physical disk reads.
* Supported Platforms: AIX, FreeBSD, HPUX, Linux, Solaris, Win32.
*
* @return Number of physical disk reads
*/
public long getReads() { return reads; }
long writes = 0;
/**
* Get the Number of physical disk writes.
* Supported Platforms: AIX, FreeBSD, HPUX, Linux, Solaris, Win32.
*
* @return Number of physical disk writes
*/
public long getWrites() { return writes; }
long readBytes = 0;
/**
* Get the Number of physical disk bytes read.
* Supported Platforms: Undocumented.
*
* @return Number of physical disk bytes read
*/
public long getReadBytes() { return readBytes; }
long writeBytes = 0;
/**
* Get the Number of physical disk bytes written.
* Supported Platforms: Undocumented.
*
* @return Number of physical disk bytes written
*/
public long getWriteBytes() { return writeBytes; }
double queue = 0;
/**
* Get the queue.
* Supported Platforms: Undocumented.
*
* @return queue
*/
public double getQueue() { return queue; }
double serviceTime = 0;
/**
* Get the service_time.
* Supported Platforms: Undocumented.
*
* @return service_time
*/
public double getServiceTime() { return serviceTime; }
void copyTo(DiskUsage copy) {
copy.reads = this.reads;
copy.writes = this.writes;
copy.readBytes = this.readBytes;
copy.writeBytes = this.writeBytes;
copy.queue = this.queue;
copy.serviceTime = this.serviceTime;
}
public Map toMap() {
Map map = new HashMap();
String strreads =
String.valueOf(this.reads);
if (!"-1".equals(strreads))
map.put("Reads", strreads);
String strwrites =
String.valueOf(this.writes);
if (!"-1".equals(strwrites))
map.put("Writes", strwrites);
String strreadBytes =
String.valueOf(this.readBytes);
if (!"-1".equals(strreadBytes))
map.put("ReadBytes", strreadBytes);
String strwriteBytes =
String.valueOf(this.writeBytes);
if (!"-1".equals(strwriteBytes))
map.put("WriteBytes", strwriteBytes);
String strqueue =
String.valueOf(this.queue);
if (!"-1".equals(strqueue))
map.put("Queue", strqueue);
String strserviceTime =
String.valueOf(this.serviceTime);
if (!"-1".equals(strserviceTime))
map.put("ServiceTime", strserviceTime);
return map;
}
public String toString() {
return toMap().toString();
}
}