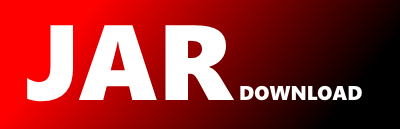
org.hyperic.sigar.FileInfo Maven / Gradle / Ivy
/*
* Copyright (c) 2006-2007 Hyperic, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.hyperic.sigar;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
public class FileInfo extends FileAttrs implements java.io.Serializable {
private static final long serialVersionUID = 02242007L;
private static final SimpleDateFormat DATE_FORMAT =
new SimpleDateFormat("MMM dd HH:mm");
String name;
private transient Sigar sigar;
private boolean dirStatEnabled = false;
private DirStat stat = null;
private boolean lstat;
private FileInfo oldInfo = null;
/**
* No file type determined.
*/
public static final int TYPE_NOFILE = 0;
/**
* A regular file.
*/
public static final int TYPE_REG = 1;
/**
* A directory.
*/
public static final int TYPE_DIR = 2;
/**
* A character device.
*/
public static final int TYPE_CHR = 3;
/**
* A block device.
*/
public static final int TYPE_BLK = 4;
/**
* A FIFO / pipe.
*/
public static final int TYPE_PIPE = 5;
/**
* A symbolic link.
*/
public static final int TYPE_LNK = 6;
/**
* A [unix domain] socket.
*/
public static final int TYPE_SOCK = 7;
/**
* A file of unknown type.
*/
public static final int TYPE_UNKFILE = 8;
/**
* Readable by user.
*/
public static final int MODE_UREAD = 0x0400;
/**
* Writable by user.
*/
public static final int MODE_UWRITE = 0x0200;
/**
* Executable by user.
*/
public static final int MODE_UEXECUTE = 0x0100;
/**
* Readable by group.
*/
public static final int MODE_GREAD = 0x0040;
/**
* Writable by group.
*/
public static final int MODE_GWRITE = 0x0020;
/**
* Executable by group.
*/
public static final int MODE_GEXECUTE = 0x0010;
/**
* Readable by others.
*/
public static final int MODE_WREAD = 0x0004;
/**
* Writable by others.
*/
public static final int MODE_WWRITE = 0x0002;
/**
* Executable by others.
*/
public static final int MODE_WEXECUTE = 0x0001;
private static native String getTypeString(int type);
native void gatherLink(Sigar sigar, String name)
throws SigarException;
public String getTypeString() {
return FileInfo.getTypeString(this.type);
}
public char getTypeChar() {
switch (this.type) {
case TYPE_DIR:
return 'd';
case TYPE_CHR:
return 'c';
case TYPE_BLK:
return 'b';
case TYPE_PIPE:
return 'p';
case TYPE_LNK:
return 'l';
case TYPE_SOCK:
return 's';
default:
return '-';
}
}
public String getName() {
return this.name;
}
public int hashCode() {
return this.name.hashCode();
}
public boolean equals(Object o) {
return o.equals(this.name);
}
private static native String getPermissionsString(long type);
public String getPermissionsString() {
return FileInfo.getPermissionsString(this.permissions);
}
private static native int getMode(long permissions);
/**
* Convert permissions bit mask to human readable number.
* Example:
* MODE_UREAD|MODE_UWRITE|MODE_GREAD|MODE_WREAD
* converts to 644
.
* @return The file permissions mode.
*/
public int getMode() {
return FileInfo.getMode(this.permissions);
}
public void enableDirStat(boolean value) {
this.dirStatEnabled = value;
if (value) {
if (this.type != TYPE_DIR) {
throw new IllegalArgumentException(this.name + " is not a directory");
}
try {
if (this.stat == null) {
this.stat = this.sigar.getDirStat(this.name);
}
else {
this.stat.gather(this.sigar, this.name);
}
} catch (SigarException e) {
//ok for now
}
}
}
private class Diff {
private String attr, old, cur;
Diff(String attr, String old, String cur) {
this.attr = attr;
this.old = old;
this.cur = cur;
}
Diff(String attr, int old, int cur) {
this(attr,
String.valueOf(old),
String.valueOf(cur));
}
Diff(String attr, long old, long cur) {
this(attr,
String.valueOf(old),
String.valueOf(cur));
}
public String toString() {
return this.attr + ": " +
this.old + "|" + this.cur;
}
}
private StringBuffer format(ArrayList changes) {
StringBuffer sb = new StringBuffer();
if (changes.size() == 0) {
return sb;
}
int size = changes.size();
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy