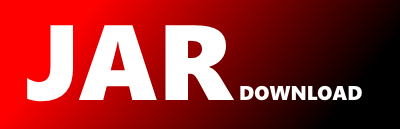
org.hyperic.sigar.FileSystemUsage Maven / Gradle / Ivy
/*****************************************************
* WARNING: this file was generated by -e
* on Mon May 24 16:26:06 2010.
* Any changes made here will be LOST.
*****************************************************/
package org.hyperic.sigar;
import java.util.HashMap;
import java.util.Map;
/**
* FileSystemUsage sigar class.
*/
public class FileSystemUsage implements java.io.Serializable {
private static final long serialVersionUID = 18905L;
public FileSystemUsage() { }
public native void gather(Sigar sigar, String name) throws SigarException;
/**
* This method is not intended to be called directly.
* use Sigar.getFileSystemUsage() instead.
* @exception SigarException on failure.
* @see org.hyperic.sigar.Sigar#getFileSystemUsage
*/
static FileSystemUsage fetch(Sigar sigar, String name) throws SigarException {
FileSystemUsage fileSystemUsage = new FileSystemUsage();
fileSystemUsage.gather(sigar, name);
return fileSystemUsage;
}
long total = 0;
/**
* Get the Total Kbytes of filesystem.
* Supported Platforms: All.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return Total Kbytes of filesystem
*/
public long getTotal() { return total; }
long free = 0;
/**
* Get the Total free Kbytes on filesystem.
* Supported Platforms: All.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return Total free Kbytes on filesystem
*/
public long getFree() { return free; }
long used = 0;
/**
* Get the Total used Kbytes on filesystem.
* Supported Platforms: All.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return Total used Kbytes on filesystem
*/
public long getUsed() { return used; }
long avail = 0;
/**
* Get the Total free Kbytes on filesystem available to caller.
* Supported Platforms: All.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return Total free Kbytes on filesystem available to caller
*/
public long getAvail() { return avail; }
long files = 0;
/**
* Get the Total number of file nodes on the filesystem.
* Supported Platforms: AIX, Darwin, FreeBSD, HPUX, Linux, Solaris.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return Total number of file nodes on the filesystem
*/
public long getFiles() { return files; }
long freeFiles = 0;
/**
* Get the Number of free file nodes on the filesystem.
* Supported Platforms: AIX, Darwin, FreeBSD, HPUX, Linux, Solaris.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return Number of free file nodes on the filesystem
*/
public long getFreeFiles() { return freeFiles; }
long diskReads = 0;
/**
* Get the Number of physical disk reads.
* Supported Platforms: AIX, FreeBSD, HPUX, Linux, Solaris, Win32.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return Number of physical disk reads
*/
public long getDiskReads() { return diskReads; }
long diskWrites = 0;
/**
* Get the Number of physical disk writes.
* Supported Platforms: AIX, FreeBSD, HPUX, Linux, Solaris, Win32.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return Number of physical disk writes
*/
public long getDiskWrites() { return diskWrites; }
long diskReadBytes = 0;
/**
* Get the Number of physical disk bytes read.
* Supported Platforms: Undocumented.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return Number of physical disk bytes read
*/
public long getDiskReadBytes() { return diskReadBytes; }
long diskWriteBytes = 0;
/**
* Get the Number of physical disk bytes written.
* Supported Platforms: Undocumented.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return Number of physical disk bytes written
*/
public long getDiskWriteBytes() { return diskWriteBytes; }
double diskQueue = 0;
/**
* Get the disk_queue.
* Supported Platforms: Undocumented.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return disk_queue
*/
public double getDiskQueue() { return diskQueue; }
double diskServiceTime = 0;
/**
* Get the disk_service_time.
* Supported Platforms: Undocumented.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return disk_service_time
*/
public double getDiskServiceTime() { return diskServiceTime; }
double usePercent = 0;
/**
* Get the Percent of disk used.
* Supported Platforms: All.
*
* System equivalent commands:
* - AIX:
df
* - Darwin:
df
* - FreeBSD:
df
* - HPUX:
df
* - Linux:
df
* - Solaris:
df
* - Win32:
*
* @return Percent of disk used
*/
public double getUsePercent() { return usePercent; }
void copyTo(FileSystemUsage copy) {
copy.total = this.total;
copy.free = this.free;
copy.used = this.used;
copy.avail = this.avail;
copy.files = this.files;
copy.freeFiles = this.freeFiles;
copy.diskReads = this.diskReads;
copy.diskWrites = this.diskWrites;
copy.diskReadBytes = this.diskReadBytes;
copy.diskWriteBytes = this.diskWriteBytes;
copy.diskQueue = this.diskQueue;
copy.diskServiceTime = this.diskServiceTime;
copy.usePercent = this.usePercent;
}
public Map toMap() {
Map map = new HashMap();
String strtotal =
String.valueOf(this.total);
if (!"-1".equals(strtotal))
map.put("Total", strtotal);
String strfree =
String.valueOf(this.free);
if (!"-1".equals(strfree))
map.put("Free", strfree);
String strused =
String.valueOf(this.used);
if (!"-1".equals(strused))
map.put("Used", strused);
String stravail =
String.valueOf(this.avail);
if (!"-1".equals(stravail))
map.put("Avail", stravail);
String strfiles =
String.valueOf(this.files);
if (!"-1".equals(strfiles))
map.put("Files", strfiles);
String strfreeFiles =
String.valueOf(this.freeFiles);
if (!"-1".equals(strfreeFiles))
map.put("FreeFiles", strfreeFiles);
String strdiskReads =
String.valueOf(this.diskReads);
if (!"-1".equals(strdiskReads))
map.put("DiskReads", strdiskReads);
String strdiskWrites =
String.valueOf(this.diskWrites);
if (!"-1".equals(strdiskWrites))
map.put("DiskWrites", strdiskWrites);
String strdiskReadBytes =
String.valueOf(this.diskReadBytes);
if (!"-1".equals(strdiskReadBytes))
map.put("DiskReadBytes", strdiskReadBytes);
String strdiskWriteBytes =
String.valueOf(this.diskWriteBytes);
if (!"-1".equals(strdiskWriteBytes))
map.put("DiskWriteBytes", strdiskWriteBytes);
String strdiskQueue =
String.valueOf(this.diskQueue);
if (!"-1".equals(strdiskQueue))
map.put("DiskQueue", strdiskQueue);
String strdiskServiceTime =
String.valueOf(this.diskServiceTime);
if (!"-1".equals(strdiskServiceTime))
map.put("DiskServiceTime", strdiskServiceTime);
String strusePercent =
String.valueOf(this.usePercent);
if (!"-1".equals(strusePercent))
map.put("UsePercent", strusePercent);
return map;
}
public String toString() {
return toMap().toString();
}
}