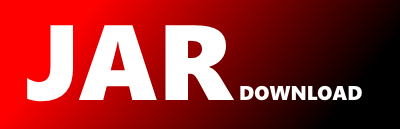
org.hyperic.sigar.NetRoute Maven / Gradle / Ivy
/*****************************************************
* WARNING: this file was generated by -e
* on Mon May 24 16:26:06 2010.
* Any changes made here will be LOST.
*****************************************************/
package org.hyperic.sigar;
import java.util.HashMap;
import java.util.Map;
/**
* NetRoute sigar class.
*/
public class NetRoute implements java.io.Serializable {
private static final long serialVersionUID = 13039L;
public NetRoute() { }
public native void gather(Sigar sigar) throws SigarException;
/**
* This method is not intended to be called directly.
* use Sigar.getNetRoute() instead.
* @exception SigarException on failure.
* @see org.hyperic.sigar.Sigar#getNetRoute
*/
static NetRoute fetch(Sigar sigar) throws SigarException {
NetRoute netRoute = new NetRoute();
netRoute.gather(sigar);
return netRoute;
}
String destination = null;
/**
* Get the destination.
* Supported Platforms: HPUX, Linux, Win32.
*
* System equivalent commands:
* - AIX:
* - Darwin:
* - FreeBSD:
* - HPUX:
* - Linux:
route -n
* - Solaris:
* - Win32:
*
* @return destination
*/
public String getDestination() { return destination; }
String gateway = null;
/**
* Get the gateway.
* Supported Platforms: HPUX, Linux, Win32.
*
* System equivalent commands:
* - AIX:
* - Darwin:
* - FreeBSD:
* - HPUX:
* - Linux:
route -n
* - Solaris:
* - Win32:
*
* @return gateway
*/
public String getGateway() { return gateway; }
long flags = 0;
/**
* Get the flags.
* Supported Platforms: Linux.
*
* System equivalent commands:
* - AIX:
* - Darwin:
* - FreeBSD:
* - HPUX:
* - Linux:
route -n
* - Solaris:
* - Win32:
*
* @return flags
*/
public long getFlags() { return flags; }
long refcnt = 0;
/**
* Get the refcnt.
* Supported Platforms: Linux.
*
* System equivalent commands:
* - AIX:
* - Darwin:
* - FreeBSD:
* - HPUX:
* - Linux:
route -n
* - Solaris:
* - Win32:
*
* @return refcnt
*/
public long getRefcnt() { return refcnt; }
long use = 0;
/**
* Get the use.
* Supported Platforms: Linux.
*
* System equivalent commands:
* - AIX:
* - Darwin:
* - FreeBSD:
* - HPUX:
* - Linux:
route -n
* - Solaris:
* - Win32:
*
* @return use
*/
public long getUse() { return use; }
long metric = 0;
/**
* Get the metric.
* Supported Platforms: Linux.
*
* System equivalent commands:
* - AIX:
* - Darwin:
* - FreeBSD:
* - HPUX:
* - Linux:
route -n
* - Solaris:
* - Win32:
*
* @return metric
*/
public long getMetric() { return metric; }
String mask = null;
/**
* Get the mask.
* Supported Platforms: HPUX, Linux.
*
* System equivalent commands:
* - AIX:
* - Darwin:
* - FreeBSD:
* - HPUX:
* - Linux:
route -n
* - Solaris:
* - Win32:
*
* @return mask
*/
public String getMask() { return mask; }
long mtu = 0;
/**
* Get the mtu.
* Supported Platforms: Linux.
*
* System equivalent commands:
* - AIX:
* - Darwin:
* - FreeBSD:
* - HPUX:
* - Linux:
route -n
* - Solaris:
* - Win32:
*
* @return mtu
*/
public long getMtu() { return mtu; }
long window = 0;
/**
* Get the window.
* Supported Platforms: Linux.
*
* System equivalent commands:
* - AIX:
* - Darwin:
* - FreeBSD:
* - HPUX:
* - Linux:
route -n
* - Solaris:
* - Win32:
*
* @return window
*/
public long getWindow() { return window; }
long irtt = 0;
/**
* Get the irtt.
* Supported Platforms: Linux.
*
* System equivalent commands:
* - AIX:
* - Darwin:
* - FreeBSD:
* - HPUX:
* - Linux:
route -n
* - Solaris:
* - Win32:
*
* @return irtt
*/
public long getIrtt() { return irtt; }
String ifname = null;
/**
* Get the ifname.
* Supported Platforms: Linux.
*
* System equivalent commands:
* - AIX:
* - Darwin:
* - FreeBSD:
* - HPUX:
* - Linux:
route -n
* - Solaris:
* - Win32:
*
* @return ifname
*/
public String getIfname() { return ifname; }
void copyTo(NetRoute copy) {
copy.destination = this.destination;
copy.gateway = this.gateway;
copy.flags = this.flags;
copy.refcnt = this.refcnt;
copy.use = this.use;
copy.metric = this.metric;
copy.mask = this.mask;
copy.mtu = this.mtu;
copy.window = this.window;
copy.irtt = this.irtt;
copy.ifname = this.ifname;
}
public Map toMap() {
Map map = new HashMap();
String strdestination =
String.valueOf(this.destination);
if (!"-1".equals(strdestination))
map.put("Destination", strdestination);
String strgateway =
String.valueOf(this.gateway);
if (!"-1".equals(strgateway))
map.put("Gateway", strgateway);
String strflags =
String.valueOf(this.flags);
if (!"-1".equals(strflags))
map.put("Flags", strflags);
String strrefcnt =
String.valueOf(this.refcnt);
if (!"-1".equals(strrefcnt))
map.put("Refcnt", strrefcnt);
String struse =
String.valueOf(this.use);
if (!"-1".equals(struse))
map.put("Use", struse);
String strmetric =
String.valueOf(this.metric);
if (!"-1".equals(strmetric))
map.put("Metric", strmetric);
String strmask =
String.valueOf(this.mask);
if (!"-1".equals(strmask))
map.put("Mask", strmask);
String strmtu =
String.valueOf(this.mtu);
if (!"-1".equals(strmtu))
map.put("Mtu", strmtu);
String strwindow =
String.valueOf(this.window);
if (!"-1".equals(strwindow))
map.put("Window", strwindow);
String strirtt =
String.valueOf(this.irtt);
if (!"-1".equals(strirtt))
map.put("Irtt", strirtt);
String strifname =
String.valueOf(this.ifname);
if (!"-1".equals(strifname))
map.put("Ifname", strifname);
return map;
}
public String toString() {
return toMap().toString();
}
}