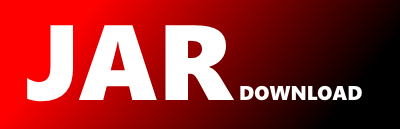
org.hyperic.sigar.ProcCred Maven / Gradle / Ivy
/*****************************************************
* WARNING: this file was generated by -e
* on Mon May 24 16:26:06 2010.
* Any changes made here will be LOST.
*****************************************************/
package org.hyperic.sigar;
import java.util.HashMap;
import java.util.Map;
/**
* ProcCred sigar class.
*/
public class ProcCred implements java.io.Serializable {
private static final long serialVersionUID = 3062L;
public ProcCred() { }
public native void gather(Sigar sigar, long pid) throws SigarException;
/**
* This method is not intended to be called directly.
* use Sigar.getProcCred() instead.
* @exception SigarException on failure.
* @see org.hyperic.sigar.Sigar#getProcCred
*/
static ProcCred fetch(Sigar sigar, long pid) throws SigarException {
ProcCred procCred = new ProcCred();
procCred.gather(sigar, pid);
return procCred;
}
long uid = 0;
/**
* Get the Process user id.
* Supported Platforms: AIX, Darwin, FreeBSD, HPUX, Linux, Solaris.
*
* System equivalent commands:
* - AIX:
top, ps
* - Darwin:
top, ps
* - FreeBSD:
top, ps
* - HPUX:
top, ps
* - Linux:
top, ps
* - Solaris:
top, ps
* - Win32:
taskman
*
* @return Process user id
*/
public long getUid() { return uid; }
long gid = 0;
/**
* Get the Process group id.
* Supported Platforms: AIX, Darwin, FreeBSD, HPUX, Linux, Solaris.
*
* System equivalent commands:
* - AIX:
top, ps
* - Darwin:
top, ps
* - FreeBSD:
top, ps
* - HPUX:
top, ps
* - Linux:
top, ps
* - Solaris:
top, ps
* - Win32:
taskman
*
* @return Process group id
*/
public long getGid() { return gid; }
long euid = 0;
/**
* Get the Process effective user id.
* Supported Platforms: AIX, Darwin, FreeBSD, HPUX, Linux, Solaris.
*
* System equivalent commands:
* - AIX:
top, ps
* - Darwin:
top, ps
* - FreeBSD:
top, ps
* - HPUX:
top, ps
* - Linux:
top, ps
* - Solaris:
top, ps
* - Win32:
taskman
*
* @return Process effective user id
*/
public long getEuid() { return euid; }
long egid = 0;
/**
* Get the Process effective group id.
* Supported Platforms: AIX, Darwin, FreeBSD, HPUX, Linux, Solaris.
*
* System equivalent commands:
* - AIX:
top, ps
* - Darwin:
top, ps
* - FreeBSD:
top, ps
* - HPUX:
top, ps
* - Linux:
top, ps
* - Solaris:
top, ps
* - Win32:
taskman
*
* @return Process effective group id
*/
public long getEgid() { return egid; }
void copyTo(ProcCred copy) {
copy.uid = this.uid;
copy.gid = this.gid;
copy.euid = this.euid;
copy.egid = this.egid;
}
public Map toMap() {
Map map = new HashMap();
String struid =
String.valueOf(this.uid);
if (!"-1".equals(struid))
map.put("Uid", struid);
String strgid =
String.valueOf(this.gid);
if (!"-1".equals(strgid))
map.put("Gid", strgid);
String streuid =
String.valueOf(this.euid);
if (!"-1".equals(streuid))
map.put("Euid", streuid);
String stregid =
String.valueOf(this.egid);
if (!"-1".equals(stregid))
map.put("Egid", stregid);
return map;
}
public String toString() {
return toMap().toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy